
Several examples run on only mbed-os5.13.0 (not 5.14.0)
Dependencies: BD_SD_DISCO_F769NI BSP_DISCO_F769NI LCD_DISCO_F769NI TS_DISCO_F769NI USBHost_F769NI
button_group.hpp
00001 //----------------------------------------------------------- 00002 // Button group class -- Header 00003 // 00004 // 2016/02/22, Copyright (c) 2016 MIKAMI, Naoki 00005 //----------------------------------------------------------- 00006 // Modified by JH1PJL/K.Arai Apr.26,2018 for DISCO-F469NI 00007 // Modified by JH1PJL/K.Arai Jul.25,2019 for DISCO-F769NI 00008 00009 #ifndef F769_BUTTON_GROUP_HPP 00010 #define F769_BUTTON_GROUP_HPP 00011 00012 #include "button.hpp" 00013 #include <string> 00014 00015 namespace Mikami 00016 { 00017 class ButtonGroup 00018 { 00019 public: 00020 // Constructor 00021 ButtonGroup(LCD_DISCO_F769NI &lcd, TS_DISCO_F769NI &ts, 00022 uint16_t x0, uint16_t y0, 00023 uint16_t width, uint16_t height, 00024 uint32_t color, uint32_t backColor, 00025 uint16_t number, const string str[], 00026 uint16_t spaceX = 0, uint16_t spaceY = 0, 00027 uint16_t column = 1, 00028 sFONT &fonts = Font12, 00029 uint32_t textColor = LCD_COLOR_WHITE); 00030 00031 // Destructor 00032 ~ButtonGroup(); 00033 00034 // Draw button 00035 bool Draw(int num, uint32_t color, 00036 uint32_t textColor = LCD_COLOR_WHITE); 00037 00038 // Draw all buttons 00039 void DrawAll(uint32_t color, 00040 uint32_t textColor = LCD_COLOR_WHITE) 00041 { 00042 for (int n=0; n<numberOfButtons_; n++) 00043 buttons_[n]->Draw(color, textColor); 00044 } 00045 00046 // Redraw button with original color 00047 bool Redraw(int num, uint32_t textColor = LCD_COLOR_WHITE); 00048 00049 // Erase button with selected color 00050 bool Erase(int num, uint32_t color); 00051 00052 // Check touch detected for specified button 00053 bool Touched(int num); 00054 00055 // Check touch detected for specified button and redraw 00056 bool Touched(int num, uint32_t color, 00057 uint32_t textColor = LCD_COLOR_WHITE); 00058 00059 // Get touched number 00060 bool GetTouchedNumber(int &num); 00061 00062 // Get touched number and redraw button if touched 00063 bool GetTouchedNumber(int &num, uint32_t color); 00064 00065 private: 00066 Button **buttons_; 00067 int numberOfButtons_; 00068 int touchedNum_; 00069 00070 // Check range of argument 00071 bool Range(int n) 00072 { return ((n >= 0) && (n < numberOfButtons_)); } 00073 00074 // disallow copy constructor and assignment operator 00075 ButtonGroup(const ButtonGroup&); 00076 ButtonGroup& operator=(const ButtonGroup&); 00077 }; 00078 } 00079 #endif // F769_BUTTON_GROUP_HPP
Generated on Sat Jul 16 2022 06:38:26 by
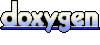