
Several examples run on only mbed-os5.13.0 (not 5.14.0)
Dependencies: BD_SD_DISCO_F769NI BSP_DISCO_F769NI LCD_DISCO_F769NI TS_DISCO_F769NI USBHost_F769NI
button_group.cpp
00001 //----------------------------------------------------------- 00002 // Button group class 00003 // 00004 // 2016/02/22, Copyright (c) 2016 MIKAMI, Naoki 00005 //----------------------------------------------------------- 00006 // Modified by JH1PJL/K.Arai Apr.26,2018 for DISCO-F469NI 00007 // Modified by JH1PJL/K.Arai Jul.25,2019 for DISCO-F769NI 00008 00009 #include "button_group.hpp" 00010 00011 namespace Mikami 00012 { 00013 // Constructor 00014 ButtonGroup::ButtonGroup(LCD_DISCO_F769NI &lcd, TS_DISCO_F769NI &ts, 00015 uint16_t x0, uint16_t y0, 00016 uint16_t width, uint16_t height, 00017 uint32_t color, uint32_t backColor, 00018 uint16_t number, const string str[], 00019 uint16_t spaceX, uint16_t spaceY, 00020 uint16_t column, 00021 sFONT &fonts, uint32_t textColor) 00022 : numberOfButtons_(number), touchedNum_(-1) 00023 { 00024 buttons_ = new Button *[number]; 00025 for (int n=0; n<number; n++) 00026 { 00027 div_t u1 = div(n, column); 00028 uint16_t x = x0 + u1.rem*(width + spaceX); 00029 uint16_t y = y0 + u1.quot*(height + spaceY); 00030 buttons_[n] = new Button(lcd, ts, x, y, width, height, 00031 color, backColor, 00032 str[n], fonts, textColor); 00033 } 00034 } 00035 00036 // Destructor 00037 ButtonGroup::~ButtonGroup() 00038 { 00039 for (int n=0; n<numberOfButtons_; n++) delete buttons_[n]; 00040 delete[] *buttons_; 00041 } 00042 00043 // Draw button 00044 bool ButtonGroup::Draw(int num, uint32_t color, uint32_t textColor) 00045 { 00046 if (!Range(num)) return false; 00047 buttons_[num]->Draw(color, textColor); 00048 touchedNum_ = num; 00049 return true; 00050 } 00051 00052 // Redraw button with original color 00053 bool ButtonGroup::Redraw(int num, uint32_t textColor) 00054 { 00055 if (!Range(num)) return false; 00056 buttons_[num]->Redraw(textColor); 00057 return true; 00058 } 00059 00060 // Erase button with selected color 00061 bool ButtonGroup::Erase(int num, uint32_t color) 00062 { 00063 if (!Range(num)) return false; 00064 buttons_[num]->Draw(color, color); 00065 return true; 00066 } 00067 00068 // Check touch detected for specified button 00069 bool ButtonGroup::Touched(int num) 00070 { 00071 if (!Range(num)) return false; 00072 bool touched = buttons_[num]->Touched(); 00073 if (touched) touchedNum_ = num; 00074 return touched; 00075 } 00076 00077 // Check touch detected for specified button and redraw 00078 bool ButtonGroup::Touched(int num, uint32_t color, 00079 uint32_t textColor) 00080 { 00081 if (!Range(num)) return false; 00082 bool touched = buttons_[num]->Touched(color, textColor); 00083 if (touched) 00084 { 00085 if (Range(touchedNum_) && (num != touchedNum_)) 00086 buttons_[touchedNum_]->Redraw(); 00087 touchedNum_ = num; 00088 } 00089 return touched; 00090 } 00091 00092 // Get touched number 00093 bool ButtonGroup::GetTouchedNumber(int &num) 00094 { 00095 if (buttons_[0]->PanelTouched()) 00096 { 00097 for (int n=0; n<numberOfButtons_; n++) 00098 if (buttons_[n]->IsOnButton()) 00099 { 00100 num = n; 00101 return true; 00102 } 00103 return false; 00104 } 00105 else 00106 return false; 00107 } 00108 00109 // Get touched number and redraw button if touched 00110 bool ButtonGroup::GetTouchedNumber(int &num, uint32_t color) 00111 { 00112 if (GetTouchedNumber(num)) 00113 { 00114 buttons_[num]->Draw(color); 00115 if (Range(touchedNum_) && (num != touchedNum_)) 00116 buttons_[touchedNum_]->Redraw(); 00117 touchedNum_ = num; 00118 return true; 00119 } 00120 else 00121 return false; 00122 } 00123 }
Generated on Sat Jul 16 2022 06:38:26 by
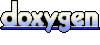