
Several examples run on only mbed-os5.13.0 (not 5.14.0)
Dependencies: BD_SD_DISCO_F769NI BSP_DISCO_F769NI LCD_DISCO_F769NI TS_DISCO_F769NI USBHost_F769NI
button.hpp
00001 //----------------------------------------------------------- 00002 // Button class handling multi-touch -- Header 00003 // Multi-touch: Enabled (default) 00004 // 00005 // 2016/02/22, Copyright (c) 2016 MIKAMI, Naoki 00006 //----------------------------------------------------------- 00007 // Modified by JH1PJL/K.Arai Nov.27,2017 for DISCO-F469NI 00008 // Modified by JH1PJL/K.Arai Jul.25,2019 for DISCO-F769NI 00009 00010 #ifndef F769_BUTTON_HPP 00011 #define F769_BUTTON_HPP 00012 00013 #include "mbed.h" 00014 #include <string> 00015 #include "TS_DISCO_F769NI.h" 00016 #include "LCD_DISCO_F769NI.h" 00017 00018 namespace Mikami 00019 { 00020 class Button 00021 { 00022 public: 00023 // Constructor 00024 Button(LCD_DISCO_F769NI &lcd, TS_DISCO_F769NI &ts, 00025 uint16_t x, uint16_t y, uint16_t width, uint16_t height, 00026 uint32_t color, uint32_t backColor, 00027 const string str = "", sFONT &fonts = Font12, 00028 uint32_t textColor = LCD_COLOR_WHITE) 00029 : lcd_(lcd), ts_(ts), X_(x), Y_(y), W_(width), H_(height), 00030 ORIGINAL_COLOR_(color), BACK_COLOR_(backColor), 00031 STR_(str), FONTS_(&fonts), FONT_WIDTH_(fonts.Width), 00032 FONT_HEIGHT_(fonts.Height) 00033 { Draw(color, textColor); } 00034 00035 // Draw button 00036 void Draw(uint32_t color, uint32_t textColor = LCD_COLOR_WHITE); 00037 00038 // Redraw button with original color 00039 void Redraw(uint32_t textColor = LCD_COLOR_WHITE) 00040 { Draw(ORIGINAL_COLOR_, textColor); } 00041 00042 // Erase button 00043 void Erase() 00044 { Draw(BACK_COLOR_, BACK_COLOR_); } 00045 00046 // Check touch detected 00047 bool Touched(); 00048 00049 // Check touch detected and redraw button 00050 bool Touched(uint32_t color, uint32_t textColor = LCD_COLOR_WHITE); 00051 00052 // Get original color 00053 uint32_t GetOriginalColor() { return ORIGINAL_COLOR_; } 00054 00055 bool PanelTouched(); 00056 bool IsOnButton(); 00057 00058 // Get previously got state 00059 static TS_StateTypeDef GottenState() 00060 { return state_; } 00061 00062 // Set or reset multi-touch 00063 static void SetMultiTouch(bool tf) { multiTouch = tf; } 00064 00065 private: 00066 LCD_DISCO_F769NI &lcd_; 00067 TS_DISCO_F769NI &ts_; 00068 00069 const uint16_t X_, Y_, W_, H_; 00070 const uint32_t ORIGINAL_COLOR_; // original color 00071 const uint32_t BACK_COLOR_; // back color of screen 00072 const string STR_; 00073 sFONT *const FONTS_; 00074 const uint16_t FONT_WIDTH_; 00075 const uint16_t FONT_HEIGHT_; 00076 00077 static TS_StateTypeDef state_; 00078 static bool multiTouch; 00079 00080 // disallow copy constructor and assignment operator 00081 Button(const Button&); 00082 Button& operator=(const Button&); 00083 }; 00084 } 00085 #endif // F769_BUTTON_HPP
Generated on Sat Jul 16 2022 06:38:26 by
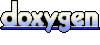