
Several examples run on only mbed-os5.13.0 (not 5.14.0)
Dependencies: BD_SD_DISCO_F769NI BSP_DISCO_F769NI LCD_DISCO_F769NI TS_DISCO_F769NI USBHost_F769NI
5_tcp_server.cpp
00001 // Original 00002 // https://os.mbed.com/teams/ST/code/mbed-os-tcp-server-example/ 00003 // 00004 // Modified by K.Arai 00005 // October 14th, 2019 00006 // 00007 00008 #include "select_program.h" 00009 //#define EXAMPLE_5_TCP_SERVER 00010 #ifdef EXAMPLE_5_TCP_SERVER 00011 00012 #if !FEATURE_LWIP 00013 #error [NOT_SUPPORTED] LWIP not supported for this target 00014 #endif 00015 00016 #include "mbed.h" 00017 #include "EthernetInterface.h" 00018 #include "TCPServer.h" 00019 #include "TCPSocket.h" 00020 00021 Serial pc(USBTX, USBRX, 115200); 00022 00023 #define HTTP_STATUS_LINE "HTTP/1.0 200 OK" 00024 #define HTTP_HEADER_FIELDS "Content-Type: text/html; charset=utf-8" 00025 #define HTTP_MESSAGE_BODY "" \ 00026 "<html>" "\r\n" \ 00027 " <body style=\"display:flex;text-align:center\">" "\r\n" \ 00028 " <div style=\"margin:auto\">" "\r\n" \ 00029 " <h1>Hello World</h1>" "\r\n" \ 00030 " <p>It works !</p>" "\r\n" \ 00031 " </div>" "\r\n" \ 00032 " </body>" "\r\n" \ 00033 "</html>" 00034 00035 #define HTTP_RESPONSE HTTP_STATUS_LINE "\r\n" \ 00036 HTTP_HEADER_FIELDS "\r\n" \ 00037 "\r\n" \ 00038 HTTP_MESSAGE_BODY "\r\n" 00039 00040 int main() 00041 { 00042 pc.printf("\x1b[2J\x1b[H %s\r\n %s %s (UTC)\r\n", 00043 __FILE__, __DATE__, __TIME__); 00044 printf(" Basic HTTP server example for DISCO-F769NI:\r\n"); 00045 00046 EthernetInterface eth; 00047 eth.connect(); 00048 00049 printf("The target IP address is '%s'\r\n", eth.get_ip_address()); 00050 00051 TCPServer srv; 00052 TCPSocket clt_sock; 00053 SocketAddress clt_addr; 00054 00055 /* Open the server on ethernet stack */ 00056 srv.open(ð); 00057 00058 /* Bind the HTTP port (TCP 80) to the server */ 00059 srv.bind(eth.get_ip_address(), 80); 00060 00061 /* Can handle 5 simultaneous connections */ 00062 srv.listen(5); 00063 00064 while (true) { 00065 srv.accept(&clt_sock, &clt_addr); 00066 printf("accept %s:%d\r\n", clt_addr.get_ip_address(), clt_addr.get_port()); 00067 clt_sock.send(HTTP_RESPONSE, strlen(HTTP_RESPONSE)); 00068 } 00069 } 00070 00071 #endif
Generated on Sat Jul 16 2022 06:38:26 by
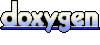