
Several examples run on only mbed-os5.13.0 (not 5.14.0)
Dependencies: BD_SD_DISCO_F769NI BSP_DISCO_F769NI LCD_DISCO_F769NI TS_DISCO_F769NI USBHost_F769NI
0_led_blinky.cpp
00001 /* 00002 * Mbed Application program / Blinky 00003 * 00004 * Copyright (c) 2018,'19 Kenji Arai / JH1PJL 00005 * http://www.page.sannet.ne.jp/kenjia/index.html 00006 * https://os.mbed.com/users/kenjiArai/ 00007 * Created: April 10th, 2018 00008 * Revised: October 14th, 2019 00009 */ 00010 00011 #include "select_program.h" 00012 //#define EXAMPLE_0_BLINKY_LED 00013 #ifdef EXAMPLE_0_BLINKY_LED 00014 00015 // Include -------------------------------------------------------------------- 00016 #include "mbed.h" 00017 00018 // Definition ----------------------------------------------------------------- 00019 #define LEDON 1 00020 #define LEDOFF 0 00021 00022 #define FOREVER 0x7fffffff 00023 00024 // Constructor ---------------------------------------------------------------- 00025 DigitalOut my_led1(LED1); 00026 DigitalOut my_led2(LED2); 00027 DigitalOut my_led3(LED3); 00028 Serial pc(USBTX, USBRX, 115200); 00029 00030 // RAM ------------------------------------------------------------------------ 00031 00032 // ROM / Constant data -------------------------------------------------------- 00033 00034 // Function prototypes -------------------------------------------------------- 00035 static void tsk_0(void const *args); 00036 static void tsk_1(void const *args); 00037 static void tsk_2(void const *args); 00038 static void tsk_3(void const *args); 00039 00040 //------------------------------------------------------------------------------ 00041 // Control Program 00042 //------------------------------------------------------------------------------ 00043 osThreadDef(tsk_0, osPriorityNormal,1024); 00044 osThreadDef(tsk_1, osPriorityNormal,1024); 00045 osThreadDef(tsk_2, osPriorityNormal,1024); 00046 osThreadDef(tsk_3, osPriorityNormal,1024); 00047 00048 int main() 00049 { 00050 pc.printf("\x1b[2J\x1b[H %s\r\n %s %s (UTC)\r\n", 00051 __FILE__, __DATE__, __TIME__); 00052 printf(" LED BLINKY EXAMPLE FOR DISCO-F769NI:\r\n"); 00053 osThreadId id0, id1, id2, id3; 00054 id0 = osThreadCreate(osThread(tsk_0), NULL); 00055 id1 = osThreadCreate(osThread(tsk_1), NULL); 00056 id2 = osThreadCreate(osThread(tsk_2), NULL); 00057 id3 = osThreadCreate(osThread(tsk_3), NULL); 00058 pc.printf("id0=0x%x, id1=0x%x, id2=0x%x, id3=0x%x\r\n", 00059 (uint32_t)id0, (uint32_t)id1, (uint32_t)id2, (uint32_t)id3); 00060 while(true) { 00061 ThisThread::sleep_for(FOREVER); 00062 } 00063 } 00064 00065 void tsk_0(void const *args) 00066 { 00067 Timer t; 00068 uint32_t count = 0; 00069 00070 while(true) { 00071 t.reset(); 00072 t.start(); 00073 pc.printf("%5d\r\n", count++); 00074 uint32_t wait = 1000 - t.read_ms(); 00075 ThisThread::sleep_for(wait); 00076 } 00077 } 00078 00079 void tsk_1(void const *args) 00080 { 00081 while(true) { 00082 my_led1 = LEDON; 00083 ThisThread::sleep_for(5); 00084 my_led1 = LEDOFF; 00085 ThisThread::sleep_for(1995); 00086 } 00087 } 00088 00089 void tsk_2(void const *args) 00090 { 00091 while(true) { 00092 my_led2 = LEDON; 00093 ThisThread::sleep_for(5); 00094 my_led2 = LEDOFF; 00095 ThisThread::sleep_for(2995); 00096 } 00097 } 00098 00099 void tsk_3(void const *args) 00100 { 00101 while(true) { 00102 my_led3 = LEDON; 00103 ThisThread::sleep_for(5); 00104 my_led3 = LEDOFF; 00105 ThisThread::sleep_for(3995); 00106 } 00107 } 00108 00109 #endif
Generated on Sat Jul 16 2022 06:38:26 by
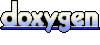