
Arduino board run on Mbed-os6.8.1 (only test purpose not official). Need Mbed Studio(1.3.1) not online compiler. If you compile on the online compiler, you can get a hex file but it does NOT work!!!
Dependencies: APDS_9960 LPS22HB LSM9DS1 HTS221
main6.cpp
00001 /* 00002 * APDS-9960 00003 * Digital Proximity, Ambient Light, RGB and Gesture Sensor 00004 * 00005 * Mbed Application program 00006 * Nano 33 BLE Sense board runs on mbed-OS6 00007 * Modified by Kenji Arai / JH1PJL 00008 * http://www7b.biglobe.ne.jp/~kenjia/ 00009 * https://os.mbed.com/users/kenjiArai/ 00010 * Started: February 2nd, 2020 00011 * Revised: February 28th, 2021 00012 * 00013 * Original: 00014 * https://os.mbed.com/users/kbhagat6/code/Gesture_User_Interface/ 00015 */ 00016 00017 // Pre-selection -------------------------------------------------------------- 00018 #include "select_example.h" 00019 //#define EXAMPLE_6_CHECK_APDS_9960 00020 #ifdef EXAMPLE_6_CHECK_APDS_9960 00021 00022 // Include -------------------------------------------------------------------- 00023 #include "mbed.h" 00024 #include "nano33blesense_iodef.h" 00025 #include "glibr.h" 00026 00027 // Definition ----------------------------------------------------------------- 00028 00029 // Object --------------------------------------------------------------------- 00030 InterruptIn apds_int(PIN_APDS_INT); 00031 DigitalOut sen_pwr(PIN_VDD_ENV, 1); 00032 DigitalOut i2c_pullup(PIN_I2C_PULLUP, 1); 00033 DigitalOut led_y(PIN_YELLOW, 0); 00034 DigitalOut led_g(PIN_GREEN, 0); 00035 DigitalOut lr(PIN_LR, 1); 00036 DigitalOut lg(PIN_LG, 1); 00037 DigitalOut lb(PIN_LB, 1); 00038 I2C i2c(PIN_SDA1, PIN_SCL1); 00039 glibr *gesture = NULL; 00040 00041 // ROM / Constant data -------------------------------------------------------- 00042 00043 // RAM ------------------------------------------------------------------------ 00044 00045 // Function prototypes -------------------------------------------------------- 00046 void apds_hit_callback (void); 00047 00048 // subroutin (must be at this below line and do NOT change order) ------------- 00049 #include "usb_serial_as_stdio.h" 00050 #include "check_revision.h" 00051 #include "common.h" 00052 00053 //------------------------------------------------------------------------------ 00054 // Control Program 00055 //------------------------------------------------------------------------------ 00056 int main() 00057 { 00058 bool gerror = false; 00059 00060 usb_serial_initialize(); 00061 print_revision(); 00062 i2c_pullup = 1; 00063 sen_pwr = 1; 00064 print_usb("Check APDS_9960\r\n"); 00065 ThisThread::sleep_for(200ms); 00066 // check I2C line 00067 check_i2c_connected_devices(); 00068 gesture = new glibr(PIN_SDA1, PIN_SCL1); 00069 if (gesture->ginit()) { 00070 print_usb("APDS-9960 initialization complete\n\r"); 00071 } else { 00072 print_usb("Something went wrong during APDS-9960 init\n\r"); 00073 gerror=true; 00074 } 00075 // Start running the APDS-9960 gesture sensor engine 00076 if ( gesture->enableGestureSensor(true) ) { 00077 print_usb("Gesture sensor is now running\n\r"); 00078 } else { 00079 print_usb("Something went wrong during gesture sensor init!\n\r"); 00080 gerror=true; 00081 } 00082 // set pullup for Interrupt input pin 00083 apds_int.mode(PullUp); 00084 // Delay for initial pullup to take effect 00085 ThisThread::sleep_for(1ms); 00086 // Setup Interrupt callback function for a pb hit 00087 apds_int.fall(&apds_hit_callback); 00088 // Enable interrut 00089 apds_int.enable_irq(); 00090 while(!(gesture->isGestureAvailable())){ 00091 ThisThread::sleep_for(200ms); 00092 } 00093 int temp; 00094 while(gerror == false) { 00095 if (gesture->isGestureAvailable()) { 00096 if(gesture->isGestureAvailable()){ 00097 temp = gesture->readGesture(); 00098 } 00099 switch ( temp) { 00100 case DIR_UP: 00101 print_usb("Forward\r\n"); 00102 break; 00103 case DIR_DOWN: 00104 print_usb("Backward\r\n"); 00105 break; 00106 case DIR_LEFT: 00107 print_usb("LEFT\r\n"); 00108 break; 00109 case DIR_RIGHT: 00110 print_usb("RIGHT\r\n"); 00111 break; 00112 case 67: 00113 print_usb("Collision\r\n"); 00114 break; 00115 case DIR_NEAR: 00116 print_usb("NEAR\r\n"); 00117 break; 00118 case DIR_FAR: 00119 print_usb("FAR\r\n"); 00120 break; 00121 default: 00122 print_usb("NONE\r\n"); 00123 break; 00124 } 00125 } 00126 } 00127 } 00128 00129 void apds_hit_callback (void) 00130 { 00131 led_y =!led_y; 00132 } 00133 00134 #endif // EXAMPLE_6_CHECK_APDS_9960
Generated on Thu Jul 14 2022 06:04:06 by
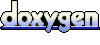