
Arduino board run on Mbed-os6.8.1 (only test purpose not official). Need Mbed Studio(1.3.1) not online compiler. If you compile on the online compiler, you can get a hex file but it does NOT work!!!
Dependencies: APDS_9960 LPS22HB LSM9DS1 HTS221
main5.cpp
00001 /* 00002 * Mbed Application program 00003 * Nano 33 BLE Sense board runs on mbed-OS6 00004 * HTS221 + LSM9DS1 + LPS22HB sensors 00005 * 00006 * Copyright (c) 2020,'21 Kenji Arai / JH1PJL 00007 * http://www7b.biglobe.ne.jp/~kenjia/ 00008 * https://os.mbed.com/users/kenjiArai/ 00009 * Started: January 22nd, 2020 00010 * Revised: February 28th, 2021 00011 */ 00012 00013 // Pre-selection -------------------------------------------------------------- 00014 #include "select_example.h" 00015 //#define EXAMPLE_5_COMB_ENV_SENSORS 00016 #ifdef EXAMPLE_5_COMB_ENV_SENSORS 00017 00018 // Include -------------------------------------------------------------------- 00019 #include "mbed.h" 00020 #include "nano33blesense_iodef.h" 00021 #include "LPS22HB.h" 00022 #include "LSM9DS1.h" 00023 #include "HTS221.h" 00024 00025 // Definition ----------------------------------------------------------------- 00026 00027 // Object --------------------------------------------------------------------- 00028 DigitalOut sen_pwr(PIN_VDD_ENV, 1); 00029 DigitalOut i2c_pullup(PIN_I2C_PULLUP, 1); 00030 DigitalOut led_y(PIN_YELLOW, 0); 00031 DigitalOut led_g(PIN_GREEN, 0); 00032 DigitalOut lr(PIN_LR, 1); 00033 DigitalOut lg(PIN_LG, 1); 00034 DigitalOut lb(PIN_LB, 1); 00035 I2C i2c(PIN_SDA1, PIN_SCL1); 00036 LSM9DS1 *imu = NULL; 00037 LPS22HB *baro = NULL; 00038 HTS221 *hum = NULL; 00039 Timer t; 00040 00041 // RAM ------------------------------------------------------------------------ 00042 00043 // ROM / Constant data -------------------------------------------------------- 00044 const char *const msg0 = "Run Nano 33 BLE Sense module on Mbed-os"; 00045 const char *const msg1 = "Compiled on Mbed Studio:1.3.1"; 00046 const char *const msg2 = "Target is ARDUINO_NANO33BLE with Debug mode"; 00047 00048 // Function prototypes -------------------------------------------------------- 00049 00050 // subroutin (must be at this below line and do NOT change order) ------------- 00051 #include "usb_serial_as_stdio.h" 00052 #include "check_revision.h" 00053 #include "common.h" 00054 00055 //------------------------------------------------------------------------------ 00056 // Control Program 00057 //------------------------------------------------------------------------------ 00058 int main() 00059 { 00060 HTS221::HTS221_status_t aux; 00061 HTS221::HTS221_data_t hum_data; 00062 00063 usb_serial_initialize(); 00064 print_revision(); 00065 led_y = 1; 00066 i2c_pullup = 1; 00067 sen_pwr = 1; 00068 print_usb("%s\r\n%s\r\n%s\r\n", msg0, msg1, msg2); 00069 ThisThread::sleep_for(200ms); 00070 imu = new LSM9DS1(PIN_SDA1, PIN_SCL1); 00071 baro = new LPS22HB(i2c, LPS22HB_G_CHIP_ADDR); 00072 hum = new HTS221(PIN_SDA1, PIN_SCL1,HTS221::HTS221_ADDRESS, 400000); 00073 baro->set_odr(); 00074 check_i2c_sensors(); 00075 uint32_t n = 0; 00076 led_y = 0; 00077 led_g = 1; 00078 while(true) { 00079 t.reset(); 00080 t.start(); 00081 // Starting 00082 lr = 0; 00083 baro->get(); 00084 hum->HTS221_SetOneShot(); 00085 imu->begin(); 00086 lr = 1; 00087 // Waiting 00088 lg = 0; 00089 do { 00090 thread_sleep_for(2); 00091 aux = hum->HTS221_GetOneShot(&hum_data); 00092 } while(hum_data.one_shot == HTS221::CTRL_REG2_ONE_SHOT_WAITING); 00093 while (baro->data_ready() == 0){ 00094 thread_sleep_for(2); 00095 } 00096 lg = 1; 00097 // Reading 00098 lb = 0; 00099 hum->HTS221_GetCalibrationCoefficients (&hum_data); 00100 hum->HTS221_GetTemperature(&hum_data); 00101 hum->HTS221_GetHumidity(&hum_data); 00102 imu->readTemp(); 00103 float press = baro->pressure(); 00104 float temp = baro->temperature(); 00105 uint32_t passed_time = chrono::duration_cast<chrono::milliseconds>( 00106 t.elapsed_time()).count(); 00107 lb = 1; 00108 // Showing 00109 lr = 0; 00110 lg = 0; 00111 lb = 0; 00112 print_usb("Temperature[degC]: HTS221=,%+4.1f,", hum_data.temperature); 00113 print_usb("LPS22HB=,%+4.1f,LSM9DS1=,%+4.1f,", 00114 temp, imu->temperature_c); 00115 print_usb("Humidity[RH %%]: HTS221=,%3.1f,", hum_data.humidity); 00116 print_usb("Pressure[hPa]: LPS22H=,%6.2f,", press); 00117 ++n; 00118 print_usb("processing time:,%2d,[ms],count:,%4d\r\n", passed_time, n); 00119 lr = 1; 00120 lg = 1; 00121 lb = 1; 00122 if (passed_time < 999){ 00123 ThisThread::sleep_for(chrono::milliseconds(1000 - passed_time)); 00124 } 00125 } 00126 } 00127 00128 #endif // EXAMPLE_5_COMB_ENV_SENSORS
Generated on Thu Jul 14 2022 06:04:06 by
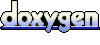