
Arduino board run on Mbed-os6.8.1 (only test purpose not official). Need Mbed Studio(1.3.1) not online compiler. If you compile on the online compiler, you can get a hex file but it does NOT work!!!
Dependencies: APDS_9960 LPS22HB LSM9DS1 HTS221
main4.cpp
00001 /* 00002 * Mbed Application program 00003 * Nano 33 BLE Sense board runs on mbed-OS6 00004 * HTS221 -> Capacitive digital sensor for relative humidity and temperature 00005 * by STMicroelectronics 00006 * 00007 * Copyright (c) 2020,'21 Kenji Arai / JH1PJL 00008 * http://www7b.biglobe.ne.jp/~kenjia/ 00009 * https://os.mbed.com/users/kenjiArai/ 00010 * Started: January 22nd, 2020 00011 * Revised: February 28th, 2021 00012 */ 00013 00014 // Pre-selection -------------------------------------------------------------- 00015 #include "select_example.h" 00016 //#define EXAMPLE_4_CHECK_HTS221 00017 #ifdef EXAMPLE_4_CHECK_HTS221 00018 00019 // Include -------------------------------------------------------------------- 00020 #include "mbed.h" 00021 #include "nano33blesense_iodef.h" 00022 #include "HTS221.h" 00023 00024 // Definition ----------------------------------------------------------------- 00025 00026 // Constructor ---------------------------------------------------------------- 00027 DigitalOut sen_pwr(PIN_VDD_ENV, 1); 00028 DigitalOut i2c_pullup(PIN_I2C_PULLUP, 1); 00029 I2C i2c(PIN_SDA1, PIN_SCL1); 00030 HTS221 *hum = NULL; 00031 Timer t; 00032 00033 // RAM ------------------------------------------------------------------------ 00034 00035 // ROM / Constant data -------------------------------------------------------- 00036 00037 // Function prototypes -------------------------------------------------------- 00038 00039 // subroutin (must be at this below line and do NOT change order) ------------- 00040 #include "usb_serial_as_stdio.h" 00041 #include "check_revision.h" 00042 #include "common.h" 00043 00044 //------------------------------------------------------------------------------ 00045 // Control Program 00046 //------------------------------------------------------------------------------ 00047 int main() 00048 { 00049 HTS221::HTS221_data_t hum_data; 00050 00051 usb_serial_initialize(); 00052 print_revision(); 00053 i2c_pullup = 1; 00054 sen_pwr = 1; 00055 print_usb("Check LSM9DS1\r\n"); 00056 ThisThread::sleep_for(200ms); 00057 // check I2C line 00058 check_i2c_connected_devices(); 00059 hum = new HTS221(PIN_SDA1, PIN_SCL1,HTS221::HTS221_ADDRESS, 400000); 00060 hum->HTS221_GetDeviceID (&hum_data); 00061 uint8_t id = hum_data.deviceID; 00062 if (id == HTS221::WHO_AM_I_VALUE) { 00063 print_usb("HTS221 is ready. ID = 0x%x\r\n", id); 00064 } else { 00065 print_usb("HTS221 is NOT ready. return value = 0x%x\r\n", id); 00066 } 00067 uint32_t n = 0; 00068 while(true) { 00069 t.reset(); 00070 t.start(); 00071 hum->HTS221_SetOneShot(); 00072 do { 00073 thread_sleep_for(2); 00074 hum->HTS221_GetOneShot(&hum_data); 00075 } while(hum_data.one_shot == HTS221::CTRL_REG2_ONE_SHOT_WAITING); 00076 hum->HTS221_GetCalibrationCoefficients (&hum_data); 00077 hum->HTS221_GetTemperature(&hum_data); 00078 hum->HTS221_GetHumidity(&hum_data); 00079 print_usb("Temperature:,%0.1f,[degC],RH:,%0.1f,[%%],", 00080 hum_data.temperature, hum_data.humidity); 00081 ++n; 00082 uint32_t passed_time = chrono::duration_cast<chrono::milliseconds>( 00083 t.elapsed_time()).count(); 00084 print_usb("processing time:,%2d,[ms],count:,%4d\r\n", passed_time, n); 00085 if (passed_time < 999){ 00086 ThisThread::sleep_for(chrono::milliseconds(1000 - passed_time)); 00087 } 00088 } 00089 } 00090 00091 #endif // EXAMPLE_4_CHECK_HTS221
Generated on Thu Jul 14 2022 06:04:06 by
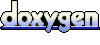