
Arduino board run on Mbed-os6.8.1 (only test purpose not official). Need Mbed Studio(1.3.1) not online compiler. If you compile on the online compiler, you can get a hex file but it does NOT work!!!
Dependencies: APDS_9960 LPS22HB LSM9DS1 HTS221
common.h
00001 /* 00002 * Nano 33 BLE Sense 00003 * Arudiono nRF52840 module 00004 * Common functions 00005 * 00006 * Copyright (c) 2020,'21 Kenji Arai / JH1PJL 00007 * http://www7b.biglobe.ne.jp/~kenjia/ 00008 * https://os.mbed.com/users/kenjiArai/ 00009 * Started: January 22nd, 2020 00010 * Revised: February 28th, 2021 00011 * 00012 */ 00013 00014 // Pre-selection -------------------------------------------------------------- 00015 #include "select_example.h" 00016 //#define USE_COMMON_FUNCTION 00017 #ifdef USE_COMMON_FUNCTION 00018 00019 // Include -------------------------------------------------------------------- 00020 #include "mbed.h" 00021 #include "nano33blesense_iodef.h" 00022 #include "LPS22HB.h" 00023 #include "LSM9DS1.h" 00024 #include "HTS221.h" 00025 //#include "glibr.h" // define as follows 00026 00027 // Definition ----------------------------------------------------------------- 00028 /* APDS-9960 I2C address */ 00029 #define APDS9960_I2C_ADDR 0x39 00030 /* APDS-9960 register */ 00031 #define APDS9960_ID 0x92 00032 /* Acceptable device IDs */ 00033 #define APDS9960_ID_1 0xAB 00034 #define APDS9960_ID_2 0x9C 00035 00036 // Constructor ---------------------------------------------------------------- 00037 00038 // RAM ------------------------------------------------------------------------ 00039 00040 // ROM / Constant data -------------------------------------------------------- 00041 00042 // Function prototypes -------------------------------------------------------- 00043 00044 //------------------------------------------------------------------------------ 00045 // Control Program 00046 //------------------------------------------------------------------------------ 00047 void check_i2c_connected_devices(void) 00048 { 00049 char dt[2]; 00050 int status; 00051 00052 // Check I2C line 00053 i2c.frequency(400000); 00054 dt[0] = 0; 00055 print_usb("check I2C device --> START\r\n"); 00056 for (uint8_t i = 0; i < 0x80; i++) { 00057 int addr = i << 1; 00058 status = i2c.write(addr, dt, 1, true); 00059 if (status == 0) { 00060 print_usb("Get ACK form address = 0x%x\r\n", i); 00061 } 00062 } 00063 } 00064 00065 void check_i2c_sensors(void) 00066 { 00067 char dt[2]; 00068 00069 // LSM9DS1 00070 dt[0] = WHO_AM_I_XG; 00071 int addr = LSM9DS1_AG_I2C_ADDR(1); 00072 i2c.write(addr, dt, 1, true); 00073 dt[0] = 0; 00074 i2c.read(addr, dt, 1, false); 00075 print_usb("LSM9DS1_AG is "); 00076 if (dt[0] != WHO_AM_I_AG_RSP) { 00077 print_usb("NOT "); 00078 } 00079 print_usb("available -> 0x%x = 0x%x\r\n", addr >> 1, dt[0]); 00080 dt[0] = WHO_AM_I_M; 00081 addr = LSM9DS1_M_I2C_ADDR(1); 00082 i2c.write(addr, dt, 1, true); 00083 dt[0] = 0; 00084 i2c.read(addr, dt, 1, false); 00085 print_usb("LSM9DS1_M is "); 00086 if (dt[0] != WHO_AM_I_M_RSP) { 00087 print_usb("NOT "); 00088 } 00089 print_usb("available -> 0x%x = 0x%x\r\n", addr >> 1, dt[0]); 00090 // LPS22HB 00091 dt[0] = LPS22HB_WHO_AM_I; 00092 addr = LPS22HB_G_CHIP_ADDR; 00093 i2c.write(addr, dt, 1, true); 00094 dt[0] = 0; 00095 i2c.read(addr, dt, 1, false); 00096 print_usb("LPS22HB is "); 00097 if (dt[0] != I_AM_LPS22HB) { 00098 print_usb("NOT "); 00099 } 00100 print_usb("available -> 0x%x = 0x%x\r\n", addr >> 1, dt[0]); 00101 // HTS221 00102 dt[0] = HTS221::HTS221_WHO_AM_I; 00103 addr = HTS221::HTS221_ADDRESS; 00104 i2c.write(addr, dt, 1, true); 00105 dt[0] = 0; 00106 i2c.read(addr, dt, 1, false); 00107 print_usb("HTS221 is "); 00108 if (dt[0] != HTS221::WHO_AM_I_VALUE) { 00109 print_usb("NOT "); 00110 } 00111 print_usb("available -> 0x%x = 0x%x\r\n", addr >> 1, dt[0]); 00112 // APDS_9960 00113 dt[0] = APDS9960_ID; 00114 addr = APDS9960_I2C_ADDR << 1; 00115 i2c.write(addr, dt, 1, true); 00116 dt[0] = 0; 00117 i2c.read(addr, dt, 1, false); 00118 print_usb("APDS_9960 is "); 00119 if (dt[0] == APDS9960_ID_1 || dt[0] == APDS9960_ID_2) { 00120 ; 00121 } else { 00122 print_usb("NOT "); 00123 } 00124 print_usb("available -> 0x%x = 0x%x\r\n", addr >> 1, dt[0]); 00125 } 00126 00127 #endif // USE_COMMON_FUNCTION
Generated on Thu Jul 14 2022 06:04:06 by
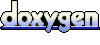