
a simple code for elevator
Dependencies: PinDetect mbed Servo
speed.cpp
00001 /* 00002 * PROGRAM RESPONSIBLE FOR open/close door. 00003 * ^^^^^^^ ^^^^^^^^^^^ ^^^ ^^^^^^^^^^ ^^^^ 00004 * 00005 * FILE NAME: speed.cpp 00006 * USAGE: program that control the speed of servo motor. 00007 */ 00008 00009 00010 /* 00011 * including the wanted library files. 00012 */ 00013 #include "mbed.h" 00014 #include "speed.h" 00015 #include "Servo.h" 00016 00017 /*--------------------------*\ 00018 * defineding the variables* 00019 \*--------------------------*/ 00020 float varying_speed; 00021 float speed_now; 00022 int delta_time; 00023 00024 Ticker motor_timer;/* using ticker class which repeatedly call a function*/ 00025 Servo motor_speed(p26); // Servo PinName (pin) 00026 00027 void motor (float speed, int time) { // void FunctionName (speed between 0-1, time in mS) 00028 // function responsible of increasing or decreasing the speed of the elevator softly 00029 if (time) { 00030 varying_speed= (speed - speed_now)/float (time); 00031 delta_time=time; 00032 /*---- Print message: shows motor speed at this point ------------------------------------*/ 00033 printf("Motor going to speed %f over %f seconds\n",speed,float(time)/1000); 00034 } else { 00035 motor_speed = speed; 00036 /*---- Print message: shows motor speed at this point ------------------------------------*/ 00037 printf("Motor at speed %f now\n",speed); 00038 speed_now=speed; 00039 } 00040 } 00041 00042 void motor_update (void) { // function responsible of checking the speed is within servo rang (0-1) 00043 if (delta_time) { 00044 00045 speed_now = speed_now + varying_speed; // event at reverst direction, this formela is removing the minus (all the result is positive) 00046 /* putting the speed in rang between 0 to 1 */ 00047 if (speed_now>1.0) { 00048 speed_now =1; 00049 } 00050 if (speed_now<0.0) { 00051 speed_now=0; 00052 } 00053 motor_speed=speed_now; 00054 delta_time--; // decrease the time by 1 00055 } 00056 } 00057 00058 void motor_start (void) {// a ticker function for the LED doors. 00059 speed_now = 0.5; 00060 motor_speed=speed_now; // stop the motor 00061 delta_time=0; // at 0S time 00062 motor_timer.attach(&motor_update,0.001);// repeatedly call "motor_update" function. 00063 }
Generated on Sat Jul 16 2022 02:23:42 by
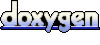