
a simple code for elevator
Dependencies: PinDetect mbed Servo
PIN_DETECT.cpp
00001 /* 00002 * SWITCH DEBOUNCING 00003 * ^^^^^^ ^^^^^^^^^^ 00004 * 00005 * FILE NAME: PIN_DETECT.cpp 00006 * USAGE: Extends DigitalIn to add mechanical switch deboucing of inputs. 00007 */ 00008 00009 00010 /* 00011 * including the wanted library files. 00012 */ 00013 #include "mbed.h" 00014 #include "main.h" 00015 #include "PIN_DETECT.h" 00016 #include "PinDetect.h" 00017 00018 /******************************************************************************** 00019 ********************************************************************************* 00020 ******* WARNING: to used this file, PinDetect library must be import ************ 00021 ******* into the program ************ 00022 ********************************************************************************* 00023 *********************************************************************************/ 00024 00025 PinDetect top_SW (p13); 00026 PinDetect NR_top_SW (p14); 00027 PinDetect bottom_SW (p15); 00028 PinDetect NR_bottom_SW (p16); 00029 PinDetect top_call (p17); 00030 PinDetect bottom_call (p19); 00031 PinDetect goto_top (p20); 00032 PinDetect goto_bottom (p21); 00033 PinDetect safety_button (p22); 00034 00035 /*>>>>>>>>>>>>>>>>>>>>>>>>>>>>> functions callbacks follow <<<<<<<<<<<<<<<<<<<<<<<<<<<<*/ 00036 void SW_1 (void) { /* top switch pressed*/ 00037 new_event(at_top); 00038 } 00039 00040 void SW_2 (void) { /*near top switch pressed*/ 00041 new_event(NR_top); 00042 } 00043 00044 void SW_3(void) { /*near bottom switch pressed*/ 00045 new_event(NR_bottom); 00046 } 00047 00048 void SW_4 (void) { /*bottom switch pressed*/ 00049 new_event(at_bottom); 00050 } 00051 00052 void SW_5 (void) { /*top call button pressed*/ 00053 new_event(call_2); 00054 flag_call_2=1; 00055 } 00056 00057 void SW_6 (void) { /*bottom call button pressed*/ 00058 new_event(call_1); 00059 flag_call_1=1; 00060 } 00061 00062 void SW_7 (void) { /*go to top button*/ 00063 new_event(B_level_2); 00064 } 00065 00066 void SW_8 (void) { /*go to bottom button*/ 00067 new_event(B_level_1); 00068 } 00069 00070 void SW_9 (void) { /*safety switch*/ 00071 new_event(safety); 00072 } 00073 00074 // C function for pin detect 00075 00076 void switches (void) { 00077 // Debouncing >>>> 00078 00079 // for switch 1 (top switch) 00080 top_SW.mode( PullUp ); 00081 top_SW.setAssertValue(0); 00082 top_SW.attach_asserted( &SW_1 ); 00083 top_SW.setSampleFrequency(); 00084 00085 00086 // for switch 2 (near top switch) 00087 NR_top_SW.mode( PullUp ); 00088 NR_top_SW.setAssertValue(0); 00089 NR_top_SW.attach_asserted( &SW_2 ); 00090 NR_top_SW.setSampleFrequency();// Defaults to 20ms. 00091 00092 00093 // for switch 3 (near bottom switch) 00094 bottom_SW.mode( PullUp ); 00095 bottom_SW.setAssertValue(0); 00096 bottom_SW.attach_asserted( &SW_3 ); 00097 bottom_SW.setSampleFrequency();// Defaults to 20ms. 00098 00099 00100 // for switch 4 (bottom switch) 00101 NR_bottom_SW.mode( PullUp ); 00102 NR_bottom_SW.setAssertValue(0); 00103 NR_bottom_SW.attach_asserted( &SW_4 ); 00104 NR_bottom_SW.setSampleFrequency();// Defaults to 20ms. 00105 00106 00107 // for switch 5 (top call switch) 00108 top_call.mode( PullUp ); 00109 top_call.setAssertValue(0); 00110 top_call.attach_asserted( &SW_5 ); 00111 top_call.setSampleFrequency();// Defaults to 20ms. 00112 00113 00114 // for switch 6 (bottom call switch) 00115 bottom_call.mode( PullUp ); 00116 bottom_call.setAssertValue(0); 00117 bottom_call.attach_asserted( &SW_6 ); 00118 bottom_call.setSampleFrequency();// Defaults to 20ms. 00119 00120 00121 // for switch 7 (level select button) 00122 goto_top.mode( PullUp ); 00123 goto_top.setAssertValue(0); 00124 goto_top.attach_asserted( &SW_7 ); 00125 goto_top.setSampleFrequency();// Defaults to 20ms. 00126 00127 00128 // for switch 8 (level select button) 00129 goto_bottom.mode( PullUp ); 00130 goto_bottom.setAssertValue(0); 00131 goto_bottom.attach_asserted( &SW_8 ); 00132 goto_bottom.setSampleFrequency();// Defaults to 20ms. 00133 00134 00135 // for switch 9 (safety switch) 00136 safety_button.mode( PullUp ); 00137 safety_button.setAssertValue(0); 00138 safety_button.attach_asserted( &SW_9 ); 00139 safety_button.setSampleFrequency();// Defaults to 20ms. 00140 } 00141 00142 00143 /******************************************************************************** 00144 ********************************************************************************* 00145 ******* for more detail about switch depancing program vist ************* 00146 ******* http://mbed.org/users/AjK/libraries/PinDetect/lkyxpw ************* 00147 ********************************************************************************* 00148 *********************************************************************************/
Generated on Sat Jul 16 2022 02:23:42 by
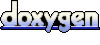