
a simple code for elevator
Dependencies: PinDetect mbed Servo
LED.cpp
00001 /* 00002 * PROGRAM RESPONSIBLE FOR open/close door. 00003 * ^^^^^^^ ^^^^^^^^^^^ ^^^ ^^^^^^^^^^ ^^^^ 00004 * 00005 * FILE NAME: LED.cpp 00006 * USAGE: program that control 16 LED's that indicate the position of the doors. 00007 */ 00008 00009 00010 /* 00011 * including the wanted library files. 00012 */ 00013 #include "mbed.h" 00014 #include "LED.h" 00015 #include "main.h" 00016 00017 /*--------------------------*\ 00018 * defineding the variables* 00019 \*--------------------------*/ 00020 int floor_level; 00021 int LED_order; 00022 00023 DigitalOut latch(p8); // control a digital output pin.. 00024 00025 SPI spi(p5, p6, p7); // SPI bus master; mosi, miso, sclk. 00026 Ticker door_timer; /* using ticker class which repeatedly call a function*/ 00027 00028 void open_close_doors ( int f) { // a function that defined the number of elements and selecting a floor 00029 floor_level=f; // for selecting the wanted floor (0, 1) 00030 LED_order=28; // max. number of the elements 00031 } 00032 00033 void doors (void) { // a function responsible of turning the LEDs on/off 00034 if (LED_order) { 00035 spi.write(door_light[floor_level][--LED_order]); //Write to the SPI Slave; Send the command. 00036 latch=1;// Deselect the device 00037 latch=0;// Select the device by seting chip select low 00038 if (LED_order==0) // when the LED order reach 0, a new event will be called (timeout) 00039 new_event(timeout); 00040 } 00041 } 00042 00043 void doors_start (void) {// a ticker function for the LED doors. 00044 spi.format(16,0); 00045 latch =0;// Select the device by seting chip select low 00046 spi.write(0xFFFF); //Write to the SPI Slave; Send (0xFFFF)the command. 00047 latch=1;// Deselect the device 00048 latch=0; 00049 LED_order=0; 00050 door_timer.attach(&doors,0.2);// repeatedly call "doors" function. 00051 }
Generated on Sat Jul 16 2022 02:23:42 by
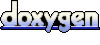