
LoRa sending TSL lux sensor
Dependencies: TSL2561_I2C libmDot-mbed5
main.cpp
00001 /* 00002 This program: 00003 - connects to a LoRaWAN by ABP/MANUAL 00004 - reads light data from an TSL2561 abient light sensor 00005 - sends the recorded data onto the LoRaWAN 00006 - sets the mDot to sleep 00007 - repeats these operations in a loop 00008 */ 00009 00010 #include "mbed.h" 00011 #include "mDot.h" 00012 #include "ChannelPlans.h" 00013 #include "MTSLog.h" 00014 #include "dot_util.h" 00015 #include "mbed.h" 00016 #include "TSL2561_I2C.h" 00017 #include <string> 00018 #include <vector> 00019 #include <algorithm> 00020 #include <sstream> 00021 00022 00023 #define TSL_SDA_PIN PC_9 00024 #define TSL_SCL_PIN PA_8 00025 //TSL2561_I2C(inName sda, PinName scl) 00026 TSL2561_I2C tsl(TSL_SDA_PIN, TSL_SCL_PIN); 00027 00028 // these options must match the settings on your gateway/server 00029 /* 00030 Current test settings 00031 dev address: 072389f7 00032 net sess key: b35aca73d283996dc3cbc0803af04547 00033 app sess key: d6f28430da4035273b9e3c07eb30c0dd 00034 */ 00035 //device address 00036 static uint8_t network_address[] = { 0x07, 0x23, 0x89, 0xf7 }; 00037 //network session key 00038 static uint8_t network_session_key[] = { 0xb3, 0x5a, 0xca, 0x73, 0xd2, 0x83, 0x99, 0x6d, 0xc3, 0xcb, 0xc0, 0x80, 0x3a, 0xf0, 0x45, 0x47 }; 00039 //application sesssion or data session key 00040 static uint8_t data_session_key[] = { 0xd6, 0xf2, 0x84, 0x30, 0xda, 0x40, 0x35, 0x27, 0x3b, 0x9e, 0x3c, 0x07, 0xeb, 0x30, 0xc0, 0xdd }; 00041 static uint8_t frequency_sub_band = 2; //VFI 00042 static bool public_network = true; 00043 //enable receipt of ackknowledge packets 0 = No, 1 = Yes 00044 static uint8_t ack = 0; 00045 //adaptive data rate enabler 00046 static bool adr = false; 00047 00048 //USB serial 00049 Serial pc(USBTX, USBRX); 00050 00051 //get ourselves an mDot pointer - we will assign to it in main() 00052 mDot* dot = NULL; 00053 00054 //converts value to string 00055 template <typename T> 00056 string ToString(T val) { 00057 stringstream stream; 00058 stream << val; 00059 return stream.str(); 00060 } 00061 00062 int main() { 00063 //setting serial rate 00064 pc.baud(9600); 00065 00066 // use AU915 plan 00067 lora::ChannelPlan* plan = new lora::ChannelPlan_AU915(); 00068 assert(plan); 00069 // get a mDot handle with the plan we chose 00070 dot = mDot::getInstance(plan); 00071 assert(dot); 00072 00073 if (!dot->getStandbyFlag()) { 00074 logInfo("mbed-os library version: %d", MBED_LIBRARY_VERSION); 00075 00076 // start from a well-known state 00077 logInfo("defaulting Dot configuration"); 00078 dot->resetConfig(); 00079 dot->resetNetworkSession(); 00080 00081 // make sure library logging is turned on 00082 dot->setLogLevel(mts::MTSLog::DEBUG_LEVEL); 00083 00084 // update configuration if necessary 00085 if (dot->getJoinMode() != mDot::MANUAL) { 00086 logInfo("changing network join mode to MANUAL"); 00087 if (dot->setJoinMode(mDot::MANUAL) != mDot::MDOT_OK) { 00088 logError("failed to set network join mode to MANUAL"); 00089 } 00090 } 00091 // in MANUAL join mode there is no join request/response transaction 00092 // as long as the Dot is configured correctly and provisioned correctly on the gateway, it should be able to communicate 00093 // network address - 4 bytes (00000001 - FFFFFFFE) 00094 // network session key - 16 bytes 00095 // data session key - 16 bytes 00096 // to provision your Dot with a Conduit gateway, follow the following steps 00097 // * ssh into the Conduit 00098 // * provision the Dot using the lora-query application: http://www.multitech.net/developer/software/lora/lora-network-server/ 00099 // lora-query -a 01020304 A 0102030401020304 <your Dot's device ID> 01020304010203040102030401020304 01020304010203040102030401020304 00100 // * if you change the network address, network session key, or data session key, make sure you update them on the gateway 00101 // to provision your Dot with a 3rd party gateway, see the gateway or network provider documentation 00102 update_manual_config(network_address, network_session_key, data_session_key, frequency_sub_band, public_network, ack); 00103 00104 00105 // enable or disable Adaptive Data Rate 00106 dot->setAdr(adr); 00107 00108 //* AU915 Datarates 00109 //* --------------- 00110 //* DR0 - SF10BW125 -- 11 bytes 00111 //* DR1 - SF9BW125 -- 53 bytes 00112 //* DR2 - SF8BW125 -- 129 byte 00113 //* DR3 - SF7BW125 -- 242 bytes 00114 //* DR4 - SF8BW500 -- 242 bytes 00115 dot->setTxDataRate(mDot::DR2); 00116 00117 // save changes to configuration 00118 logInfo("saving configuration"); 00119 if (!dot->saveConfig()) { 00120 logError("failed to save configuration"); 00121 } 00122 00123 // display configuration 00124 display_config(); 00125 } else { 00126 // restore the saved session if the dot woke from deepsleep mode 00127 // useful to use with deepsleep because session info is otherwise lost when the dot enters deepsleep 00128 logInfo("restoring network session from NVM"); 00129 dot->restoreNetworkSession(); 00130 } 00131 00132 //set gain level on lux sensor 00133 //setgain accepts 1 or 16 - 1 for normal, 16 for very low light 00134 logInfo("Gain return %d", tsl.setGain(1)); //16 seems to cause NaNs 00135 00136 //this is where the magic happens 00137 while (true) { 00138 00139 //init data variable 00140 std::vector<uint8_t> data; 00141 00142 //read Lux Level 00143 //thank you https://developer.mbed.org/users/merckeng/code/mDot_TTN_DHT11_Boston16_CAM/file/a2c9c4cc4863/main.cpp 00144 tsl.enablePower(); 00145 //float lux = tsl.getLux(); 00146 //logInfo("Lux level: %.4f", lux); 00147 00148 00149 int lux2 = tsl.getVisibleAndIR(); 00150 logInfo("Lux level: %d", lux2); 00151 //put data in string 00152 string output = "D206.Lux: " + ToString(lux2); 00153 00154 //serial output for debugging 00155 logInfo("Sending %s", output.c_str()); 00156 00157 // format data for sending to the gateway 00158 for (std::string::iterator it = output.begin(); it != output.end(); it++) 00159 data.push_back((uint8_t) *it); 00160 00161 //now send 00162 send_data(data); 00163 00164 // go to sleep and wake up automatically sleep_time seconds later 00165 uint32_t sleep_time = 60; 00166 //false is "don't deep sleep" - mDot doesn't do that 00167 dot->sleep(sleep_time, mDot::RTC_ALARM, false); 00168 } 00169 00170 return 0; //shouldn't happen 00171 } 00172
Generated on Mon Jul 18 2022 20:08:11 by
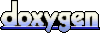