
Uses the APDS_9960 Digital Proximity, Ambient Light, RGB and Gesture Sensor library to play detected gesture sounds on a speaker from the SDcard
Dependencies: mbed SDFileSystem wave_player
glibr.h
00001 #include "mbed.h" 00002 00003 00004 #define DEBUG 0 00005 00006 /* APDS-9960 I2C address */ 00007 #define APDS9960_I2C_ADDR 0x39 00008 00009 /* Gesture parameters */ 00010 #define GESTURE_THRESHOLD_OUT 10 00011 #define GESTURE_SENSITIVITY_1 50 00012 #define GESTURE_SENSITIVITY_2 20 00013 00014 /* Error code for returned values */ 00015 #define ERROR 0xFF 00016 00017 /* Acceptable device IDs */ 00018 #define APDS9960_ID_1 0xAB 00019 #define APDS9960_ID_2 0x9C 00020 00021 /* Misc parameters */ 00022 #define FIFO_PAUSE_TIME 0.30 // Wait period (ms) between FIFO reads 00023 00024 /* APDS-9960 register addresses */ 00025 #define APDS9960_ENABLE 0x80 00026 #define APDS9960_ATIME 0x81 00027 #define APDS9960_WTIME 0x83 00028 #define APDS9960_AILTL 0x84 00029 #define APDS9960_AILTH 0x85 00030 #define APDS9960_AIHTL 0x86 00031 #define APDS9960_AIHTH 0x87 00032 #define APDS9960_PILT 0x89 00033 #define APDS9960_PIHT 0x8B 00034 #define APDS9960_PERS 0x8C 00035 #define APDS9960_CONFIG1 0x8D 00036 #define APDS9960_PPULSE 0x8E 00037 #define APDS9960_CONTROL 0x8F 00038 #define APDS9960_CONFIG2 0x90 00039 #define APDS9960_ID 0x92 00040 #define APDS9960_STATUS 0x93 00041 #define APDS9960_CDATAL 0x94 00042 #define APDS9960_CDATAH 0x95 00043 #define APDS9960_RDATAL 0x96 00044 #define APDS9960_RDATAH 0x97 00045 #define APDS9960_GDATAL 0x98 00046 #define APDS9960_GDATAH 0x99 00047 #define APDS9960_BDATAL 0x9A 00048 #define APDS9960_BDATAH 0x9B 00049 #define APDS9960_PDATA 0x9C 00050 #define APDS9960_POFFSET_UR 0x9D 00051 #define APDS9960_POFFSET_DL 0x9E 00052 #define APDS9960_CONFIG3 0x9F 00053 #define APDS9960_GPENTH 0xA0 00054 #define APDS9960_GEXTH 0xA1 00055 #define APDS9960_GCONF1 0xA2 00056 #define APDS9960_GCONF2 0xA3 00057 #define APDS9960_GOFFSET_U 0xA4 00058 #define APDS9960_GOFFSET_D 0xA5 00059 #define APDS9960_GOFFSET_L 0xA7 00060 #define APDS9960_GOFFSET_R 0xA9 00061 #define APDS9960_GPULSE 0xA6 00062 #define APDS9960_GCONF3 0xAA 00063 #define APDS9960_GCONF4 0xAB 00064 #define APDS9960_GFLVL 0xAE 00065 #define APDS9960_GSTATUS 0xAF 00066 #define APDS9960_IFORCE 0xE4 00067 #define APDS9960_PICLEAR 0xE5 00068 #define APDS9960_CICLEAR 0xE6 00069 #define APDS9960_AICLEAR 0xE7 00070 #define APDS9960_GFIFO_U 0xFC 00071 #define APDS9960_GFIFO_D 0xFD 00072 #define APDS9960_GFIFO_L 0xFE 00073 #define APDS9960_GFIFO_R 0xFF 00074 00075 /* Bit fields */ 00076 #define APDS9960_PON 0x01 00077 #define APDS9960_AEN 0x02 00078 #define APDS9960_PEN 0x04 00079 #define APDS9960_WEN 0x08 00080 #define APSD9960_AIEN 0x10 00081 #define APDS9960_PIEN 0x20 00082 #define APDS9960_GEN 0x40 00083 #define APDS9960_GVALID 0x01 00084 00085 /* On/Off definitions */ 00086 #define OFF 0 00087 #define ON 1 00088 00089 /* Acceptable parameters for setMode */ 00090 #define POWER 0 00091 #define AMBIENT_LIGHT 1 00092 #define PROXIMITY 2 00093 #define WAIT 3 00094 #define AMBIENT_LIGHT_INT 4 00095 #define PROXIMITY_INT 5 00096 #define GESTURE 6 00097 #define ALL 7 00098 00099 /* LED Drive values */ 00100 #define LED_DRIVE_100MA 0 00101 #define LED_DRIVE_50MA 1 00102 #define LED_DRIVE_25MA 2 00103 #define LED_DRIVE_12_5MA 3 00104 00105 /* Proximity Gain (PGAIN) values */ 00106 #define PGAIN_1X 0 00107 #define PGAIN_2X 1 00108 #define PGAIN_4X 2 00109 #define PGAIN_8X 3 00110 00111 /* ALS Gain (AGAIN) values */ 00112 #define AGAIN_1X 0 00113 #define AGAIN_4X 1 00114 #define AGAIN_16X 2 00115 #define AGAIN_64X 3 00116 00117 /* Gesture Gain (GGAIN) values */ 00118 #define GGAIN_1X 0 00119 #define GGAIN_2X 1 00120 #define GGAIN_4X 2 00121 #define GGAIN_8X 3 00122 00123 /* LED Boost values */ 00124 #define LED_BOOST_100 0 00125 #define LED_BOOST_150 1 00126 #define LED_BOOST_200 2 00127 #define LED_BOOST_300 3 00128 00129 /* Gesture wait time values */ 00130 #define GWTIME_0MS 0 00131 #define GWTIME_2_8MS 1 00132 #define GWTIME_5_6MS 2 00133 #define GWTIME_8_4MS 3 00134 #define GWTIME_14_0MS 4 00135 #define GWTIME_22_4MS 5 00136 #define GWTIME_30_8MS 6 00137 #define GWTIME_39_2MS 7 00138 00139 /* Default values */ 00140 #define DEFAULT_ATIME 219 // 103ms 00141 #define DEFAULT_WTIME 246 // 27ms 00142 #define DEFAULT_PROX_PPULSE 0x87 // 16us, 8 pulses 00143 #define DEFAULT_GESTURE_PPULSE 0x89 // 16us, 10 pulses 00144 #define DEFAULT_POFFSET_UR 0 // 0 offset 00145 #define DEFAULT_POFFSET_DL 0 // 0 offset 00146 #define DEFAULT_CONFIG1 0x60 // No 12x wait (WTIME) factor 00147 #define DEFAULT_LDRIVE LED_DRIVE_100MA 00148 #define DEFAULT_PGAIN PGAIN_4X 00149 #define DEFAULT_AGAIN AGAIN_4X 00150 #define DEFAULT_PILT 0 // Low proximity threshold 00151 #define DEFAULT_PIHT 50 // High proximity threshold 00152 #define DEFAULT_AILT 0xFFFF // Force interrupt for calibration 00153 #define DEFAULT_AIHT 0 00154 #define DEFAULT_PERS 0x11 // 2 consecutive prox or ALS for int. 00155 #define DEFAULT_CONFIG2 0x01 // No saturation interrupts or LED boost 00156 #define DEFAULT_CONFIG3 0 // Enable all photodiodes, no SAI 00157 #define DEFAULT_GPENTH 40 // Threshold for entering gesture mode 00158 #define DEFAULT_GEXTH 30 // Threshold for exiting gesture mode 00159 #define DEFAULT_GCONF1 0x40 // 4 gesture events for int., 1 for exit 00160 #define DEFAULT_GGAIN GGAIN_4X 00161 #define DEFAULT_GLDRIVE LED_DRIVE_100MA 00162 #define DEFAULT_GWTIME GWTIME_2_8MS 00163 #define DEFAULT_GOFFSET 0 // No offset scaling for gesture mode 00164 #define DEFAULT_GPULSE 0xC9 // 32us, 10 pulses 00165 #define DEFAULT_GCONF3 0 // All photodiodes active during gesture 00166 #define DEFAULT_GIEN 0 // Disable gesture interrupts 00167 00168 /* Direction definitions */ 00169 enum { 00170 DIR_NONE, 00171 DIR_LEFT, 00172 DIR_RIGHT, 00173 DIR_UP, 00174 DIR_DOWN, 00175 DIR_NEAR, 00176 DIR_FAR, 00177 DIR_ALL 00178 }; 00179 00180 /* State definitions */ 00181 enum { 00182 NA_STATE, 00183 NEAR_STATE, 00184 FAR_STATE, 00185 ALL_STATE 00186 }; 00187 00188 /* Container for gesture data */ 00189 typedef struct gesture_data_type { 00190 uint8_t u_data[32]; 00191 uint8_t d_data[32]; 00192 uint8_t l_data[32]; 00193 uint8_t r_data[32]; 00194 uint8_t sindex; 00195 uint8_t total_gestures; 00196 uint8_t in_threshold; 00197 uint8_t out_threshold; 00198 } gesture_data_type; 00199 00200 00201 class glibr{ 00202 00203 public: 00204 00205 00206 glibr(PinName sda, PinName scl); //constructor 00207 ~glibr(); 00208 00209 00210 bool ginit(); 00211 /* Initialization methods */ 00212 00213 uint8_t getMode(); 00214 bool setMode(uint8_t mode, uint8_t enable); 00215 00216 /* Turn the APDS-9960 on and off */ 00217 bool enablePower(); 00218 bool disablePower(); 00219 00220 /* Enable or disable specific sensors */ 00221 bool enableLightSensor(bool interrupts = false); 00222 bool disableLightSensor(); 00223 bool enableProximitySensor(bool interrupts = false); 00224 bool disableProximitySensor(); 00225 bool enableGestureSensor(bool interrupts = true); 00226 bool disableGestureSensor(); 00227 00228 /* LED drive strength control */ 00229 uint8_t getLEDDrive(); 00230 bool setLEDDrive(uint8_t drive); 00231 uint8_t getGestureLEDDrive(); 00232 bool setGestureLEDDrive(uint8_t drive); 00233 00234 /* Gain control */ 00235 uint8_t getAmbientLightGain(); 00236 bool setAmbientLightGain(uint8_t gain); 00237 uint8_t getProximityGain(); 00238 bool setProximityGain(uint8_t gain); 00239 uint8_t getGestureGain(); 00240 bool setGestureGain(uint8_t gain); 00241 00242 /* Get and set light interrupt thresholds */ 00243 bool getLightIntLowThreshold(uint16_t &threshold); 00244 bool setLightIntLowThreshold(uint16_t threshold); 00245 bool getLightIntHighThreshold(uint16_t &threshold); 00246 bool setLightIntHighThreshold(uint16_t threshold); 00247 00248 /* Get and set proximity interrupt thresholds */ 00249 bool getProximityIntLowThreshold(uint8_t &threshold); 00250 bool setProximityIntLowThreshold(uint8_t threshold); 00251 bool getProximityIntHighThreshold(uint8_t &threshold); 00252 bool setProximityIntHighThreshold(uint8_t threshold); 00253 00254 /* Get and set interrupt enables */ 00255 uint8_t getAmbientLightIntEnable(); 00256 bool setAmbientLightIntEnable(uint8_t enable); 00257 uint8_t getProximityIntEnable(); 00258 bool setProximityIntEnable(uint8_t enable); 00259 uint8_t getGestureIntEnable(); 00260 bool setGestureIntEnable(uint8_t enable); 00261 00262 /* Clear interrupts */ 00263 bool clearAmbientLightInt(); 00264 bool clearProximityInt(); 00265 00266 /* Ambient light methods */ 00267 bool readAmbientLight(uint16_t &val); 00268 bool readRedLight(uint16_t &val); 00269 bool readGreenLight(uint16_t &val); 00270 bool readBlueLight(uint16_t &val); 00271 00272 /* Proximity methods */ 00273 bool readProximity(uint8_t &val); 00274 00275 /* Gesture methods */ 00276 bool isGestureAvailable(); 00277 int readGesture(); 00278 00279 private: 00280 /* Gesture processing */ 00281 void resetGestureParameters(); 00282 bool processGestureData(); 00283 bool decodeGesture(); 00284 00285 /* Proximity Interrupt Threshold */ 00286 uint8_t getProxIntLowThresh(); 00287 bool setProxIntLowThresh(uint8_t threshold); 00288 uint8_t getProxIntHighThresh(); 00289 bool setProxIntHighThresh(uint8_t threshold); 00290 00291 /* LED Boost Control */ 00292 uint8_t getLEDBoost(); 00293 bool setLEDBoost(uint8_t boost); 00294 00295 /* Proximity photodiode select */ 00296 uint8_t getProxGainCompEnable(); 00297 bool setProxGainCompEnable(uint8_t enable); 00298 uint8_t getProxPhotoMask(); 00299 bool setProxPhotoMask(uint8_t mask); 00300 00301 /* Gesture threshold control */ 00302 uint8_t getGestureEnterThresh(); 00303 bool setGestureEnterThresh(uint8_t threshold); 00304 uint8_t getGestureExitThresh(); 00305 bool setGestureExitThresh(uint8_t threshold); 00306 00307 /* Gesture LED, gain, and time control */ 00308 uint8_t getGestureWaitTime(); 00309 bool setGestureWaitTime(uint8_t time); 00310 00311 /* Gesture mode */ 00312 uint8_t getGestureMode(); 00313 bool setGestureMode(uint8_t mode); 00314 00315 /* Members */ 00316 gesture_data_type gesture_data_; //instanciation from struct 00317 int gesture_ud_delta_; 00318 int gesture_lr_delta_; 00319 int gesture_ud_count_; 00320 int gesture_lr_count_; 00321 int gesture_near_count_; 00322 int gesture_far_count_; 00323 int gesture_state_; 00324 int gesture_motion_; 00325 00326 00327 00328 uint8_t I2CreadByte(char address, char subAddress); 00329 int I2CwriteByte(char address, char subAddress, char data); //if return value is 1, then ack not sent 00330 int I2CReadDataBlock(char address, char subAddress, char *data, unsigned int len); 00331 I2C i2c; 00332 00333 };
Generated on Wed Jul 13 2022 05:03:27 by
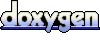