
Very simple example using ESP-WROOM-02 (ESP-8266) from LPC1114
Embed:
(wiki syntax)
Show/hide line numbers
ESP8266.h
00001 /** 00002 * Use ESP-8266 (called ESP-WROOM-02 in Japan) from LPC1114 00003 * LPC1114 has only 1 Hardware UART so this class uses SoftSerial to 00004 * communicate with ESP-8266. 00005 * I wrote like this class for Arduino then partially ported to mbed. 00006 * 00007 * 2016/05/13 00008 * By Kazuo Tsubaki 00009 **/ 00010 00011 #ifndef __ESP8266__ 00012 #define __ESP8266__ 00013 00014 #include <mbed.h> 00015 #include "SoftSerial.h" 00016 00017 class ESP8266 { 00018 public: 00019 00020 // Constructor 00021 ESP8266(SoftSerial *s); 00022 ~ESP8266(); 00023 00024 // Example for configuring ESP 00025 bool config(); 00026 // Use Serial to logging 00027 void setLogger(Serial *log); 00028 00029 // Connect to WiFi AP 00030 bool connect(char *ssid, char *password); 00031 // Send command to ESP-8266 (see AT command list of the module) 00032 void sendRequest(char *req); 00033 // Read resultant response of AT command. Needs some waits to read after AT command. 00034 char *readResponse(); 00035 00036 // AT 00037 bool nop(); 00038 // AT+GMR 00039 void version(); 00040 // AT+RST 00041 bool reset(); 00042 // AT+CWMODE=n n= 1:Station Mode, 2:AP Mode, 3:Station Mode + AP Mode 00043 bool mode(int mode); 00044 // AT+CIPMUX=n n= 0:Single, 1=Multi 00045 bool connectionMode(int connMode); 00046 // AT+CIPSTA? 00047 void connectionStatus(); 00048 00049 private: 00050 00051 void sendCmd(); 00052 void getReply(); 00053 bool _ok(); 00054 00055 private: 00056 00057 char cmdbuffer[266]; 00058 char replybuffer[1024]; 00059 SoftSerial *stream; 00060 int replycount, timeout, getcount; 00061 Timer t; 00062 Serial *logger; 00063 }; 00064 00065 00066 #endif
Generated on Thu Jul 14 2022 01:02:14 by
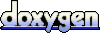