
mbed Geiger counter
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 00003 // OLED 00004 #include "MARMEX_OB_oled.h" 00005 MARMEX_OB_oled oled1( p5, p7, p27, p24, p26 ); // mosi, sclk, cs, rst, power_control 00006 00007 InterruptIn button(p29); 00008 DigitalOut beep(p30); 00009 00010 DigitalOut led(LED1); 00011 DigitalOut min(LED3); 00012 DigitalOut flash(LED4); 00013 00014 int total_cnt = 0; 00015 int cpm_cnt = 0; 00016 int cpm_old = 0; 00017 00018 void splash_oled(void) { 00019 oled1.background( 0x000000 ); 00020 oled1.cls(); 00021 00022 oled1.locate( 0, 0 ); 00023 oled1.printf( "GMT D3372 Count" ); 00024 00025 oled1.locate( 0, 1 ); 00026 oled1.printf( "SPI = %s", MERMEX_OB_SPI_MODE_STR ); 00027 00028 for (int s = 1 ; s < 8 ; s++) { 00029 oled1.fill(0, MARMEX_OB_oled::HEIGHT - (s * 10), MARMEX_OB_oled::WIDTH, 1, 0x000000ff); 00030 } 00031 } 00032 00033 void beep_on(void) { 00034 for (int i = 1; i < 100 ; i++) { 00035 beep = 1; 00036 wait_us(500); 00037 beep = 0; 00038 wait_us(500); 00039 } 00040 } 00041 00042 void flip() { 00043 led = !led; 00044 total_cnt++; 00045 cpm_cnt++; 00046 oled1.locate(0, 3); 00047 oled1.printf("Total:%d", total_cnt); 00048 00049 beep_on(); 00050 } 00051 00052 int main() { 00053 00054 int x = 0; 00055 00056 splash_oled(); 00057 00058 beep_on(); 00059 00060 button.rise(&flip); // attach the address of the flip function to the rising edge 00061 while(1) { 00062 if (cpm_cnt != 0) { 00063 cpm_old = cpm_cnt; 00064 } 00065 cpm_cnt = 0; 00066 for (int i = 1; i < 60; i++) { // wait around, interrupts will interrupt this! 00067 flash = !flash; // 1sec 00068 wait(1); 00069 } 00070 min = !min; // 1min 00071 oled1.locate(0,4); 00072 oled1.printf("%d cpm", cpm_old); 00073 00074 oled1.fill(x, MARMEX_OB_oled::HEIGHT - (cpm_old * 10), 1, (cpm_old * 10), 0x0000ff00); 00075 x++; 00076 if (x > MARMEX_OB_oled::WIDTH) { x = 0; } 00077 } 00078 }
Generated on Wed Jul 13 2022 22:59:04 by
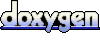