This is Wi-Fi interface of WizFi310 for mbed os 5
Dependents: mbed-os-example-wifi
WizFi310Interface.cpp
00001 /* WizFi310 implementation of NetworkInterfaceAPI 00002 * Copyright (c) 2015 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #include <string.h> 00017 #include "WizFi310Interface.h" 00018 #include "mbed_debug.h" 00019 00020 // Various timeouts for different WizFi310 operations 00021 #ifndef WIZFI310_CONNECT_TIMEOUT 00022 #define WIZFI310_CONNECT_TIMEOUT 15000 00023 #endif 00024 #ifndef WIZFI310_SEND_TIMEOUT 00025 #define WIZFI310_SEND_TIMEOUT 500 00026 #endif 00027 #ifndef WIZFI310_RECV_TIMEOUT 00028 #define WIZFI310_RECV_TIMEOUT 0 00029 #endif 00030 #ifndef WIZFI310_MISC_TIMEOUT 00031 #define WIZFI310_MISC_TIMEOUT 500 00032 #endif 00033 #ifndef WIZFI310_OPEN_CLOSE_TIMEOUT 00034 #define WIZFI310_OPEN_CLOSE_TIMEOUT 10000 00035 #endif 00036 00037 #ifndef WIZFI310_DELAY_MS 00038 #define WIZFI310_DELAY_MS 300 00039 #endif 00040 00041 // WizFi310Interface implementation 00042 WizFi310Interface::WizFi310Interface(PinName tx, PinName rx, bool debug) 00043 : _wizfi310(tx, rx, debug) 00044 { 00045 memset(_ids, 0, sizeof(_ids)); 00046 memset(_cbs, 0, sizeof(_cbs)); 00047 00048 _wizfi310.attach(this, &WizFi310Interface::event); 00049 } 00050 00051 int WizFi310Interface::connect(const char *ssid, const char *pass, nsapi_security_t security, 00052 uint8_t channel) 00053 { 00054 if (channel != 0) { 00055 return NSAPI_ERROR_UNSUPPORTED; 00056 } 00057 00058 set_credentials(ssid, pass, security); 00059 00060 return connect(); 00061 } 00062 00063 int WizFi310Interface::connect() 00064 { 00065 char sec[10]; 00066 00067 _wizfi310.setTimeout(WIZFI310_CONNECT_TIMEOUT); 00068 00069 _wizfi310.startup(0); 00070 00071 if( !_wizfi310.dhcp(true) ) 00072 { 00073 return NSAPI_ERROR_DHCP_FAILURE; 00074 } 00075 00076 switch( ap_sec ) 00077 { 00078 case NSAPI_SECURITY_NONE: 00079 strncpy(sec,"OPEN",strlen("OPEN")+1); 00080 break; 00081 case NSAPI_SECURITY_WEP: 00082 strncpy(sec,"WEP",strlen("WEP")+1); 00083 break; 00084 case NSAPI_SECURITY_WPA: 00085 strncpy(sec,"WPA",strlen("WPA")+1); 00086 break; 00087 case NSAPI_SECURITY_WPA2: 00088 strncpy(sec,"WPA2",strlen("WPA2")+1); 00089 break; 00090 case NSAPI_SECURITY_WPA_WPA2: 00091 strncpy(sec,"WPAWPA2",strlen("WPAWPA2")+1); 00092 break; 00093 default: 00094 strncpy(sec,"",strlen("")+1); 00095 break; 00096 } 00097 00098 if( !(_wizfi310.connect(ap_ssid, ap_pass, sec)) ) 00099 { 00100 return NSAPI_ERROR_NO_CONNECTION; 00101 } 00102 00103 if( !_wizfi310.getIPAddress() ) 00104 { 00105 return NSAPI_ERROR_DHCP_FAILURE; 00106 } 00107 00108 return NSAPI_ERROR_OK; 00109 } 00110 00111 00112 int WizFi310Interface::set_credentials(const char *ssid, const char *pass, nsapi_security_t security) 00113 { 00114 memset(ap_ssid, 0, sizeof(ap_ssid)); 00115 strncpy(ap_ssid, ssid, sizeof(ap_ssid)); 00116 00117 memset(ap_pass, 0, sizeof(ap_pass)); 00118 strncpy(ap_pass, pass, sizeof(ap_pass)); 00119 00120 ap_sec = security; 00121 return 0; 00122 } 00123 00124 00125 int WizFi310Interface::set_channel(uint8_t channel) 00126 { 00127 } 00128 00129 int WizFi310Interface::disconnect() 00130 { 00131 _wizfi310.setTimeout(WIZFI310_MISC_TIMEOUT); 00132 00133 if (!_wizfi310.disconnect()) 00134 { 00135 return NSAPI_ERROR_DEVICE_ERROR; 00136 } 00137 return NSAPI_ERROR_OK; 00138 } 00139 00140 const char *WizFi310Interface::get_ip_address() 00141 { 00142 return _wizfi310.getIPAddress(); 00143 } 00144 00145 const char *WizFi310Interface::get_mac_address() 00146 { 00147 return _wizfi310.getMACAddress(); 00148 } 00149 00150 const char *WizFi310Interface::get_gateway() 00151 { 00152 return _wizfi310.getGateway(); 00153 } 00154 00155 const char *WizFi310Interface::get_netmask() 00156 { 00157 return _wizfi310.getNetmask(); 00158 } 00159 00160 int8_t WizFi310Interface::get_rssi() 00161 { 00162 return _wizfi310.getRSSI(); 00163 } 00164 00165 int WizFi310Interface::scan(WiFiAccessPoint *res, unsigned count) 00166 { 00167 return _wizfi310.scan(res, count); 00168 } 00169 00170 nsapi_error_t WizFi310Interface::gethostbyname(const char *host, 00171 SocketAddress *address, nsapi_version_t version) 00172 { 00173 char host_ip[16]; 00174 00175 if( !_wizfi310.dns_lookup(host,host_ip) ){ 00176 return NSAPI_ERROR_DNS_FAILURE; 00177 } 00178 if ( !address->set_ip_address(host_ip) ){ 00179 return NSAPI_ERROR_DNS_FAILURE; 00180 } 00181 00182 return NSAPI_ERROR_OK; 00183 } 00184 00185 struct wizfi310_socket { 00186 int id; 00187 nsapi_protocol_t proto; 00188 bool connected; 00189 SocketAddress addr; 00190 }; 00191 00192 00193 int WizFi310Interface::socket_open(void **handle, nsapi_protocol_t proto) 00194 { 00195 // Look for an unused socket 00196 int id = -1; 00197 00198 for (int i=0; i<WIZFI310_SOCKET_COUNT; i++) { 00199 if (!_ids[i]){ 00200 id = i; 00201 //_ids[i] = true; 00202 break; 00203 } 00204 } 00205 00206 if (id == -1){ 00207 return NSAPI_ERROR_NO_SOCKET; 00208 } 00209 00210 struct wizfi310_socket *socket = new struct wizfi310_socket; 00211 if (!socket){ 00212 return NSAPI_ERROR_NO_SOCKET; 00213 } 00214 00215 socket->id = id; 00216 socket->proto = proto; 00217 socket->connected = false; 00218 *handle = socket; 00219 00220 return 0; 00221 } 00222 00223 int WizFi310Interface::socket_close(void *handle) 00224 { 00225 struct wizfi310_socket *socket = (struct wizfi310_socket *)handle; 00226 int err = 0; 00227 _wizfi310.setTimeout(WIZFI310_OPEN_CLOSE_TIMEOUT); 00228 00229 if (socket->connected && !_wizfi310.close(socket->id)) { 00230 err = NSAPI_ERROR_DEVICE_ERROR; 00231 } 00232 00233 socket->connected = false; 00234 _ids[socket->id] = false; 00235 delete socket; 00236 return err; 00237 } 00238 00239 int WizFi310Interface::socket_bind(void *handle, const SocketAddress &address) 00240 { 00241 return NSAPI_ERROR_UNSUPPORTED; 00242 } 00243 00244 int WizFi310Interface::socket_listen(void *handle, int backlog) 00245 { 00246 return NSAPI_ERROR_UNSUPPORTED; 00247 } 00248 00249 int WizFi310Interface::socket_connect(void *handle, const SocketAddress &addr) 00250 { 00251 struct wizfi310_socket *socket = (struct wizfi310_socket *)handle; 00252 _wizfi310.setTimeout(WIZFI310_OPEN_CLOSE_TIMEOUT); 00253 00254 const char *proto = (socket->proto == NSAPI_UDP) ? "UCN" : "TCN"; 00255 if (!_wizfi310.open(proto, socket->id, addr.get_ip_address(), addr.get_port())) { 00256 return NSAPI_ERROR_DEVICE_ERROR; 00257 } 00258 00259 socket->connected = true; 00260 _ids[socket->id] = true; 00261 return 0; 00262 } 00263 00264 int WizFi310Interface::socket_accept(void *server, void **socket, SocketAddress *addr) 00265 { 00266 return NSAPI_ERROR_UNSUPPORTED; 00267 } 00268 00269 int WizFi310Interface::socket_send(void *handle, const void *data, unsigned size) 00270 { 00271 struct wizfi310_socket *socket = (struct wizfi310_socket *)handle; 00272 _wizfi310.setTimeout(WIZFI310_SEND_TIMEOUT); 00273 00274 if (!_wizfi310.send(socket->id, data, size)) { 00275 return NSAPI_ERROR_DEVICE_ERROR; 00276 } 00277 00278 return size; 00279 } 00280 00281 int WizFi310Interface::socket_recv(void *handle, void *data, unsigned size) 00282 { 00283 struct wizfi310_socket *socket = (struct wizfi310_socket *)handle; 00284 _wizfi310.setTimeout(WIZFI310_RECV_TIMEOUT); 00285 00286 int32_t recv = _wizfi310.recv(socket->id, data, size); 00287 if (recv < 0) { 00288 return NSAPI_ERROR_WOULD_BLOCK; 00289 } 00290 00291 return recv; 00292 } 00293 00294 int WizFi310Interface::socket_sendto(void *handle, const SocketAddress &addr, const void *data, unsigned size) 00295 { 00296 struct wizfi310_socket *socket = (struct wizfi310_socket *)handle; 00297 00298 if (socket->connected && socket->addr != addr) { 00299 _wizfi310.setTimeout(WIZFI310_MISC_TIMEOUT); 00300 if (!_wizfi310.close(socket->id)) { 00301 return NSAPI_ERROR_DEVICE_ERROR; 00302 } 00303 socket->connected = false; 00304 } 00305 00306 if (!socket->connected) { 00307 int err = socket_connect(socket, addr); 00308 if (err < 0 ) { 00309 return err; 00310 } 00311 socket->addr = addr; 00312 } 00313 00314 return socket_send(socket, data, size); 00315 } 00316 00317 int WizFi310Interface::socket_recvfrom(void *handle, SocketAddress *addr, void *data, unsigned size) 00318 { 00319 struct wizfi310_socket *socket = (struct wizfi310_socket *)handle; 00320 int ret = socket_recv(socket, data, size); 00321 if (ret >= 0 && addr) { 00322 *addr = socket->addr; 00323 } 00324 00325 return ret; 00326 } 00327 00328 void WizFi310Interface::socket_attach(void *handle, void (*callback)(void *), void *data) 00329 { 00330 struct wizfi310_socket *socket = (struct wizfi310_socket *)handle; 00331 _cbs[socket->id].callback = callback; 00332 _cbs[socket->id].data = data; 00333 } 00334 00335 void WizFi310Interface::event() 00336 { 00337 for(int i=0; i<WIZFI310_SOCKET_COUNT; i++){ 00338 if (_cbs[i].callback) { 00339 _cbs[i].callback(_cbs[i].data); 00340 } 00341 } 00342 }
Generated on Tue Jul 12 2022 17:32:54 by
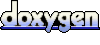