
USBAudio play by PWM.
Fork of USBAudio_HelloWorld by
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 // Hello World example for the USBAudio library 00002 00003 #include "mbed.h" 00004 #include "USBAudio.h" 00005 PwmOut led(p22); 00006 Serial pc(USBTX, USBRX); 00007 00008 // frequency: 48 kHz 00009 #define FREQ 48000 00010 00011 // 1 channel: mono 00012 #define NB_CHA 1 00013 00014 // length of an audio packet: each ms, we receive 48 * 16bits ->48 * 2 bytes. as there is one channel, the length will be 48 * 2 * 1 00015 #define AUDIO_LENGTH_PACKET FREQ/1000 * 2 * 1 00016 00017 // USBAudio 00018 USBAudio audio(FREQ, NB_CHA); 00019 int16_t buf[AUDIO_LENGTH_PACKET/2]; 00020 float bufo[2][AUDIO_LENGTH_PACKET/2]; 00021 bool swb=false,swb2=false; 00022 int pli=0; 00023 void Sample_timer_interrupt(void) 00024 { 00025 //static unsigned int i=0; 00026 00027 // send next analog sample out to D to A 00028 led = bufo[swb][pli]; 00029 // increment pointer and wrap around back to 0 at 128 00030 pli++; 00031 if(pli==(AUDIO_LENGTH_PACKET>>1))pli=0,swb=!swb; 00032 //if(i==0)audio.read((uint8_t *)buf); 00033 } 00034 00035 int main() { 00036 //int16_t buf[AUDIO_LENGTH_PACKET/2]; 00037 //led.pulsewidth(0.000000001); 00038 led.period(1.0/(96000.0*2));//1.0/(48000.0*16)); 00039 // set up a timer to be used for sample rate interrupts 00040 Ticker Sample_Period; 00041 Sample_Period.attach(&Sample_timer_interrupt, 1.0/(FREQ)); 00042 // audio.read((uint8_t *)buf); 00043 while (1) { 00044 // read an audio packet 00045 audio.read((uint8_t *)buf); 00046 float vol=audio.getVolume(); 00047 // print packet received 00048 //pc.printf("recv: "); 00049 //swb2=false; 00050 for(int i = 0; i < (AUDIO_LENGTH_PACKET>>1); i++) { 00051 bufo[!swb][i]=((float)buf[i]*vol/510.0)+0.5;} 00052 pli=0; 00053 //swb2=true; 00054 // led = (float)buf[i]/128.0; 00055 //pc.printf("%d ", buf[i]); 00056 // led=(float)buf[i]/128.0; 00057 // } 00058 // pc.printf("\r\n"); 00059 } 00060 00061 }
Generated on Tue Jul 19 2022 00:27:56 by
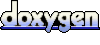