
Lab3 - Pressure, temperature, and humidity sensors displayed on a webpage.
Dependencies: EthernetNetIf NTPClient_NetServices GPS mbed HTTPServer SDFileSystem
PachubeClient.cpp
00001 #include "PachubeClient.h" 00002 00003 PachubeClient::PachubeClient(const string& apiKey) : _client(), _csvContent("text/csv") { 00004 _client.setRequestHeader("X-PachubeApiKey", apiKey); 00005 } 00006 00007 PachubeClient::~PachubeClient() { 00008 } 00009 00010 // put csv method to feed 00011 void PachubeClient::PutCsv(const string& environmentID, const string& data) { 00012 _csvContent.set(data); 00013 string uri = "http://api.pachube.com/v1/feeds/" + environmentID + ".csv?_method=put"; 00014 _result = _client.post(uri.c_str(), _csvContent, NULL); 00015 _response = _client.getHTTPResponseCode(); 00016 } 00017 00018 // put csv method to datastream 00019 void PachubeClient::PutCsv(const string& environmentID, const string& datastreamID, const string& data) { 00020 _csvContent.set(data); 00021 string uri = "http://api.pachube.com/v1/feeds/" + environmentID + "/datastreams/" + datastreamID + ".csv?_method=put"; 00022 _result = _client.post(uri.c_str(), _csvContent, NULL); 00023 _response = _client.getHTTPResponseCode(); 00024 } 00025 00026 // http result and response 00027 HTTPResult PachubeClient::Result() { 00028 return _result; 00029 } 00030 int PachubeClient::Response() { 00031 return _response; 00032 }
Generated on Tue Jul 19 2022 00:59:33 by
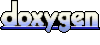