
This a great example of how Bluetooth (BT) reads incoming chars. This program constantly check if a char has been sent over BT and then turns on or off a digital output on the K64F board. We used MIT App Inventor to download an app to a android phone. This app would connect to our HC06 BT module on the K64F. The app would send chars when certain buttons were pressed. The K64F would then receive them and take appropriate action. Our digital output would send signal to relays, that's why you see variables called relay1,2,3... The relays then allowed power to got to a power strip.
main.cpp
00001 #include "mbed.h" 00002 00003 Serial bluetooth(PTC15, PTC14); // pins to connect on HC-06 00004 int mydata=0; 00005 00006 00007 DigitalOut led_r(LED1); // on board LED 00008 DigitalOut Relay1(PTC17); 00009 DigitalOut Relay2(PTB9); 00010 DigitalOut Relay3(PTA1); 00011 DigitalOut Relay4(PTB23); 00012 DigitalOut Relay5(PTA2); 00013 00014 int main() 00015 00016 { 00017 Relay1 = 0; 00018 Relay2 = 0; 00019 Relay3 = 0; 00020 Relay4 = 0; 00021 Relay5 = 0; 00022 led_r = 1; 00023 00024 bluetooth.baud(9600); 00025 bluetooth.printf("\nSTM32 bluetooth\n"); 00026 printf("\r\nready\r\n"); 00027 while(1) 00028 { 00029 00030 if(bluetooth.readable()) 00031 { 00032 mydata=bluetooth.getc(); 00033 bluetooth.putc(mydata); 00034 printf("mydata = %c\r\n",mydata); 00035 } // end if 00036 00037 switch(mydata) 00038 { 00039 case 'a': 00040 Relay1 = 0; 00041 break; 00042 case 'A': 00043 Relay1 = 1; 00044 break; 00045 case 'b': 00046 Relay2 = 0; 00047 break; 00048 case 'B': 00049 Relay2 = 1; 00050 break; 00051 case 'c': 00052 Relay3 = 0; 00053 break; 00054 case 'C': 00055 Relay3 = 1; 00056 break; 00057 case 'd': 00058 Relay4 = 0; 00059 break; 00060 case 'D': 00061 Relay4 = 1; 00062 break; 00063 case 'e': 00064 Relay5 = 0; 00065 break; 00066 case 'E': 00067 Relay5 = 1; 00068 break; 00069 default: 00070 led_r = 0; 00071 } // end switch 00072 00073 //wait(1.0f); 00074 //printf("one second has elapsed.\r\n"); 00075 00076 } // end while 00077 00078 } // end main
Generated on Sun Aug 28 2022 06:10:05 by
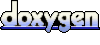