
FRDM-K64F, Avnet M14A2A, Grove Shield, to create smart home system. In use with AT&Ts M2x & Flow.
Dependencies: mbed FXOS8700CQ MODSERIAL
xadow_gps.h
00001 /* =================================================================== 00002 Copyright © 2016, AVNET Inc. 00003 00004 Licensed under the Apache License, Version 2.0 (the "License"); 00005 you may not use this file except in compliance with the License. 00006 You may obtain a copy of the License at 00007 00008 http://www.apache.org/licenses/LICENSE-2.0 00009 00010 Unless required by applicable law or agreed to in writing, 00011 software distributed under the License is distributed on an 00012 "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, 00013 either express or implied. See the License for the specific 00014 language governing permissions and limitations under the License. 00015 00016 ======================================================================== */ 00017 00018 #ifndef __XADOW_GPS_H_ 00019 #define __XADOW_GPS_H_ 00020 00021 /*! 00022 \def GPS_DEVICE_ADDR 00023 The I2C address GPS 00024 00025 \def GPS_SCAN_ID 00026 The id of scan data, the format is 0,0,0,Device address 00027 \def GPS_SCAN_SIZE 00028 The length of scan data 00029 00030 \def GPS_UTC_DATE_TIME_ID 00031 The id of utc date and time, the format is YMDHMS 00032 \def GPS_UTC_DATE_TIME_SIZE 00033 The length of utc date and time data 00034 00035 \def GPS_STATUS_ID 00036 The id of GPS status, the format is A/V 00037 \def GPS_STATUS_SIZE 00038 The length of GPS status data 00039 00040 \def GPS_LATITUDE_ID 00041 The id of latitude, the format is dd.dddddd 00042 \def GPS_LATITUDE_SIZE 00043 The length of latitude data 00044 00045 \def GPS_NS_ID 00046 The id of latitude direction, the format is N/S 00047 \def GPS_NS_SIZE 00048 The length of latitude direction data 00049 00050 \def GPS_LONGITUDE_ID 00051 The id of longitude, the format is ddd.dddddd 00052 \def GPS_LONGITUDE_SIZE 00053 The length of longitude data 00054 00055 \def GPS_EW_ID 00056 The id of longitude direction, the format is E/W 00057 \def GPS_EW_SIZE 00058 The length of longitude direction data 00059 00060 \def GPS_SPEED_ID 00061 The id of speed, the format is 000.0~999.9 Knots 00062 \def GPS_SPEED_SIZE 00063 The length of speed data 00064 00065 \def GPS_COURSE_ID 00066 The id of course, the format is 000.0~359.9 00067 \def GPS_COURSE_SIZE 00068 The length of course data 00069 00070 \def GPS_POSITION_FIX_ID 00071 The id of position fix status, the format is 0,1,2,6 00072 \def GPS_POSITION_FIX_SIZE 00073 The length of position fix status data 00074 00075 \def GPS_SATE_USED_ID 00076 The id of state used, the format is 00~12 00077 \def GPS_SATE_USED_SIZE 00078 The length of sate used data 00079 00080 \def GPS_ALTITUDE_ID 00081 The id of altitude, the format is -9999.9~99999.9 00082 \def GPS_ALTITUDE_SIZE 00083 The length of altitude data 00084 00085 \def GPS_MODE_ID 00086 The id of locate mode, the format is A/M 00087 \def GPS_MODE_SIZE 00088 The length of locate mode data 00089 00090 \def GPS_MODE2_ID 00091 The id of current status, the format is 1,2,3 00092 \def GPS_MODE2_SIZE 00093 The length of current status data 00094 00095 \def GPS_SATE_IN_VIEW_ID 00096 The id of sate in view 00097 \def GPS_SATE_IN_VIEW_SIZE 00098 The length of sate in view data 00099 */ 00100 00101 /* Data format: 00102 * ID(1 byte), Data length(1 byte), Data 1, Data 2, ... Data n (n bytes, n = data length) 00103 * For example, get the scan data. 00104 * First, Send GPS_SCAN_ID(1 byte) to device. 00105 * Second, Receive scan data(ID + Data length + GPS_SCAN_SIZE = 6 bytes). 00106 * Third, The scan data begin from the third data of received. 00107 * End 00108 */ 00109 00110 #define GPS_DEVICE_ID 0x05 00111 //#define GPS_DEVICE_ADDR 0x05 00112 #define GPS_DEVICE_ADDR 0x0A //For mbed, the address has to be << 1 00113 00114 #define GPS_SCAN_ID 0 // 4 bytes 00115 #define GPS_SCAN_SIZE 4 // 0,0,0,Device address 00116 00117 #define GPS_UTC_DATE_TIME_ID 1 // YMDHMS 00118 #define GPS_UTC_DATE_TIME_SIZE 6 // 6 bytes 00119 00120 #define GPS_STATUS_ID 2 // A/V 00121 #define GPS_STATUS_SIZE 1 // 1 byte 00122 00123 #define GPS_LATITUDE_ID 3 // dd.dddddd 00124 #define GPS_LATITUDE_SIZE 9 // 9 bytes 00125 00126 #define GPS_NS_ID 4 // N/S 00127 #define GPS_NS_SIZE 1 // 1 byte 00128 00129 #define GPS_LONGITUDE_ID 5 // ddd.dddddd 00130 #define GPS_LONGITUDE_SIZE 10 // 10 bytes 00131 00132 #define GPS_EW_ID 6 // E/W 00133 #define GPS_EW_SIZE 1 // 1 byte 00134 00135 #define GPS_SPEED_ID 7 // 000.0~999.9 Knots 00136 #define GPS_SPEED_SIZE 5 // 5 bytes 00137 00138 #define GPS_COURSE_ID 8 // 000.0~359.9 00139 #define GPS_COURSE_SIZE 5 // 5 bytes 00140 00141 #define GPS_POSITION_FIX_ID 9 // 0,1,2,6 00142 #define GPS_POSITION_FIX_SIZE 1 // 1 byte 00143 00144 #define GPS_SATE_USED_ID 10 // 00~12 00145 #define GPS_SATE_USED_SIZE 2 // 2 bytes 00146 00147 #define GPS_ALTITUDE_ID 11 // -9999.9~99999.9 00148 #define GPS_ALTITUDE_SIZE 7 // 7 bytes 00149 00150 #define GPS_MODE_ID 12 // A/M 00151 #define GPS_MODE_SIZE 1 // 1 byte 00152 00153 #define GPS_MODE2_ID 13 // 1,2,3 00154 #define GPS_MODE2_SIZE 1 // 1 byte 00155 00156 #define GPS_SATE_IN_VIEW_ID 14 // 0~12 00157 #define GPS_SATE_IN_VIEW_SIZE 1 // 1 byte 00158 00159 00160 /** 00161 * \brief Get the status of the device. 00162 * 00163 * \return Return TRUE or FALSE. TRUE is on line, FALSE is off line. 00164 * 00165 */ 00166 unsigned char gps_check_online(void); 00167 00168 /** 00169 * \brief Get the utc date and time. 00170 * 00171 * \return Return the pointer of utc date sand time, the format is YMDHMS. 00172 * 00173 */ 00174 unsigned char* gps_get_utc_date_time(void); 00175 00176 /** 00177 * \brief Get the status of GPS. 00178 * 00179 * \return Return A or V. A is orientation, V is navigation. 00180 * 00181 */ 00182 unsigned char gps_get_status(void); 00183 00184 /** 00185 * \brief Get the altitude. 00186 * 00187 * \return Return altitude data. The format is dd.dddddd. 00188 * 00189 */ 00190 float gps_get_latitude(void); 00191 00192 /** 00193 * \brief Get the lattitude direction. 00194 * 00195 * \return Return lattitude direction data. The format is N/S. N is north, S is south. 00196 * 00197 */ 00198 unsigned char gps_get_ns(void); 00199 00200 /** 00201 * \brief Get the longitude. 00202 * 00203 * \return Return longitude data. The format is ddd.dddddd. 00204 * 00205 */ 00206 float gps_get_longitude(void); 00207 00208 /** 00209 * \brief Get the longitude direction. 00210 * 00211 * \return Return longitude direction data. The format is E/W. E is east, W is west. 00212 * 00213 */ 00214 unsigned char gps_get_ew(void); 00215 00216 /** 00217 * \brief Get the speed. 00218 * 00219 * \return Return speed data. The format is 000.0~999.9 Knots. 00220 * 00221 */ 00222 float gps_get_speed(void); 00223 00224 /** 00225 * \brief Get the course. 00226 * 00227 * \return Return course data. The format is 000.0~359.9. 00228 * 00229 */ 00230 float gps_get_course(void); 00231 00232 /** 00233 * \brief Get the status of position fix. 00234 * 00235 * \return Return position fix data. The format is 0,1,2,6. 00236 * 00237 */ 00238 unsigned char gps_get_position_fix(void); 00239 00240 /** 00241 * \brief Get the number of state used¡£ 00242 * 00243 * \return Return number of state used. The format is 0-12. 00244 * 00245 */ 00246 unsigned char gps_get_sate_used(void); 00247 00248 /** 00249 * \brief Get the altitude¡£ the format is -9999.9~99999.9 00250 * 00251 * \return Return altitude data. The format is -9999.9~99999.9. 00252 * 00253 */ 00254 float gps_get_altitude(void); 00255 00256 /** 00257 * \brief Get the mode of location. 00258 * 00259 * \return Return mode of location. The format is A/M. A:automatic, M:manual. 00260 * 00261 */ 00262 unsigned char gps_get_mode(void); 00263 00264 /** 00265 * \brief Get the current status of GPS. 00266 * 00267 * \return Return current status. The format is 1,2,3. 1:null, 2:2D, 3:3D. 00268 * 00269 */ 00270 unsigned char gps_get_mode2(void); 00271 00272 /** 00273 * \brief Get the number of sate in view. 00274 * 00275 * \return Return the number of sate in view. 00276 * 00277 */ 00278 unsigned char gps_get_sate_in_veiw(void); 00279 00280 #endif
Generated on Wed Jul 13 2022 15:25:27 by
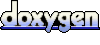