
FRDM-K64F, Avnet M14A2A, Grove Shield, to create smart home system. In use with AT&Ts M2x & Flow.
Dependencies: mbed FXOS8700CQ MODSERIAL
xadow_gps.cpp
00001 /* =================================================================== 00002 Copyright © 2016, AVNET Inc. 00003 00004 Licensed under the Apache License, Version 2.0 (the "License"); 00005 you may not use this file except in compliance with the License. 00006 You may obtain a copy of the License at 00007 00008 http://www.apache.org/licenses/LICENSE-2.0 00009 00010 Unless required by applicable law or agreed to in writing, 00011 software distributed under the License is distributed on an 00012 "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, 00013 either express or implied. See the License for the specific 00014 language governing permissions and limitations under the License. 00015 00016 ======================================================================== */ 00017 00018 #include "mbed.h" 00019 #include "xadow_gps.h" 00020 #include "hardware.h" 00021 00022 // Xadow code based on Eclipse test project at 00023 // https://github.com/WayenWeng/Xadow_GPS_v2_test/ 00024 00025 // These first 3 routines are to allow the mbed I2C to be used instead of what was in the Eclipse test code 00026 void dlc_i2c_configure(int slave_addr, int speed) 00027 { 00028 } //dlc_i2c_configure 00029 00030 unsigned char dlc_i2c_receive_byte(void) 00031 { 00032 char rxbuffer [1]; 00033 i2c.read(GPS_DEVICE_ADDR, rxbuffer, 1 ); 00034 return (unsigned char)rxbuffer[0]; 00035 } //dlc_i2c_receive_byte() 00036 00037 unsigned char dlc_i2c_send_byte(unsigned char ucData) 00038 { 00039 int status; 00040 char txbuffer [1]; 00041 txbuffer[0] = (char)ucData; 00042 status = i2c.write(GPS_DEVICE_ADDR, txbuffer, 1, false); //true: do not send stop 00043 return status; 00044 } //dlc_i2c_send_byte() 00045 00046 00047 unsigned char gps_check_online(void) 00048 { 00049 unsigned char data[GPS_SCAN_SIZE+2]; 00050 unsigned char i; 00051 00052 dlc_i2c_configure(GPS_DEVICE_ADDR, 100); 00053 00054 dlc_i2c_send_byte(GPS_SCAN_ID); 00055 00056 for(i=0;i<(GPS_SCAN_SIZE+2);i++) 00057 { 00058 data[i] = dlc_i2c_receive_byte(); 00059 } 00060 00061 if(data[5] == GPS_DEVICE_ID) 00062 return 1; 00063 else return 0; 00064 } 00065 00066 unsigned char gps_utc_date_time[GPS_UTC_DATE_TIME_SIZE] = {0}; 00067 00068 unsigned char* gps_get_utc_date_time(void) 00069 { 00070 unsigned char data[GPS_UTC_DATE_TIME_SIZE+2]; 00071 unsigned char i; 00072 00073 dlc_i2c_configure(GPS_DEVICE_ADDR, 100); 00074 00075 dlc_i2c_send_byte(GPS_UTC_DATE_TIME_ID); 00076 00077 for(i=0;i<(GPS_UTC_DATE_TIME_SIZE+2);i++) 00078 { 00079 data[i] = dlc_i2c_receive_byte(); 00080 } 00081 00082 for(i=0;i<GPS_UTC_DATE_TIME_SIZE;i++) 00083 gps_utc_date_time[i] = data[i+2]; 00084 00085 return gps_utc_date_time; 00086 } 00087 00088 unsigned char gps_get_status(void) 00089 { 00090 unsigned char data[GPS_STATUS_SIZE+2]; 00091 unsigned char i; 00092 00093 dlc_i2c_configure(GPS_DEVICE_ADDR, 100); 00094 00095 dlc_i2c_send_byte(GPS_STATUS_ID); 00096 for(i=0;i<(GPS_STATUS_SIZE+2);i++) 00097 { 00098 data[i] = dlc_i2c_receive_byte(); 00099 } 00100 00101 return data[2]; 00102 } 00103 00104 float gps_get_latitude(void) 00105 { 00106 char data[GPS_LATITUDE_SIZE+2]; 00107 unsigned char i; 00108 00109 dlc_i2c_configure(GPS_DEVICE_ADDR, 100); 00110 00111 dlc_i2c_send_byte(GPS_LATITUDE_ID); 00112 for(i=0;i<(GPS_LATITUDE_SIZE+2);i++) 00113 { 00114 data[i] = (char)dlc_i2c_receive_byte(); 00115 } 00116 00117 return atof(&data[2]); 00118 } 00119 00120 unsigned char gps_get_ns(void) 00121 { 00122 unsigned char data[GPS_NS_SIZE+2]; 00123 unsigned char i; 00124 00125 dlc_i2c_configure(GPS_DEVICE_ADDR, 100); 00126 00127 dlc_i2c_send_byte(GPS_NS_ID); 00128 for(i=0;i<(GPS_NS_SIZE+2);i++) 00129 { 00130 data[i] = dlc_i2c_receive_byte(); 00131 } 00132 00133 if(data[2] == 'N' || data[2] == 'S')return data[2]; 00134 else return data[2] = '-'; 00135 00136 } 00137 00138 float gps_get_longitude(void) 00139 { 00140 char data[GPS_LONGITUDE_SIZE+2]; 00141 unsigned char i; 00142 00143 dlc_i2c_configure(GPS_DEVICE_ADDR, 100); 00144 00145 dlc_i2c_send_byte(GPS_LONGITUDE_ID); 00146 for(i=0;i<(GPS_LONGITUDE_SIZE+2);i++) 00147 { 00148 data[i] = (char)dlc_i2c_receive_byte(); 00149 } 00150 00151 return atof(&data[2]); 00152 } 00153 00154 unsigned char gps_get_ew(void) 00155 { 00156 unsigned char data[GPS_EW_SIZE+2]; 00157 unsigned char i; 00158 00159 dlc_i2c_configure(GPS_DEVICE_ADDR, 100); 00160 00161 dlc_i2c_send_byte(GPS_EW_ID); 00162 for(i=0;i<(GPS_EW_SIZE+2);i++) 00163 { 00164 data[i] = dlc_i2c_receive_byte(); 00165 } 00166 00167 if(data[2] == 'E' || data[2] == 'W')return data[2]; 00168 else return data[2] = '-'; 00169 } 00170 00171 float gps_get_speed(void) 00172 { 00173 char data[GPS_SPEED_SIZE+2]; 00174 unsigned char i; 00175 00176 dlc_i2c_configure(GPS_DEVICE_ADDR, 100); 00177 00178 dlc_i2c_send_byte(GPS_SPEED_ID); 00179 for(i=0;i<(GPS_SPEED_SIZE+2);i++) 00180 { 00181 data[i] = (char)dlc_i2c_receive_byte(); 00182 } 00183 00184 return atof(&data[2]); 00185 } 00186 00187 float gps_get_course(void) 00188 { 00189 char data[GPS_COURSE_SIZE+2]; 00190 unsigned char i; 00191 00192 dlc_i2c_configure(GPS_DEVICE_ADDR, 100); 00193 00194 dlc_i2c_send_byte(GPS_COURSE_ID); 00195 for(i=0;i<(GPS_COURSE_SIZE+2);i++) 00196 { 00197 data[i] = (char)dlc_i2c_receive_byte(); 00198 } 00199 00200 return atof(&data[2]); 00201 } 00202 00203 unsigned char gps_get_position_fix(void) 00204 { 00205 unsigned char data[GPS_POSITION_FIX_SIZE+2]; 00206 unsigned char i; 00207 00208 dlc_i2c_configure(GPS_DEVICE_ADDR, 100); 00209 00210 dlc_i2c_send_byte(GPS_POSITION_FIX_ID); 00211 for(i=0;i<(GPS_POSITION_FIX_SIZE+2);i++) 00212 { 00213 data[i] = dlc_i2c_receive_byte(); 00214 } 00215 00216 return data[2]; 00217 } 00218 00219 unsigned char gps_get_sate_used(void) 00220 { 00221 unsigned char data[GPS_SATE_USED_SIZE+2]; 00222 unsigned char i; 00223 unsigned char value; 00224 00225 dlc_i2c_configure(GPS_DEVICE_ADDR, 100); 00226 00227 dlc_i2c_send_byte(GPS_SATE_USED_ID); 00228 for(i=0;i<(GPS_SATE_USED_SIZE+2);i++) 00229 { 00230 data[i] = dlc_i2c_receive_byte(); 00231 } 00232 00233 if(data[3] >= '0' && data[3] <= '9')value = (data[3] - '0') * 10; 00234 else value = 0; 00235 if(data[2] >= '0' && data[2] <= '9')value += (data[2] - '0'); 00236 else value += 0; 00237 00238 return value; 00239 } 00240 00241 float gps_get_altitude(void) 00242 { 00243 char data[GPS_ALTITUDE_SIZE+2]; 00244 unsigned char i; 00245 00246 dlc_i2c_configure(GPS_DEVICE_ADDR, 100); 00247 00248 dlc_i2c_send_byte(GPS_ALTITUDE_ID); 00249 for(i=0;i<(GPS_ALTITUDE_SIZE+2);i++) 00250 { 00251 data[i] = (char)dlc_i2c_receive_byte(); 00252 } 00253 00254 return atof(&data[2]); 00255 } 00256 00257 unsigned char gps_get_mode(void) 00258 { 00259 unsigned char data[GPS_MODE_SIZE+2]; 00260 unsigned char i; 00261 00262 dlc_i2c_configure(GPS_DEVICE_ADDR, 100); 00263 00264 dlc_i2c_send_byte(GPS_MODE_ID); 00265 for(i=0;i<(GPS_MODE_SIZE+2);i++) 00266 { 00267 data[i] = dlc_i2c_receive_byte(); 00268 } 00269 00270 return data[2]; 00271 } 00272 00273 unsigned char gps_get_mode2(void) 00274 { 00275 unsigned char data[GPS_MODE2_SIZE+2]; 00276 unsigned char i; 00277 00278 dlc_i2c_configure(GPS_DEVICE_ADDR, 100); 00279 00280 dlc_i2c_send_byte(GPS_MODE2_ID); 00281 for(i=0;i<(GPS_MODE2_SIZE+2);i++) 00282 { 00283 data[i] = dlc_i2c_receive_byte(); 00284 } 00285 00286 return data[2]; 00287 } 00288 00289 unsigned char gps_get_sate_in_veiw(void) 00290 { 00291 unsigned char data[GPS_SATE_IN_VIEW_SIZE+2]; 00292 unsigned char i; 00293 00294 dlc_i2c_configure(GPS_DEVICE_ADDR, 100); 00295 00296 dlc_i2c_send_byte(GPS_SATE_IN_VIEW_ID); 00297 for(i=0;i<(GPS_SATE_IN_VIEW_SIZE+2);i++) 00298 { 00299 data[i] = dlc_i2c_receive_byte(); 00300 } 00301 00302 return data[2]; 00303 }
Generated on Wed Jul 13 2022 15:25:27 by
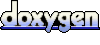