Working towards recieving twists
Fork of ros_lib_kinetic by
Embed:
(wiki syntax)
Show/hide line numbers
SmachContainerStructure.h
00001 #ifndef _ROS_smach_msgs_SmachContainerStructure_h 00002 #define _ROS_smach_msgs_SmachContainerStructure_h 00003 00004 #include <stdint.h> 00005 #include <string.h> 00006 #include <stdlib.h> 00007 #include "ros/msg.h" 00008 #include "std_msgs/Header.h" 00009 00010 namespace smach_msgs 00011 { 00012 00013 class SmachContainerStructure : public ros::Msg 00014 { 00015 public: 00016 typedef std_msgs::Header _header_type; 00017 _header_type header; 00018 typedef const char* _path_type; 00019 _path_type path; 00020 uint32_t children_length; 00021 typedef char* _children_type; 00022 _children_type st_children; 00023 _children_type * children; 00024 uint32_t internal_outcomes_length; 00025 typedef char* _internal_outcomes_type; 00026 _internal_outcomes_type st_internal_outcomes; 00027 _internal_outcomes_type * internal_outcomes; 00028 uint32_t outcomes_from_length; 00029 typedef char* _outcomes_from_type; 00030 _outcomes_from_type st_outcomes_from; 00031 _outcomes_from_type * outcomes_from; 00032 uint32_t outcomes_to_length; 00033 typedef char* _outcomes_to_type; 00034 _outcomes_to_type st_outcomes_to; 00035 _outcomes_to_type * outcomes_to; 00036 uint32_t container_outcomes_length; 00037 typedef char* _container_outcomes_type; 00038 _container_outcomes_type st_container_outcomes; 00039 _container_outcomes_type * container_outcomes; 00040 00041 SmachContainerStructure(): 00042 header(), 00043 path(""), 00044 children_length(0), children(NULL), 00045 internal_outcomes_length(0), internal_outcomes(NULL), 00046 outcomes_from_length(0), outcomes_from(NULL), 00047 outcomes_to_length(0), outcomes_to(NULL), 00048 container_outcomes_length(0), container_outcomes(NULL) 00049 { 00050 } 00051 00052 virtual int serialize(unsigned char *outbuffer) const 00053 { 00054 int offset = 0; 00055 offset += this->header.serialize(outbuffer + offset); 00056 uint32_t length_path = strlen(this->path); 00057 varToArr(outbuffer + offset, length_path); 00058 offset += 4; 00059 memcpy(outbuffer + offset, this->path, length_path); 00060 offset += length_path; 00061 *(outbuffer + offset + 0) = (this->children_length >> (8 * 0)) & 0xFF; 00062 *(outbuffer + offset + 1) = (this->children_length >> (8 * 1)) & 0xFF; 00063 *(outbuffer + offset + 2) = (this->children_length >> (8 * 2)) & 0xFF; 00064 *(outbuffer + offset + 3) = (this->children_length >> (8 * 3)) & 0xFF; 00065 offset += sizeof(this->children_length); 00066 for( uint32_t i = 0; i < children_length; i++){ 00067 uint32_t length_childreni = strlen(this->children[i]); 00068 varToArr(outbuffer + offset, length_childreni); 00069 offset += 4; 00070 memcpy(outbuffer + offset, this->children[i], length_childreni); 00071 offset += length_childreni; 00072 } 00073 *(outbuffer + offset + 0) = (this->internal_outcomes_length >> (8 * 0)) & 0xFF; 00074 *(outbuffer + offset + 1) = (this->internal_outcomes_length >> (8 * 1)) & 0xFF; 00075 *(outbuffer + offset + 2) = (this->internal_outcomes_length >> (8 * 2)) & 0xFF; 00076 *(outbuffer + offset + 3) = (this->internal_outcomes_length >> (8 * 3)) & 0xFF; 00077 offset += sizeof(this->internal_outcomes_length); 00078 for( uint32_t i = 0; i < internal_outcomes_length; i++){ 00079 uint32_t length_internal_outcomesi = strlen(this->internal_outcomes[i]); 00080 varToArr(outbuffer + offset, length_internal_outcomesi); 00081 offset += 4; 00082 memcpy(outbuffer + offset, this->internal_outcomes[i], length_internal_outcomesi); 00083 offset += length_internal_outcomesi; 00084 } 00085 *(outbuffer + offset + 0) = (this->outcomes_from_length >> (8 * 0)) & 0xFF; 00086 *(outbuffer + offset + 1) = (this->outcomes_from_length >> (8 * 1)) & 0xFF; 00087 *(outbuffer + offset + 2) = (this->outcomes_from_length >> (8 * 2)) & 0xFF; 00088 *(outbuffer + offset + 3) = (this->outcomes_from_length >> (8 * 3)) & 0xFF; 00089 offset += sizeof(this->outcomes_from_length); 00090 for( uint32_t i = 0; i < outcomes_from_length; i++){ 00091 uint32_t length_outcomes_fromi = strlen(this->outcomes_from[i]); 00092 varToArr(outbuffer + offset, length_outcomes_fromi); 00093 offset += 4; 00094 memcpy(outbuffer + offset, this->outcomes_from[i], length_outcomes_fromi); 00095 offset += length_outcomes_fromi; 00096 } 00097 *(outbuffer + offset + 0) = (this->outcomes_to_length >> (8 * 0)) & 0xFF; 00098 *(outbuffer + offset + 1) = (this->outcomes_to_length >> (8 * 1)) & 0xFF; 00099 *(outbuffer + offset + 2) = (this->outcomes_to_length >> (8 * 2)) & 0xFF; 00100 *(outbuffer + offset + 3) = (this->outcomes_to_length >> (8 * 3)) & 0xFF; 00101 offset += sizeof(this->outcomes_to_length); 00102 for( uint32_t i = 0; i < outcomes_to_length; i++){ 00103 uint32_t length_outcomes_toi = strlen(this->outcomes_to[i]); 00104 varToArr(outbuffer + offset, length_outcomes_toi); 00105 offset += 4; 00106 memcpy(outbuffer + offset, this->outcomes_to[i], length_outcomes_toi); 00107 offset += length_outcomes_toi; 00108 } 00109 *(outbuffer + offset + 0) = (this->container_outcomes_length >> (8 * 0)) & 0xFF; 00110 *(outbuffer + offset + 1) = (this->container_outcomes_length >> (8 * 1)) & 0xFF; 00111 *(outbuffer + offset + 2) = (this->container_outcomes_length >> (8 * 2)) & 0xFF; 00112 *(outbuffer + offset + 3) = (this->container_outcomes_length >> (8 * 3)) & 0xFF; 00113 offset += sizeof(this->container_outcomes_length); 00114 for( uint32_t i = 0; i < container_outcomes_length; i++){ 00115 uint32_t length_container_outcomesi = strlen(this->container_outcomes[i]); 00116 varToArr(outbuffer + offset, length_container_outcomesi); 00117 offset += 4; 00118 memcpy(outbuffer + offset, this->container_outcomes[i], length_container_outcomesi); 00119 offset += length_container_outcomesi; 00120 } 00121 return offset; 00122 } 00123 00124 virtual int deserialize(unsigned char *inbuffer) 00125 { 00126 int offset = 0; 00127 offset += this->header.deserialize(inbuffer + offset); 00128 uint32_t length_path; 00129 arrToVar(length_path, (inbuffer + offset)); 00130 offset += 4; 00131 for(unsigned int k= offset; k< offset+length_path; ++k){ 00132 inbuffer[k-1]=inbuffer[k]; 00133 } 00134 inbuffer[offset+length_path-1]=0; 00135 this->path = (char *)(inbuffer + offset-1); 00136 offset += length_path; 00137 uint32_t children_lengthT = ((uint32_t) (*(inbuffer + offset))); 00138 children_lengthT |= ((uint32_t) (*(inbuffer + offset + 1))) << (8 * 1); 00139 children_lengthT |= ((uint32_t) (*(inbuffer + offset + 2))) << (8 * 2); 00140 children_lengthT |= ((uint32_t) (*(inbuffer + offset + 3))) << (8 * 3); 00141 offset += sizeof(this->children_length); 00142 if(children_lengthT > children_length) 00143 this->children = (char**)realloc(this->children, children_lengthT * sizeof(char*)); 00144 children_length = children_lengthT; 00145 for( uint32_t i = 0; i < children_length; i++){ 00146 uint32_t length_st_children; 00147 arrToVar(length_st_children, (inbuffer + offset)); 00148 offset += 4; 00149 for(unsigned int k= offset; k< offset+length_st_children; ++k){ 00150 inbuffer[k-1]=inbuffer[k]; 00151 } 00152 inbuffer[offset+length_st_children-1]=0; 00153 this->st_children = (char *)(inbuffer + offset-1); 00154 offset += length_st_children; 00155 memcpy( &(this->children[i]), &(this->st_children), sizeof(char*)); 00156 } 00157 uint32_t internal_outcomes_lengthT = ((uint32_t) (*(inbuffer + offset))); 00158 internal_outcomes_lengthT |= ((uint32_t) (*(inbuffer + offset + 1))) << (8 * 1); 00159 internal_outcomes_lengthT |= ((uint32_t) (*(inbuffer + offset + 2))) << (8 * 2); 00160 internal_outcomes_lengthT |= ((uint32_t) (*(inbuffer + offset + 3))) << (8 * 3); 00161 offset += sizeof(this->internal_outcomes_length); 00162 if(internal_outcomes_lengthT > internal_outcomes_length) 00163 this->internal_outcomes = (char**)realloc(this->internal_outcomes, internal_outcomes_lengthT * sizeof(char*)); 00164 internal_outcomes_length = internal_outcomes_lengthT; 00165 for( uint32_t i = 0; i < internal_outcomes_length; i++){ 00166 uint32_t length_st_internal_outcomes; 00167 arrToVar(length_st_internal_outcomes, (inbuffer + offset)); 00168 offset += 4; 00169 for(unsigned int k= offset; k< offset+length_st_internal_outcomes; ++k){ 00170 inbuffer[k-1]=inbuffer[k]; 00171 } 00172 inbuffer[offset+length_st_internal_outcomes-1]=0; 00173 this->st_internal_outcomes = (char *)(inbuffer + offset-1); 00174 offset += length_st_internal_outcomes; 00175 memcpy( &(this->internal_outcomes[i]), &(this->st_internal_outcomes), sizeof(char*)); 00176 } 00177 uint32_t outcomes_from_lengthT = ((uint32_t) (*(inbuffer + offset))); 00178 outcomes_from_lengthT |= ((uint32_t) (*(inbuffer + offset + 1))) << (8 * 1); 00179 outcomes_from_lengthT |= ((uint32_t) (*(inbuffer + offset + 2))) << (8 * 2); 00180 outcomes_from_lengthT |= ((uint32_t) (*(inbuffer + offset + 3))) << (8 * 3); 00181 offset += sizeof(this->outcomes_from_length); 00182 if(outcomes_from_lengthT > outcomes_from_length) 00183 this->outcomes_from = (char**)realloc(this->outcomes_from, outcomes_from_lengthT * sizeof(char*)); 00184 outcomes_from_length = outcomes_from_lengthT; 00185 for( uint32_t i = 0; i < outcomes_from_length; i++){ 00186 uint32_t length_st_outcomes_from; 00187 arrToVar(length_st_outcomes_from, (inbuffer + offset)); 00188 offset += 4; 00189 for(unsigned int k= offset; k< offset+length_st_outcomes_from; ++k){ 00190 inbuffer[k-1]=inbuffer[k]; 00191 } 00192 inbuffer[offset+length_st_outcomes_from-1]=0; 00193 this->st_outcomes_from = (char *)(inbuffer + offset-1); 00194 offset += length_st_outcomes_from; 00195 memcpy( &(this->outcomes_from[i]), &(this->st_outcomes_from), sizeof(char*)); 00196 } 00197 uint32_t outcomes_to_lengthT = ((uint32_t) (*(inbuffer + offset))); 00198 outcomes_to_lengthT |= ((uint32_t) (*(inbuffer + offset + 1))) << (8 * 1); 00199 outcomes_to_lengthT |= ((uint32_t) (*(inbuffer + offset + 2))) << (8 * 2); 00200 outcomes_to_lengthT |= ((uint32_t) (*(inbuffer + offset + 3))) << (8 * 3); 00201 offset += sizeof(this->outcomes_to_length); 00202 if(outcomes_to_lengthT > outcomes_to_length) 00203 this->outcomes_to = (char**)realloc(this->outcomes_to, outcomes_to_lengthT * sizeof(char*)); 00204 outcomes_to_length = outcomes_to_lengthT; 00205 for( uint32_t i = 0; i < outcomes_to_length; i++){ 00206 uint32_t length_st_outcomes_to; 00207 arrToVar(length_st_outcomes_to, (inbuffer + offset)); 00208 offset += 4; 00209 for(unsigned int k= offset; k< offset+length_st_outcomes_to; ++k){ 00210 inbuffer[k-1]=inbuffer[k]; 00211 } 00212 inbuffer[offset+length_st_outcomes_to-1]=0; 00213 this->st_outcomes_to = (char *)(inbuffer + offset-1); 00214 offset += length_st_outcomes_to; 00215 memcpy( &(this->outcomes_to[i]), &(this->st_outcomes_to), sizeof(char*)); 00216 } 00217 uint32_t container_outcomes_lengthT = ((uint32_t) (*(inbuffer + offset))); 00218 container_outcomes_lengthT |= ((uint32_t) (*(inbuffer + offset + 1))) << (8 * 1); 00219 container_outcomes_lengthT |= ((uint32_t) (*(inbuffer + offset + 2))) << (8 * 2); 00220 container_outcomes_lengthT |= ((uint32_t) (*(inbuffer + offset + 3))) << (8 * 3); 00221 offset += sizeof(this->container_outcomes_length); 00222 if(container_outcomes_lengthT > container_outcomes_length) 00223 this->container_outcomes = (char**)realloc(this->container_outcomes, container_outcomes_lengthT * sizeof(char*)); 00224 container_outcomes_length = container_outcomes_lengthT; 00225 for( uint32_t i = 0; i < container_outcomes_length; i++){ 00226 uint32_t length_st_container_outcomes; 00227 arrToVar(length_st_container_outcomes, (inbuffer + offset)); 00228 offset += 4; 00229 for(unsigned int k= offset; k< offset+length_st_container_outcomes; ++k){ 00230 inbuffer[k-1]=inbuffer[k]; 00231 } 00232 inbuffer[offset+length_st_container_outcomes-1]=0; 00233 this->st_container_outcomes = (char *)(inbuffer + offset-1); 00234 offset += length_st_container_outcomes; 00235 memcpy( &(this->container_outcomes[i]), &(this->st_container_outcomes), sizeof(char*)); 00236 } 00237 return offset; 00238 } 00239 00240 const char * getType(){ return "smach_msgs/SmachContainerStructure"; }; 00241 const char * getMD5(){ return "3d3d1e0d0f99779ee9e58101a5dcf7ea"; }; 00242 00243 }; 00244 00245 } 00246 #endif
Generated on Tue Jul 12 2022 21:32:17 by
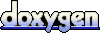