Working towards recieving twists
Fork of ros_lib_kinetic by
Embed:
(wiki syntax)
Show/hide line numbers
SetModelConfiguration.h
00001 #ifndef _ROS_SERVICE_SetModelConfiguration_h 00002 #define _ROS_SERVICE_SetModelConfiguration_h 00003 #include <stdint.h> 00004 #include <string.h> 00005 #include <stdlib.h> 00006 #include "ros/msg.h" 00007 00008 namespace gazebo_msgs 00009 { 00010 00011 static const char SETMODELCONFIGURATION[] = "gazebo_msgs/SetModelConfiguration"; 00012 00013 class SetModelConfigurationRequest : public ros::Msg 00014 { 00015 public: 00016 typedef const char* _model_name_type; 00017 _model_name_type model_name; 00018 typedef const char* _urdf_param_name_type; 00019 _urdf_param_name_type urdf_param_name; 00020 uint32_t joint_names_length; 00021 typedef char* _joint_names_type; 00022 _joint_names_type st_joint_names; 00023 _joint_names_type * joint_names; 00024 uint32_t joint_positions_length; 00025 typedef double _joint_positions_type; 00026 _joint_positions_type st_joint_positions; 00027 _joint_positions_type * joint_positions; 00028 00029 SetModelConfigurationRequest(): 00030 model_name(""), 00031 urdf_param_name(""), 00032 joint_names_length(0), joint_names(NULL), 00033 joint_positions_length(0), joint_positions(NULL) 00034 { 00035 } 00036 00037 virtual int serialize(unsigned char *outbuffer) const 00038 { 00039 int offset = 0; 00040 uint32_t length_model_name = strlen(this->model_name); 00041 varToArr(outbuffer + offset, length_model_name); 00042 offset += 4; 00043 memcpy(outbuffer + offset, this->model_name, length_model_name); 00044 offset += length_model_name; 00045 uint32_t length_urdf_param_name = strlen(this->urdf_param_name); 00046 varToArr(outbuffer + offset, length_urdf_param_name); 00047 offset += 4; 00048 memcpy(outbuffer + offset, this->urdf_param_name, length_urdf_param_name); 00049 offset += length_urdf_param_name; 00050 *(outbuffer + offset + 0) = (this->joint_names_length >> (8 * 0)) & 0xFF; 00051 *(outbuffer + offset + 1) = (this->joint_names_length >> (8 * 1)) & 0xFF; 00052 *(outbuffer + offset + 2) = (this->joint_names_length >> (8 * 2)) & 0xFF; 00053 *(outbuffer + offset + 3) = (this->joint_names_length >> (8 * 3)) & 0xFF; 00054 offset += sizeof(this->joint_names_length); 00055 for( uint32_t i = 0; i < joint_names_length; i++){ 00056 uint32_t length_joint_namesi = strlen(this->joint_names[i]); 00057 varToArr(outbuffer + offset, length_joint_namesi); 00058 offset += 4; 00059 memcpy(outbuffer + offset, this->joint_names[i], length_joint_namesi); 00060 offset += length_joint_namesi; 00061 } 00062 *(outbuffer + offset + 0) = (this->joint_positions_length >> (8 * 0)) & 0xFF; 00063 *(outbuffer + offset + 1) = (this->joint_positions_length >> (8 * 1)) & 0xFF; 00064 *(outbuffer + offset + 2) = (this->joint_positions_length >> (8 * 2)) & 0xFF; 00065 *(outbuffer + offset + 3) = (this->joint_positions_length >> (8 * 3)) & 0xFF; 00066 offset += sizeof(this->joint_positions_length); 00067 for( uint32_t i = 0; i < joint_positions_length; i++){ 00068 union { 00069 double real; 00070 uint64_t base; 00071 } u_joint_positionsi; 00072 u_joint_positionsi.real = this->joint_positions[i]; 00073 *(outbuffer + offset + 0) = (u_joint_positionsi.base >> (8 * 0)) & 0xFF; 00074 *(outbuffer + offset + 1) = (u_joint_positionsi.base >> (8 * 1)) & 0xFF; 00075 *(outbuffer + offset + 2) = (u_joint_positionsi.base >> (8 * 2)) & 0xFF; 00076 *(outbuffer + offset + 3) = (u_joint_positionsi.base >> (8 * 3)) & 0xFF; 00077 *(outbuffer + offset + 4) = (u_joint_positionsi.base >> (8 * 4)) & 0xFF; 00078 *(outbuffer + offset + 5) = (u_joint_positionsi.base >> (8 * 5)) & 0xFF; 00079 *(outbuffer + offset + 6) = (u_joint_positionsi.base >> (8 * 6)) & 0xFF; 00080 *(outbuffer + offset + 7) = (u_joint_positionsi.base >> (8 * 7)) & 0xFF; 00081 offset += sizeof(this->joint_positions[i]); 00082 } 00083 return offset; 00084 } 00085 00086 virtual int deserialize(unsigned char *inbuffer) 00087 { 00088 int offset = 0; 00089 uint32_t length_model_name; 00090 arrToVar(length_model_name, (inbuffer + offset)); 00091 offset += 4; 00092 for(unsigned int k= offset; k< offset+length_model_name; ++k){ 00093 inbuffer[k-1]=inbuffer[k]; 00094 } 00095 inbuffer[offset+length_model_name-1]=0; 00096 this->model_name = (char *)(inbuffer + offset-1); 00097 offset += length_model_name; 00098 uint32_t length_urdf_param_name; 00099 arrToVar(length_urdf_param_name, (inbuffer + offset)); 00100 offset += 4; 00101 for(unsigned int k= offset; k< offset+length_urdf_param_name; ++k){ 00102 inbuffer[k-1]=inbuffer[k]; 00103 } 00104 inbuffer[offset+length_urdf_param_name-1]=0; 00105 this->urdf_param_name = (char *)(inbuffer + offset-1); 00106 offset += length_urdf_param_name; 00107 uint32_t joint_names_lengthT = ((uint32_t) (*(inbuffer + offset))); 00108 joint_names_lengthT |= ((uint32_t) (*(inbuffer + offset + 1))) << (8 * 1); 00109 joint_names_lengthT |= ((uint32_t) (*(inbuffer + offset + 2))) << (8 * 2); 00110 joint_names_lengthT |= ((uint32_t) (*(inbuffer + offset + 3))) << (8 * 3); 00111 offset += sizeof(this->joint_names_length); 00112 if(joint_names_lengthT > joint_names_length) 00113 this->joint_names = (char**)realloc(this->joint_names, joint_names_lengthT * sizeof(char*)); 00114 joint_names_length = joint_names_lengthT; 00115 for( uint32_t i = 0; i < joint_names_length; i++){ 00116 uint32_t length_st_joint_names; 00117 arrToVar(length_st_joint_names, (inbuffer + offset)); 00118 offset += 4; 00119 for(unsigned int k= offset; k< offset+length_st_joint_names; ++k){ 00120 inbuffer[k-1]=inbuffer[k]; 00121 } 00122 inbuffer[offset+length_st_joint_names-1]=0; 00123 this->st_joint_names = (char *)(inbuffer + offset-1); 00124 offset += length_st_joint_names; 00125 memcpy( &(this->joint_names[i]), &(this->st_joint_names), sizeof(char*)); 00126 } 00127 uint32_t joint_positions_lengthT = ((uint32_t) (*(inbuffer + offset))); 00128 joint_positions_lengthT |= ((uint32_t) (*(inbuffer + offset + 1))) << (8 * 1); 00129 joint_positions_lengthT |= ((uint32_t) (*(inbuffer + offset + 2))) << (8 * 2); 00130 joint_positions_lengthT |= ((uint32_t) (*(inbuffer + offset + 3))) << (8 * 3); 00131 offset += sizeof(this->joint_positions_length); 00132 if(joint_positions_lengthT > joint_positions_length) 00133 this->joint_positions = (double*)realloc(this->joint_positions, joint_positions_lengthT * sizeof(double)); 00134 joint_positions_length = joint_positions_lengthT; 00135 for( uint32_t i = 0; i < joint_positions_length; i++){ 00136 union { 00137 double real; 00138 uint64_t base; 00139 } u_st_joint_positions; 00140 u_st_joint_positions.base = 0; 00141 u_st_joint_positions.base |= ((uint64_t) (*(inbuffer + offset + 0))) << (8 * 0); 00142 u_st_joint_positions.base |= ((uint64_t) (*(inbuffer + offset + 1))) << (8 * 1); 00143 u_st_joint_positions.base |= ((uint64_t) (*(inbuffer + offset + 2))) << (8 * 2); 00144 u_st_joint_positions.base |= ((uint64_t) (*(inbuffer + offset + 3))) << (8 * 3); 00145 u_st_joint_positions.base |= ((uint64_t) (*(inbuffer + offset + 4))) << (8 * 4); 00146 u_st_joint_positions.base |= ((uint64_t) (*(inbuffer + offset + 5))) << (8 * 5); 00147 u_st_joint_positions.base |= ((uint64_t) (*(inbuffer + offset + 6))) << (8 * 6); 00148 u_st_joint_positions.base |= ((uint64_t) (*(inbuffer + offset + 7))) << (8 * 7); 00149 this->st_joint_positions = u_st_joint_positions.real; 00150 offset += sizeof(this->st_joint_positions); 00151 memcpy( &(this->joint_positions[i]), &(this->st_joint_positions), sizeof(double)); 00152 } 00153 return offset; 00154 } 00155 00156 const char * getType(){ return SETMODELCONFIGURATION; }; 00157 const char * getMD5(){ return "160eae60f51fabff255480c70afa289f"; }; 00158 00159 }; 00160 00161 class SetModelConfigurationResponse : public ros::Msg 00162 { 00163 public: 00164 typedef bool _success_type; 00165 _success_type success; 00166 typedef const char* _status_message_type; 00167 _status_message_type status_message; 00168 00169 SetModelConfigurationResponse(): 00170 success(0), 00171 status_message("") 00172 { 00173 } 00174 00175 virtual int serialize(unsigned char *outbuffer) const 00176 { 00177 int offset = 0; 00178 union { 00179 bool real; 00180 uint8_t base; 00181 } u_success; 00182 u_success.real = this->success; 00183 *(outbuffer + offset + 0) = (u_success.base >> (8 * 0)) & 0xFF; 00184 offset += sizeof(this->success); 00185 uint32_t length_status_message = strlen(this->status_message); 00186 varToArr(outbuffer + offset, length_status_message); 00187 offset += 4; 00188 memcpy(outbuffer + offset, this->status_message, length_status_message); 00189 offset += length_status_message; 00190 return offset; 00191 } 00192 00193 virtual int deserialize(unsigned char *inbuffer) 00194 { 00195 int offset = 0; 00196 union { 00197 bool real; 00198 uint8_t base; 00199 } u_success; 00200 u_success.base = 0; 00201 u_success.base |= ((uint8_t) (*(inbuffer + offset + 0))) << (8 * 0); 00202 this->success = u_success.real; 00203 offset += sizeof(this->success); 00204 uint32_t length_status_message; 00205 arrToVar(length_status_message, (inbuffer + offset)); 00206 offset += 4; 00207 for(unsigned int k= offset; k< offset+length_status_message; ++k){ 00208 inbuffer[k-1]=inbuffer[k]; 00209 } 00210 inbuffer[offset+length_status_message-1]=0; 00211 this->status_message = (char *)(inbuffer + offset-1); 00212 offset += length_status_message; 00213 return offset; 00214 } 00215 00216 const char * getType(){ return SETMODELCONFIGURATION; }; 00217 const char * getMD5(){ return "2ec6f3eff0161f4257b808b12bc830c2"; }; 00218 00219 }; 00220 00221 class SetModelConfiguration { 00222 public: 00223 typedef SetModelConfigurationRequest Request; 00224 typedef SetModelConfigurationResponse Response; 00225 }; 00226 00227 } 00228 #endif
Generated on Tue Jul 12 2022 21:32:17 by
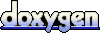