Working towards recieving twists
Fork of ros_lib_kinetic by
Embed:
(wiki syntax)
Show/hide line numbers
RegionOfInterest.h
00001 #ifndef _ROS_sensor_msgs_RegionOfInterest_h 00002 #define _ROS_sensor_msgs_RegionOfInterest_h 00003 00004 #include <stdint.h> 00005 #include <string.h> 00006 #include <stdlib.h> 00007 #include "ros/msg.h" 00008 00009 namespace sensor_msgs 00010 { 00011 00012 class RegionOfInterest : public ros::Msg 00013 { 00014 public: 00015 typedef uint32_t _x_offset_type; 00016 _x_offset_type x_offset; 00017 typedef uint32_t _y_offset_type; 00018 _y_offset_type y_offset; 00019 typedef uint32_t _height_type; 00020 _height_type height; 00021 typedef uint32_t _width_type; 00022 _width_type width; 00023 typedef bool _do_rectify_type; 00024 _do_rectify_type do_rectify; 00025 00026 RegionOfInterest(): 00027 x_offset(0), 00028 y_offset(0), 00029 height(0), 00030 width(0), 00031 do_rectify(0) 00032 { 00033 } 00034 00035 virtual int serialize(unsigned char *outbuffer) const 00036 { 00037 int offset = 0; 00038 *(outbuffer + offset + 0) = (this->x_offset >> (8 * 0)) & 0xFF; 00039 *(outbuffer + offset + 1) = (this->x_offset >> (8 * 1)) & 0xFF; 00040 *(outbuffer + offset + 2) = (this->x_offset >> (8 * 2)) & 0xFF; 00041 *(outbuffer + offset + 3) = (this->x_offset >> (8 * 3)) & 0xFF; 00042 offset += sizeof(this->x_offset); 00043 *(outbuffer + offset + 0) = (this->y_offset >> (8 * 0)) & 0xFF; 00044 *(outbuffer + offset + 1) = (this->y_offset >> (8 * 1)) & 0xFF; 00045 *(outbuffer + offset + 2) = (this->y_offset >> (8 * 2)) & 0xFF; 00046 *(outbuffer + offset + 3) = (this->y_offset >> (8 * 3)) & 0xFF; 00047 offset += sizeof(this->y_offset); 00048 *(outbuffer + offset + 0) = (this->height >> (8 * 0)) & 0xFF; 00049 *(outbuffer + offset + 1) = (this->height >> (8 * 1)) & 0xFF; 00050 *(outbuffer + offset + 2) = (this->height >> (8 * 2)) & 0xFF; 00051 *(outbuffer + offset + 3) = (this->height >> (8 * 3)) & 0xFF; 00052 offset += sizeof(this->height); 00053 *(outbuffer + offset + 0) = (this->width >> (8 * 0)) & 0xFF; 00054 *(outbuffer + offset + 1) = (this->width >> (8 * 1)) & 0xFF; 00055 *(outbuffer + offset + 2) = (this->width >> (8 * 2)) & 0xFF; 00056 *(outbuffer + offset + 3) = (this->width >> (8 * 3)) & 0xFF; 00057 offset += sizeof(this->width); 00058 union { 00059 bool real; 00060 uint8_t base; 00061 } u_do_rectify; 00062 u_do_rectify.real = this->do_rectify; 00063 *(outbuffer + offset + 0) = (u_do_rectify.base >> (8 * 0)) & 0xFF; 00064 offset += sizeof(this->do_rectify); 00065 return offset; 00066 } 00067 00068 virtual int deserialize(unsigned char *inbuffer) 00069 { 00070 int offset = 0; 00071 this->x_offset = ((uint32_t) (*(inbuffer + offset))); 00072 this->x_offset |= ((uint32_t) (*(inbuffer + offset + 1))) << (8 * 1); 00073 this->x_offset |= ((uint32_t) (*(inbuffer + offset + 2))) << (8 * 2); 00074 this->x_offset |= ((uint32_t) (*(inbuffer + offset + 3))) << (8 * 3); 00075 offset += sizeof(this->x_offset); 00076 this->y_offset = ((uint32_t) (*(inbuffer + offset))); 00077 this->y_offset |= ((uint32_t) (*(inbuffer + offset + 1))) << (8 * 1); 00078 this->y_offset |= ((uint32_t) (*(inbuffer + offset + 2))) << (8 * 2); 00079 this->y_offset |= ((uint32_t) (*(inbuffer + offset + 3))) << (8 * 3); 00080 offset += sizeof(this->y_offset); 00081 this->height = ((uint32_t) (*(inbuffer + offset))); 00082 this->height |= ((uint32_t) (*(inbuffer + offset + 1))) << (8 * 1); 00083 this->height |= ((uint32_t) (*(inbuffer + offset + 2))) << (8 * 2); 00084 this->height |= ((uint32_t) (*(inbuffer + offset + 3))) << (8 * 3); 00085 offset += sizeof(this->height); 00086 this->width = ((uint32_t) (*(inbuffer + offset))); 00087 this->width |= ((uint32_t) (*(inbuffer + offset + 1))) << (8 * 1); 00088 this->width |= ((uint32_t) (*(inbuffer + offset + 2))) << (8 * 2); 00089 this->width |= ((uint32_t) (*(inbuffer + offset + 3))) << (8 * 3); 00090 offset += sizeof(this->width); 00091 union { 00092 bool real; 00093 uint8_t base; 00094 } u_do_rectify; 00095 u_do_rectify.base = 0; 00096 u_do_rectify.base |= ((uint8_t) (*(inbuffer + offset + 0))) << (8 * 0); 00097 this->do_rectify = u_do_rectify.real; 00098 offset += sizeof(this->do_rectify); 00099 return offset; 00100 } 00101 00102 const char * getType(){ return "sensor_msgs/RegionOfInterest"; }; 00103 const char * getMD5(){ return "bdb633039d588fcccb441a4d43ccfe09"; }; 00104 00105 }; 00106 00107 } 00108 #endif
Generated on Tue Jul 12 2022 21:32:17 by
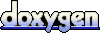