Working towards recieving twists
Fork of ros_lib_kinetic by
Embed:
(wiki syntax)
Show/hide line numbers
Plane.h
00001 #ifndef _ROS_shape_msgs_Plane_h 00002 #define _ROS_shape_msgs_Plane_h 00003 00004 #include <stdint.h> 00005 #include <string.h> 00006 #include <stdlib.h> 00007 #include "ros/msg.h" 00008 00009 namespace shape_msgs 00010 { 00011 00012 class Plane : public ros::Msg 00013 { 00014 public: 00015 double coef[4]; 00016 00017 Plane(): 00018 coef() 00019 { 00020 } 00021 00022 virtual int serialize(unsigned char *outbuffer) const 00023 { 00024 int offset = 0; 00025 for( uint32_t i = 0; i < 4; i++){ 00026 union { 00027 double real; 00028 uint64_t base; 00029 } u_coefi; 00030 u_coefi.real = this->coef[i]; 00031 *(outbuffer + offset + 0) = (u_coefi.base >> (8 * 0)) & 0xFF; 00032 *(outbuffer + offset + 1) = (u_coefi.base >> (8 * 1)) & 0xFF; 00033 *(outbuffer + offset + 2) = (u_coefi.base >> (8 * 2)) & 0xFF; 00034 *(outbuffer + offset + 3) = (u_coefi.base >> (8 * 3)) & 0xFF; 00035 *(outbuffer + offset + 4) = (u_coefi.base >> (8 * 4)) & 0xFF; 00036 *(outbuffer + offset + 5) = (u_coefi.base >> (8 * 5)) & 0xFF; 00037 *(outbuffer + offset + 6) = (u_coefi.base >> (8 * 6)) & 0xFF; 00038 *(outbuffer + offset + 7) = (u_coefi.base >> (8 * 7)) & 0xFF; 00039 offset += sizeof(this->coef[i]); 00040 } 00041 return offset; 00042 } 00043 00044 virtual int deserialize(unsigned char *inbuffer) 00045 { 00046 int offset = 0; 00047 for( uint32_t i = 0; i < 4; i++){ 00048 union { 00049 double real; 00050 uint64_t base; 00051 } u_coefi; 00052 u_coefi.base = 0; 00053 u_coefi.base |= ((uint64_t) (*(inbuffer + offset + 0))) << (8 * 0); 00054 u_coefi.base |= ((uint64_t) (*(inbuffer + offset + 1))) << (8 * 1); 00055 u_coefi.base |= ((uint64_t) (*(inbuffer + offset + 2))) << (8 * 2); 00056 u_coefi.base |= ((uint64_t) (*(inbuffer + offset + 3))) << (8 * 3); 00057 u_coefi.base |= ((uint64_t) (*(inbuffer + offset + 4))) << (8 * 4); 00058 u_coefi.base |= ((uint64_t) (*(inbuffer + offset + 5))) << (8 * 5); 00059 u_coefi.base |= ((uint64_t) (*(inbuffer + offset + 6))) << (8 * 6); 00060 u_coefi.base |= ((uint64_t) (*(inbuffer + offset + 7))) << (8 * 7); 00061 this->coef[i] = u_coefi.real; 00062 offset += sizeof(this->coef[i]); 00063 } 00064 return offset; 00065 } 00066 00067 const char * getType(){ return "shape_msgs/Plane"; }; 00068 const char * getMD5(){ return "2c1b92ed8f31492f8e73f6a4a44ca796"; }; 00069 00070 }; 00071 00072 } 00073 #endif
Generated on Tue Jul 12 2022 21:32:16 by
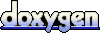