Working towards recieving twists
Fork of ros_lib_kinetic by
Embed:
(wiki syntax)
Show/hide line numbers
MultiDOFJointTrajectoryPoint.h
00001 #ifndef _ROS_trajectory_msgs_MultiDOFJointTrajectoryPoint_h 00002 #define _ROS_trajectory_msgs_MultiDOFJointTrajectoryPoint_h 00003 00004 #include <stdint.h> 00005 #include <string.h> 00006 #include <stdlib.h> 00007 #include "ros/msg.h" 00008 #include "geometry_msgs/Transform.h" 00009 #include "geometry_msgs/Twist.h" 00010 #include "ros/duration.h" 00011 00012 namespace trajectory_msgs 00013 { 00014 00015 class MultiDOFJointTrajectoryPoint : public ros::Msg 00016 { 00017 public: 00018 uint32_t transforms_length; 00019 typedef geometry_msgs::Transform _transforms_type; 00020 _transforms_type st_transforms; 00021 _transforms_type * transforms; 00022 uint32_t velocities_length; 00023 typedef geometry_msgs::Twist _velocities_type; 00024 _velocities_type st_velocities; 00025 _velocities_type * velocities; 00026 uint32_t accelerations_length; 00027 typedef geometry_msgs::Twist _accelerations_type; 00028 _accelerations_type st_accelerations; 00029 _accelerations_type * accelerations; 00030 typedef ros::Duration _time_from_start_type; 00031 _time_from_start_type time_from_start; 00032 00033 MultiDOFJointTrajectoryPoint(): 00034 transforms_length(0), transforms(NULL), 00035 velocities_length(0), velocities(NULL), 00036 accelerations_length(0), accelerations(NULL), 00037 time_from_start() 00038 { 00039 } 00040 00041 virtual int serialize(unsigned char *outbuffer) const 00042 { 00043 int offset = 0; 00044 *(outbuffer + offset + 0) = (this->transforms_length >> (8 * 0)) & 0xFF; 00045 *(outbuffer + offset + 1) = (this->transforms_length >> (8 * 1)) & 0xFF; 00046 *(outbuffer + offset + 2) = (this->transforms_length >> (8 * 2)) & 0xFF; 00047 *(outbuffer + offset + 3) = (this->transforms_length >> (8 * 3)) & 0xFF; 00048 offset += sizeof(this->transforms_length); 00049 for( uint32_t i = 0; i < transforms_length; i++){ 00050 offset += this->transforms[i].serialize(outbuffer + offset); 00051 } 00052 *(outbuffer + offset + 0) = (this->velocities_length >> (8 * 0)) & 0xFF; 00053 *(outbuffer + offset + 1) = (this->velocities_length >> (8 * 1)) & 0xFF; 00054 *(outbuffer + offset + 2) = (this->velocities_length >> (8 * 2)) & 0xFF; 00055 *(outbuffer + offset + 3) = (this->velocities_length >> (8 * 3)) & 0xFF; 00056 offset += sizeof(this->velocities_length); 00057 for( uint32_t i = 0; i < velocities_length; i++){ 00058 offset += this->velocities[i].serialize(outbuffer + offset); 00059 } 00060 *(outbuffer + offset + 0) = (this->accelerations_length >> (8 * 0)) & 0xFF; 00061 *(outbuffer + offset + 1) = (this->accelerations_length >> (8 * 1)) & 0xFF; 00062 *(outbuffer + offset + 2) = (this->accelerations_length >> (8 * 2)) & 0xFF; 00063 *(outbuffer + offset + 3) = (this->accelerations_length >> (8 * 3)) & 0xFF; 00064 offset += sizeof(this->accelerations_length); 00065 for( uint32_t i = 0; i < accelerations_length; i++){ 00066 offset += this->accelerations[i].serialize(outbuffer + offset); 00067 } 00068 *(outbuffer + offset + 0) = (this->time_from_start.sec >> (8 * 0)) & 0xFF; 00069 *(outbuffer + offset + 1) = (this->time_from_start.sec >> (8 * 1)) & 0xFF; 00070 *(outbuffer + offset + 2) = (this->time_from_start.sec >> (8 * 2)) & 0xFF; 00071 *(outbuffer + offset + 3) = (this->time_from_start.sec >> (8 * 3)) & 0xFF; 00072 offset += sizeof(this->time_from_start.sec); 00073 *(outbuffer + offset + 0) = (this->time_from_start.nsec >> (8 * 0)) & 0xFF; 00074 *(outbuffer + offset + 1) = (this->time_from_start.nsec >> (8 * 1)) & 0xFF; 00075 *(outbuffer + offset + 2) = (this->time_from_start.nsec >> (8 * 2)) & 0xFF; 00076 *(outbuffer + offset + 3) = (this->time_from_start.nsec >> (8 * 3)) & 0xFF; 00077 offset += sizeof(this->time_from_start.nsec); 00078 return offset; 00079 } 00080 00081 virtual int deserialize(unsigned char *inbuffer) 00082 { 00083 int offset = 0; 00084 uint32_t transforms_lengthT = ((uint32_t) (*(inbuffer + offset))); 00085 transforms_lengthT |= ((uint32_t) (*(inbuffer + offset + 1))) << (8 * 1); 00086 transforms_lengthT |= ((uint32_t) (*(inbuffer + offset + 2))) << (8 * 2); 00087 transforms_lengthT |= ((uint32_t) (*(inbuffer + offset + 3))) << (8 * 3); 00088 offset += sizeof(this->transforms_length); 00089 if(transforms_lengthT > transforms_length) 00090 this->transforms = (geometry_msgs::Transform*)realloc(this->transforms, transforms_lengthT * sizeof(geometry_msgs::Transform)); 00091 transforms_length = transforms_lengthT; 00092 for( uint32_t i = 0; i < transforms_length; i++){ 00093 offset += this->st_transforms.deserialize(inbuffer + offset); 00094 memcpy( &(this->transforms[i]), &(this->st_transforms), sizeof(geometry_msgs::Transform)); 00095 } 00096 uint32_t velocities_lengthT = ((uint32_t) (*(inbuffer + offset))); 00097 velocities_lengthT |= ((uint32_t) (*(inbuffer + offset + 1))) << (8 * 1); 00098 velocities_lengthT |= ((uint32_t) (*(inbuffer + offset + 2))) << (8 * 2); 00099 velocities_lengthT |= ((uint32_t) (*(inbuffer + offset + 3))) << (8 * 3); 00100 offset += sizeof(this->velocities_length); 00101 if(velocities_lengthT > velocities_length) 00102 this->velocities = (geometry_msgs::Twist*)realloc(this->velocities, velocities_lengthT * sizeof(geometry_msgs::Twist)); 00103 velocities_length = velocities_lengthT; 00104 for( uint32_t i = 0; i < velocities_length; i++){ 00105 offset += this->st_velocities.deserialize(inbuffer + offset); 00106 memcpy( &(this->velocities[i]), &(this->st_velocities), sizeof(geometry_msgs::Twist)); 00107 } 00108 uint32_t accelerations_lengthT = ((uint32_t) (*(inbuffer + offset))); 00109 accelerations_lengthT |= ((uint32_t) (*(inbuffer + offset + 1))) << (8 * 1); 00110 accelerations_lengthT |= ((uint32_t) (*(inbuffer + offset + 2))) << (8 * 2); 00111 accelerations_lengthT |= ((uint32_t) (*(inbuffer + offset + 3))) << (8 * 3); 00112 offset += sizeof(this->accelerations_length); 00113 if(accelerations_lengthT > accelerations_length) 00114 this->accelerations = (geometry_msgs::Twist*)realloc(this->accelerations, accelerations_lengthT * sizeof(geometry_msgs::Twist)); 00115 accelerations_length = accelerations_lengthT; 00116 for( uint32_t i = 0; i < accelerations_length; i++){ 00117 offset += this->st_accelerations.deserialize(inbuffer + offset); 00118 memcpy( &(this->accelerations[i]), &(this->st_accelerations), sizeof(geometry_msgs::Twist)); 00119 } 00120 this->time_from_start.sec = ((uint32_t) (*(inbuffer + offset))); 00121 this->time_from_start.sec |= ((uint32_t) (*(inbuffer + offset + 1))) << (8 * 1); 00122 this->time_from_start.sec |= ((uint32_t) (*(inbuffer + offset + 2))) << (8 * 2); 00123 this->time_from_start.sec |= ((uint32_t) (*(inbuffer + offset + 3))) << (8 * 3); 00124 offset += sizeof(this->time_from_start.sec); 00125 this->time_from_start.nsec = ((uint32_t) (*(inbuffer + offset))); 00126 this->time_from_start.nsec |= ((uint32_t) (*(inbuffer + offset + 1))) << (8 * 1); 00127 this->time_from_start.nsec |= ((uint32_t) (*(inbuffer + offset + 2))) << (8 * 2); 00128 this->time_from_start.nsec |= ((uint32_t) (*(inbuffer + offset + 3))) << (8 * 3); 00129 offset += sizeof(this->time_from_start.nsec); 00130 return offset; 00131 } 00132 00133 const char * getType(){ return "trajectory_msgs/MultiDOFJointTrajectoryPoint"; }; 00134 const char * getMD5(){ return "3ebe08d1abd5b65862d50e09430db776"; }; 00135 00136 }; 00137 00138 } 00139 #endif
Generated on Tue Jul 12 2022 21:32:16 by
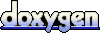