Working towards recieving twists
Fork of ros_lib_kinetic by
Embed:
(wiki syntax)
Show/hide line numbers
GroupState.h
00001 #ifndef _ROS_dynamic_reconfigure_GroupState_h 00002 #define _ROS_dynamic_reconfigure_GroupState_h 00003 00004 #include <stdint.h> 00005 #include <string.h> 00006 #include <stdlib.h> 00007 #include "ros/msg.h" 00008 00009 namespace dynamic_reconfigure 00010 { 00011 00012 class GroupState : public ros::Msg 00013 { 00014 public: 00015 typedef const char* _name_type; 00016 _name_type name; 00017 typedef bool _state_type; 00018 _state_type state; 00019 typedef int32_t _id_type; 00020 _id_type id; 00021 typedef int32_t _parent_type; 00022 _parent_type parent; 00023 00024 GroupState(): 00025 name(""), 00026 state(0), 00027 id(0), 00028 parent(0) 00029 { 00030 } 00031 00032 virtual int serialize(unsigned char *outbuffer) const 00033 { 00034 int offset = 0; 00035 uint32_t length_name = strlen(this->name); 00036 varToArr(outbuffer + offset, length_name); 00037 offset += 4; 00038 memcpy(outbuffer + offset, this->name, length_name); 00039 offset += length_name; 00040 union { 00041 bool real; 00042 uint8_t base; 00043 } u_state; 00044 u_state.real = this->state; 00045 *(outbuffer + offset + 0) = (u_state.base >> (8 * 0)) & 0xFF; 00046 offset += sizeof(this->state); 00047 union { 00048 int32_t real; 00049 uint32_t base; 00050 } u_id; 00051 u_id.real = this->id; 00052 *(outbuffer + offset + 0) = (u_id.base >> (8 * 0)) & 0xFF; 00053 *(outbuffer + offset + 1) = (u_id.base >> (8 * 1)) & 0xFF; 00054 *(outbuffer + offset + 2) = (u_id.base >> (8 * 2)) & 0xFF; 00055 *(outbuffer + offset + 3) = (u_id.base >> (8 * 3)) & 0xFF; 00056 offset += sizeof(this->id); 00057 union { 00058 int32_t real; 00059 uint32_t base; 00060 } u_parent; 00061 u_parent.real = this->parent; 00062 *(outbuffer + offset + 0) = (u_parent.base >> (8 * 0)) & 0xFF; 00063 *(outbuffer + offset + 1) = (u_parent.base >> (8 * 1)) & 0xFF; 00064 *(outbuffer + offset + 2) = (u_parent.base >> (8 * 2)) & 0xFF; 00065 *(outbuffer + offset + 3) = (u_parent.base >> (8 * 3)) & 0xFF; 00066 offset += sizeof(this->parent); 00067 return offset; 00068 } 00069 00070 virtual int deserialize(unsigned char *inbuffer) 00071 { 00072 int offset = 0; 00073 uint32_t length_name; 00074 arrToVar(length_name, (inbuffer + offset)); 00075 offset += 4; 00076 for(unsigned int k= offset; k< offset+length_name; ++k){ 00077 inbuffer[k-1]=inbuffer[k]; 00078 } 00079 inbuffer[offset+length_name-1]=0; 00080 this->name = (char *)(inbuffer + offset-1); 00081 offset += length_name; 00082 union { 00083 bool real; 00084 uint8_t base; 00085 } u_state; 00086 u_state.base = 0; 00087 u_state.base |= ((uint8_t) (*(inbuffer + offset + 0))) << (8 * 0); 00088 this->state = u_state.real; 00089 offset += sizeof(this->state); 00090 union { 00091 int32_t real; 00092 uint32_t base; 00093 } u_id; 00094 u_id.base = 0; 00095 u_id.base |= ((uint32_t) (*(inbuffer + offset + 0))) << (8 * 0); 00096 u_id.base |= ((uint32_t) (*(inbuffer + offset + 1))) << (8 * 1); 00097 u_id.base |= ((uint32_t) (*(inbuffer + offset + 2))) << (8 * 2); 00098 u_id.base |= ((uint32_t) (*(inbuffer + offset + 3))) << (8 * 3); 00099 this->id = u_id.real; 00100 offset += sizeof(this->id); 00101 union { 00102 int32_t real; 00103 uint32_t base; 00104 } u_parent; 00105 u_parent.base = 0; 00106 u_parent.base |= ((uint32_t) (*(inbuffer + offset + 0))) << (8 * 0); 00107 u_parent.base |= ((uint32_t) (*(inbuffer + offset + 1))) << (8 * 1); 00108 u_parent.base |= ((uint32_t) (*(inbuffer + offset + 2))) << (8 * 2); 00109 u_parent.base |= ((uint32_t) (*(inbuffer + offset + 3))) << (8 * 3); 00110 this->parent = u_parent.real; 00111 offset += sizeof(this->parent); 00112 return offset; 00113 } 00114 00115 const char * getType(){ return "dynamic_reconfigure/GroupState"; }; 00116 const char * getMD5(){ return "a2d87f51dc22930325041a2f8b1571f8"; }; 00117 00118 }; 00119 00120 } 00121 #endif
Generated on Tue Jul 12 2022 21:32:16 by
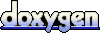