Working towards recieving twists
Fork of ros_lib_kinetic by
Embed:
(wiki syntax)
Show/hide line numbers
FollowJointTrajectoryGoal.h
00001 #ifndef _ROS_control_msgs_FollowJointTrajectoryGoal_h 00002 #define _ROS_control_msgs_FollowJointTrajectoryGoal_h 00003 00004 #include <stdint.h> 00005 #include <string.h> 00006 #include <stdlib.h> 00007 #include "ros/msg.h" 00008 #include "trajectory_msgs/JointTrajectory.h" 00009 #include "control_msgs/JointTolerance.h" 00010 #include "ros/duration.h" 00011 00012 namespace control_msgs 00013 { 00014 00015 class FollowJointTrajectoryGoal : public ros::Msg 00016 { 00017 public: 00018 typedef trajectory_msgs::JointTrajectory _trajectory_type; 00019 _trajectory_type trajectory; 00020 uint32_t path_tolerance_length; 00021 typedef control_msgs::JointTolerance _path_tolerance_type; 00022 _path_tolerance_type st_path_tolerance; 00023 _path_tolerance_type * path_tolerance; 00024 uint32_t goal_tolerance_length; 00025 typedef control_msgs::JointTolerance _goal_tolerance_type; 00026 _goal_tolerance_type st_goal_tolerance; 00027 _goal_tolerance_type * goal_tolerance; 00028 typedef ros::Duration _goal_time_tolerance_type; 00029 _goal_time_tolerance_type goal_time_tolerance; 00030 00031 FollowJointTrajectoryGoal(): 00032 trajectory(), 00033 path_tolerance_length(0), path_tolerance(NULL), 00034 goal_tolerance_length(0), goal_tolerance(NULL), 00035 goal_time_tolerance() 00036 { 00037 } 00038 00039 virtual int serialize(unsigned char *outbuffer) const 00040 { 00041 int offset = 0; 00042 offset += this->trajectory.serialize(outbuffer + offset); 00043 *(outbuffer + offset + 0) = (this->path_tolerance_length >> (8 * 0)) & 0xFF; 00044 *(outbuffer + offset + 1) = (this->path_tolerance_length >> (8 * 1)) & 0xFF; 00045 *(outbuffer + offset + 2) = (this->path_tolerance_length >> (8 * 2)) & 0xFF; 00046 *(outbuffer + offset + 3) = (this->path_tolerance_length >> (8 * 3)) & 0xFF; 00047 offset += sizeof(this->path_tolerance_length); 00048 for( uint32_t i = 0; i < path_tolerance_length; i++){ 00049 offset += this->path_tolerance[i].serialize(outbuffer + offset); 00050 } 00051 *(outbuffer + offset + 0) = (this->goal_tolerance_length >> (8 * 0)) & 0xFF; 00052 *(outbuffer + offset + 1) = (this->goal_tolerance_length >> (8 * 1)) & 0xFF; 00053 *(outbuffer + offset + 2) = (this->goal_tolerance_length >> (8 * 2)) & 0xFF; 00054 *(outbuffer + offset + 3) = (this->goal_tolerance_length >> (8 * 3)) & 0xFF; 00055 offset += sizeof(this->goal_tolerance_length); 00056 for( uint32_t i = 0; i < goal_tolerance_length; i++){ 00057 offset += this->goal_tolerance[i].serialize(outbuffer + offset); 00058 } 00059 *(outbuffer + offset + 0) = (this->goal_time_tolerance.sec >> (8 * 0)) & 0xFF; 00060 *(outbuffer + offset + 1) = (this->goal_time_tolerance.sec >> (8 * 1)) & 0xFF; 00061 *(outbuffer + offset + 2) = (this->goal_time_tolerance.sec >> (8 * 2)) & 0xFF; 00062 *(outbuffer + offset + 3) = (this->goal_time_tolerance.sec >> (8 * 3)) & 0xFF; 00063 offset += sizeof(this->goal_time_tolerance.sec); 00064 *(outbuffer + offset + 0) = (this->goal_time_tolerance.nsec >> (8 * 0)) & 0xFF; 00065 *(outbuffer + offset + 1) = (this->goal_time_tolerance.nsec >> (8 * 1)) & 0xFF; 00066 *(outbuffer + offset + 2) = (this->goal_time_tolerance.nsec >> (8 * 2)) & 0xFF; 00067 *(outbuffer + offset + 3) = (this->goal_time_tolerance.nsec >> (8 * 3)) & 0xFF; 00068 offset += sizeof(this->goal_time_tolerance.nsec); 00069 return offset; 00070 } 00071 00072 virtual int deserialize(unsigned char *inbuffer) 00073 { 00074 int offset = 0; 00075 offset += this->trajectory.deserialize(inbuffer + offset); 00076 uint32_t path_tolerance_lengthT = ((uint32_t) (*(inbuffer + offset))); 00077 path_tolerance_lengthT |= ((uint32_t) (*(inbuffer + offset + 1))) << (8 * 1); 00078 path_tolerance_lengthT |= ((uint32_t) (*(inbuffer + offset + 2))) << (8 * 2); 00079 path_tolerance_lengthT |= ((uint32_t) (*(inbuffer + offset + 3))) << (8 * 3); 00080 offset += sizeof(this->path_tolerance_length); 00081 if(path_tolerance_lengthT > path_tolerance_length) 00082 this->path_tolerance = (control_msgs::JointTolerance*)realloc(this->path_tolerance, path_tolerance_lengthT * sizeof(control_msgs::JointTolerance)); 00083 path_tolerance_length = path_tolerance_lengthT; 00084 for( uint32_t i = 0; i < path_tolerance_length; i++){ 00085 offset += this->st_path_tolerance.deserialize(inbuffer + offset); 00086 memcpy( &(this->path_tolerance[i]), &(this->st_path_tolerance), sizeof(control_msgs::JointTolerance)); 00087 } 00088 uint32_t goal_tolerance_lengthT = ((uint32_t) (*(inbuffer + offset))); 00089 goal_tolerance_lengthT |= ((uint32_t) (*(inbuffer + offset + 1))) << (8 * 1); 00090 goal_tolerance_lengthT |= ((uint32_t) (*(inbuffer + offset + 2))) << (8 * 2); 00091 goal_tolerance_lengthT |= ((uint32_t) (*(inbuffer + offset + 3))) << (8 * 3); 00092 offset += sizeof(this->goal_tolerance_length); 00093 if(goal_tolerance_lengthT > goal_tolerance_length) 00094 this->goal_tolerance = (control_msgs::JointTolerance*)realloc(this->goal_tolerance, goal_tolerance_lengthT * sizeof(control_msgs::JointTolerance)); 00095 goal_tolerance_length = goal_tolerance_lengthT; 00096 for( uint32_t i = 0; i < goal_tolerance_length; i++){ 00097 offset += this->st_goal_tolerance.deserialize(inbuffer + offset); 00098 memcpy( &(this->goal_tolerance[i]), &(this->st_goal_tolerance), sizeof(control_msgs::JointTolerance)); 00099 } 00100 this->goal_time_tolerance.sec = ((uint32_t) (*(inbuffer + offset))); 00101 this->goal_time_tolerance.sec |= ((uint32_t) (*(inbuffer + offset + 1))) << (8 * 1); 00102 this->goal_time_tolerance.sec |= ((uint32_t) (*(inbuffer + offset + 2))) << (8 * 2); 00103 this->goal_time_tolerance.sec |= ((uint32_t) (*(inbuffer + offset + 3))) << (8 * 3); 00104 offset += sizeof(this->goal_time_tolerance.sec); 00105 this->goal_time_tolerance.nsec = ((uint32_t) (*(inbuffer + offset))); 00106 this->goal_time_tolerance.nsec |= ((uint32_t) (*(inbuffer + offset + 1))) << (8 * 1); 00107 this->goal_time_tolerance.nsec |= ((uint32_t) (*(inbuffer + offset + 2))) << (8 * 2); 00108 this->goal_time_tolerance.nsec |= ((uint32_t) (*(inbuffer + offset + 3))) << (8 * 3); 00109 offset += sizeof(this->goal_time_tolerance.nsec); 00110 return offset; 00111 } 00112 00113 const char * getType(){ return "control_msgs/FollowJointTrajectoryGoal"; }; 00114 const char * getMD5(){ return "69636787b6ecbde4d61d711979bc7ecb"; }; 00115 00116 }; 00117 00118 } 00119 #endif
Generated on Tue Jul 12 2022 21:32:15 by
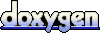