Working towards recieving twists
Fork of ros_lib_kinetic by
Embed:
(wiki syntax)
Show/hide line numbers
ConfigDescription.h
00001 #ifndef _ROS_dynamic_reconfigure_ConfigDescription_h 00002 #define _ROS_dynamic_reconfigure_ConfigDescription_h 00003 00004 #include <stdint.h> 00005 #include <string.h> 00006 #include <stdlib.h> 00007 #include "ros/msg.h" 00008 #include "dynamic_reconfigure/Group.h" 00009 #include "dynamic_reconfigure/Config.h" 00010 00011 namespace dynamic_reconfigure 00012 { 00013 00014 class ConfigDescription : public ros::Msg 00015 { 00016 public: 00017 uint32_t groups_length; 00018 typedef dynamic_reconfigure::Group _groups_type; 00019 _groups_type st_groups; 00020 _groups_type * groups; 00021 typedef dynamic_reconfigure::Config _max_type; 00022 _max_type max; 00023 typedef dynamic_reconfigure::Config _min_type; 00024 _min_type min; 00025 typedef dynamic_reconfigure::Config _dflt_type; 00026 _dflt_type dflt; 00027 00028 ConfigDescription(): 00029 groups_length(0), groups(NULL), 00030 max(), 00031 min(), 00032 dflt() 00033 { 00034 } 00035 00036 virtual int serialize(unsigned char *outbuffer) const 00037 { 00038 int offset = 0; 00039 *(outbuffer + offset + 0) = (this->groups_length >> (8 * 0)) & 0xFF; 00040 *(outbuffer + offset + 1) = (this->groups_length >> (8 * 1)) & 0xFF; 00041 *(outbuffer + offset + 2) = (this->groups_length >> (8 * 2)) & 0xFF; 00042 *(outbuffer + offset + 3) = (this->groups_length >> (8 * 3)) & 0xFF; 00043 offset += sizeof(this->groups_length); 00044 for( uint32_t i = 0; i < groups_length; i++){ 00045 offset += this->groups[i].serialize(outbuffer + offset); 00046 } 00047 offset += this->max.serialize(outbuffer + offset); 00048 offset += this->min.serialize(outbuffer + offset); 00049 offset += this->dflt.serialize(outbuffer + offset); 00050 return offset; 00051 } 00052 00053 virtual int deserialize(unsigned char *inbuffer) 00054 { 00055 int offset = 0; 00056 uint32_t groups_lengthT = ((uint32_t) (*(inbuffer + offset))); 00057 groups_lengthT |= ((uint32_t) (*(inbuffer + offset + 1))) << (8 * 1); 00058 groups_lengthT |= ((uint32_t) (*(inbuffer + offset + 2))) << (8 * 2); 00059 groups_lengthT |= ((uint32_t) (*(inbuffer + offset + 3))) << (8 * 3); 00060 offset += sizeof(this->groups_length); 00061 if(groups_lengthT > groups_length) 00062 this->groups = (dynamic_reconfigure::Group*)realloc(this->groups, groups_lengthT * sizeof(dynamic_reconfigure::Group)); 00063 groups_length = groups_lengthT; 00064 for( uint32_t i = 0; i < groups_length; i++){ 00065 offset += this->st_groups.deserialize(inbuffer + offset); 00066 memcpy( &(this->groups[i]), &(this->st_groups), sizeof(dynamic_reconfigure::Group)); 00067 } 00068 offset += this->max.deserialize(inbuffer + offset); 00069 offset += this->min.deserialize(inbuffer + offset); 00070 offset += this->dflt.deserialize(inbuffer + offset); 00071 return offset; 00072 } 00073 00074 const char * getType(){ return "dynamic_reconfigure/ConfigDescription"; }; 00075 const char * getMD5(){ return "757ce9d44ba8ddd801bb30bc456f946f"; }; 00076 00077 }; 00078 00079 } 00080 #endif
Generated on Tue Jul 12 2022 21:32:15 by
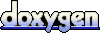