Working towards recieving twists
Fork of ros_lib_kinetic by
Embed:
(wiki syntax)
Show/hide line numbers
Color.h
00001 #ifndef _ROS_turtlesim_Color_h 00002 #define _ROS_turtlesim_Color_h 00003 00004 #include <stdint.h> 00005 #include <string.h> 00006 #include <stdlib.h> 00007 #include "ros/msg.h" 00008 00009 namespace turtlesim 00010 { 00011 00012 class Color : public ros::Msg 00013 { 00014 public: 00015 typedef uint8_t _r_type; 00016 _r_type r; 00017 typedef uint8_t _g_type; 00018 _g_type g; 00019 typedef uint8_t _b_type; 00020 _b_type b; 00021 00022 Color(): 00023 r(0), 00024 g(0), 00025 b(0) 00026 { 00027 } 00028 00029 virtual int serialize(unsigned char *outbuffer) const 00030 { 00031 int offset = 0; 00032 *(outbuffer + offset + 0) = (this->r >> (8 * 0)) & 0xFF; 00033 offset += sizeof(this->r); 00034 *(outbuffer + offset + 0) = (this->g >> (8 * 0)) & 0xFF; 00035 offset += sizeof(this->g); 00036 *(outbuffer + offset + 0) = (this->b >> (8 * 0)) & 0xFF; 00037 offset += sizeof(this->b); 00038 return offset; 00039 } 00040 00041 virtual int deserialize(unsigned char *inbuffer) 00042 { 00043 int offset = 0; 00044 this->r = ((uint8_t) (*(inbuffer + offset))); 00045 offset += sizeof(this->r); 00046 this->g = ((uint8_t) (*(inbuffer + offset))); 00047 offset += sizeof(this->g); 00048 this->b = ((uint8_t) (*(inbuffer + offset))); 00049 offset += sizeof(this->b); 00050 return offset; 00051 } 00052 00053 const char * getType(){ return "turtlesim/Color"; }; 00054 const char * getMD5(){ return "353891e354491c51aabe32df673fb446"; }; 00055 00056 }; 00057 00058 } 00059 #endif
Generated on Tue Jul 12 2022 21:32:15 by
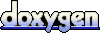