PID Library Brought in from the PID Arduino Library
Dependents: Basic_PID wheelchaircontrol wheelchaircontrolRosCom wheelchaircontrol ... more
PID.h
00001 #ifndef PID_H 00002 #define PID_H 00003 00004 #include "mbed.h" 00005 00006 class PID 00007 { 00008 public: 00009 #define AUTOMATIC 1 00010 #define MANUAL 0 00011 #define DIRECT 0 00012 #define REVERSE 1 00013 #define P_ON_M 0 00014 #define P_ON_E 1 00015 Timer PIDtimer; 00016 PID(volatile double* Input, volatile double* Output, volatile double* Setpoint, 00017 double Kp, double Ki, double Kd, int POn, int ControllerDirection); 00018 void SetMode(int Mode); // * sets PID to either Manual (0) or Auto (non-0) 00019 00020 bool Compute(); // * performs the PID calculation. it should be 00021 // called every time loop() cycles. ON/OFF and 00022 // calculation frequency can be set using SetMode 00023 // SetSampleTime respectively 00024 00025 void SetOutputLimits(double, double); // * clamps the output to a specific range. 0-255 by default, but 00026 // it's likely the user will want to change this depending on 00027 // the application 00028 00029 00030 00031 //available but not commonly used functions ******************************************************** 00032 void SetTunings(double, double, // * While most users will set the tunings once in the 00033 double); // constructor, this function gives the user the option 00034 // of changing tunings during runtime for Adaptive control 00035 void SetTunings(double, double, // * overload for specifying proportional mode 00036 double, int); 00037 00038 void SetControllerDirection(int); // * Sets the Direction, or "Action" of the controller. DIRECT 00039 // means the output will increase when error is positive. REVERSE 00040 // means the opposite. it's very unlikely that this will be needed 00041 // once it is set in the constructor.*/ 00042 void SetSampleTime(int); // * sets the frequency, in Milliseconds, with which 00043 // the PID calculation is performed. default is 100 00044 00045 00046 00047 00048 //Display functions **************************************************************** 00049 double GetKp(); // These functions query the pid for interal values. 00050 double GetKi(); // they were created mainly for the pid front-end, 00051 double GetKd(); // where it's important to know what is actually 00052 int GetMode(); // inside the PID. 00053 int GetDirection(); // 00054 00055 private: 00056 void Initialize(); 00057 00058 double dispKp; // * we'll hold on to the tuning parameters in user-entered 00059 double dispKi; // format for display purposes 00060 double dispKd; // 00061 00062 double kp; // * (P)roportional Tuning Parameter 00063 double ki; // * (I)ntegral Tuning Parameter 00064 double kd; // * (D)erivative Tuning Parameter 00065 00066 int controllerDirection; 00067 int pOn; 00068 00069 volatile double *myInput; // * Pointers to the Input, Output, and Setpoint variables 00070 volatile double *myOutput; // This creates a hard link between the variables and the 00071 volatile double *mySetpoint; // PID, freeing the user from having to constantly tell us 00072 // what these values are. with pointers we'll just know. 00073 00074 double lastTime; 00075 double outputSum, lastInput; 00076 00077 double SampleTime; 00078 double outMin, outMax; 00079 bool inAuto, pOnE; 00080 00081 }; 00082 00083 #endif 00084
Generated on Tue Jul 12 2022 21:32:38 by
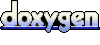