Library For DHT
Embed:
(wiki syntax)
Show/hide line numbers
DHT.cpp
00001 #include "DHT.h" 00002 00003 #define DHT_DATA_BIT_COUNT 41 00004 00005 DHT::DHT(PinName pin,int DHTtype) { 00006 _pin = pin; 00007 _DHTtype = DHTtype; 00008 _firsttime=true; 00009 } 00010 00011 DHT::~DHT() { 00012 } 00013 00014 int DHT::readData() { 00015 int i, j, retryCount,b; 00016 unsigned int bitTimes[DHT_DATA_BIT_COUNT]; 00017 00018 eError err = ERROR_NONE; 00019 time_t currentTime = time(NULL); 00020 00021 DigitalInOut DHT_io(_pin); 00022 00023 for (i = 0; i < DHT_DATA_BIT_COUNT; i++) { 00024 bitTimes[i] = 0; 00025 } 00026 00027 if (!_firsttime) { 00028 if (int(currentTime - _lastReadTime) < 2) { 00029 err = ERROR_NO_PATIENCE; 00030 return err; 00031 } 00032 } else { 00033 _firsttime=false; 00034 _lastReadTime=currentTime; 00035 } 00036 retryCount = 0; 00037 00038 do { 00039 if (retryCount > 125) { 00040 err = BUS_BUSY; 00041 return err; 00042 } 00043 retryCount ++; 00044 wait_us(2); 00045 } while ((DHT_io==0)); 00046 00047 00048 DHT_io.output(); 00049 DHT_io = 0; 00050 wait_ms(18); 00051 DHT_io = 1; 00052 wait_us(40); 00053 DHT_io.input(); 00054 00055 retryCount = 0; 00056 do { 00057 if (retryCount > 40) { 00058 err = ERROR_NOT_PRESENT; 00059 return err; 00060 } 00061 retryCount++; 00062 wait_us(1); 00063 } while ((DHT_io==1)); 00064 00065 if (err != ERROR_NONE) { 00066 return err; 00067 } 00068 00069 wait_us(80); 00070 00071 for (i = 0; i < 5; i++) { 00072 for (j = 0; j < 8; j++) { 00073 00074 retryCount = 0; 00075 do { 00076 if (retryCount > 75) { 00077 err = ERROR_DATA_TIMEOUT; 00078 return err; 00079 } 00080 retryCount++; 00081 wait_us(1); 00082 } while (DHT_io == 0); 00083 wait_us(40); 00084 bitTimes[i*8+j]=DHT_io; 00085 00086 int count = 0; 00087 while (DHT_io == 1 && count < 100) { 00088 wait_us(1); 00089 count++; 00090 } 00091 } 00092 } 00093 DHT_io.output(); 00094 DHT_io = 1; 00095 for (i = 0; i < 5; i++) { 00096 b=0; 00097 for (j=0; j<8; j++) { 00098 if (bitTimes[i*8+j+1] > 0) { 00099 b |= ( 1 << (7-j)); 00100 } 00101 } 00102 DHT_data[i]=b; 00103 } 00104 00105 if (DHT_data[4] == ((DHT_data[0] + DHT_data[1] + DHT_data[2] + DHT_data[3]) & 0xFF)) { 00106 _lastReadTime = currentTime; 00107 _lastTemperature=CalcTemperature(); 00108 _lastHumidity=CalcHumidity(); 00109 00110 } else { 00111 err = ERROR_CHECKSUM; 00112 } 00113 00114 return err; 00115 00116 } 00117 00118 float DHT::CalcTemperature() { 00119 int v; 00120 float outvalue; 00121 switch (_DHTtype) { 00122 case DHT11: 00123 v = DHT_data[2]; 00124 return float(v); 00125 case DHT22: 00126 v = DHT_data[2] & 0x7F; 00127 v *= 256; 00128 v += DHT_data[3]; 00129 outvalue=(float) v/10; 00130 if (DHT_data[2] & 0x80) 00131 outvalue *= -1; 00132 return outvalue; 00133 } 00134 return 0; 00135 } 00136 00137 float DHT::ReadHumidity() { 00138 return _lastHumidity; 00139 } 00140 00141 float DHT::ConvertCelciustoFarenheit(float celsius) { 00142 return celsius * 9 / 5 + 32; 00143 } 00144 00145 float DHT::ConvertCelciustoKelvin(float celsius) { 00146 return celsius + 273.15; 00147 } 00148 00149 // dewPoint function NOAA 00150 // reference: http://wahiduddin.net/calc/density_algorithms.htm 00151 float DHT::CalcdewPoint(float celsius, float humidity) { 00152 float A0= 373.15/(273.15 + celsius); 00153 float SUM = -7.90298 * (A0-1); 00154 SUM += 5.02808 * log10(A0); 00155 SUM += -1.3816e-7 * (pow(10, (11.344*(1-1/A0)))-1) ; 00156 SUM += 8.1328e-3 * (pow(10,(-3.49149*(A0-1)))-1) ; 00157 SUM += log10(1013.246); 00158 float VP = pow(10, SUM-3) * humidity; 00159 float T = log(VP/0.61078); // temp var 00160 return (241.88 * T) / (17.558-T); 00161 } 00162 00163 // delta max = 0.6544 wrt dewPoint() 00164 // 5x faster than dewPoint() 00165 // reference: http://en.wikipedia.org/wiki/Dew_point 00166 float DHT::CalcdewPointFast(float celsius, float humidity) 00167 { 00168 float a = 17.271; 00169 float b = 237.7; 00170 float temp = (a * celsius) / (b + celsius) + log(humidity/100); 00171 float Td = (b * temp) / (a - temp); 00172 return Td; 00173 } 00174 00175 float DHT::ReadTemperature(eScale Scale) { 00176 if (Scale == FARENHEIT) 00177 return ConvertCelciustoFarenheit(_lastTemperature); 00178 else if (Scale == KELVIN) 00179 return ConvertCelciustoKelvin(_lastTemperature); 00180 else 00181 return _lastTemperature; 00182 } 00183 00184 float DHT::CalcHumidity() { 00185 int v; 00186 float outvalue; 00187 00188 switch (_DHTtype) { 00189 case DHT11: 00190 v = DHT_data[0]; 00191 return float(v); 00192 case DHT22: 00193 v = DHT_data[0]; 00194 v *= 256; 00195 v += DHT_data[1]; 00196 outvalue=(float) v/10; 00197 return outvalue; 00198 } 00199 return 0; 00200 } 00201 00202
Generated on Tue Jul 19 2022 10:01:12 by
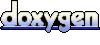