
Still won't work
Dependencies: mbed wave_player 4DGL-uLCD-SE MMA8452
map.h
00001 #ifndef MAP_H 00002 #define MAP_H 00003 00004 #include "hash_table.h" 00005 00006 /** 00007 * A structure to represent the map. The implementation is private. 00008 */ 00009 struct Map; 00010 00011 // A function pointer type for drawing MapItems. 00012 // All tiles are 11x11 blocks. 00013 // u,v is the top left corner pixel of the block 00014 typedef void (*DrawFunc)(int u, int v); 00015 00016 /** 00017 * The data for elements in the map. Each item in the map HashTable is a 00018 * MapItem. 00019 */ 00020 typedef struct { 00021 /** 00022 * Indicates the "type" of the MapItem: WALL, DOOR, PLANT, etc. This is 00023 * useful for determining how to interact with the object when updating the 00024 * game state. 00025 */ 00026 int type; 00027 00028 /** 00029 * A function pointer to the drawing function for this item. Used by draw_game. 00030 */ 00031 DrawFunc draw; 00032 00033 /** 00034 * If zero, this item should block character motion. 00035 */ 00036 int walkable; 00037 00038 /** 00039 * Arbitrary extra data for the MapItem. Could be useful for keeping up with 00040 * special information, like where a set of stairs should take the player. 00041 * 00042 * Iterpretation of this can depend on the type of the MapItem. For example, 00043 * a WALL probably doesn't need to use this (it can be NULL), where an NPC 00044 * might use it to store game state (have I given the player the key yet?). 00045 */ 00046 void* data; 00047 } MapItem; 00048 00049 typedef struct { 00050 int tm; 00051 int tx, ty; 00052 } StairsData; 00053 00054 // MapItem types 00055 // Define more of these! 00056 #define WALL 0 00057 #define PLANT 1 00058 00059 /** 00060 * Initializes the internal structures for all maps. This does not populate 00061 * the map with items, but allocates space for them, initializes the hash tables, 00062 * and sets the width and height. 00063 */ 00064 void maps_init(); 00065 00066 /** 00067 * Returns a pointer to the active map. 00068 */ 00069 Map* get_active_map(); 00070 00071 /** 00072 * Sets the active map to map m, where m is the index of the map to activate. 00073 * Returns a pointer to the new active map. 00074 */ 00075 Map* set_active_map(int m); 00076 00077 /** 00078 * Returns the map m, regardless of whether it is the active map. This function 00079 * does not change the active map. 00080 */ 00081 Map* get_map(int m); 00082 00083 /** 00084 * Print the active map to the serial console. 00085 */ 00086 void print_map(); 00087 00088 // Access 00089 /** 00090 * Returns the width of the active map. 00091 */ 00092 int map_width(); 00093 00094 /** 00095 * Returns the heigh of the active map. 00096 */ 00097 int map_height(); 00098 00099 /** 00100 * Returns the total number of cells in the active map. 00101 */ 00102 int map_area(); 00103 00104 /** 00105 * Returns the MapItem immediately above the given location. 00106 */ 00107 MapItem* get_north(int x, int y); 00108 00109 /** 00110 * Returns the MapItem immediately below the given location. 00111 */ 00112 MapItem* get_south(int x, int y); 00113 00114 /** 00115 * Returns the MapItem immediately to the right of the given location. 00116 */ 00117 MapItem* get_east(int x, int y); 00118 00119 /** 00120 * Returns the MapItem immediately to the left of the given location. 00121 */ 00122 MapItem* get_west(int x, int y); 00123 00124 /** 00125 * Returns the MapItem at the given location. 00126 */ 00127 MapItem* get_here(int x, int y); 00128 00129 // Directions, for using the modification functions 00130 #define HORIZONTAL 0 00131 #define VERTICAL 1 00132 00133 /** 00134 * If there is a MapItem at (x,y), remove it from the map. 00135 */ 00136 void map_erase(int x, int y); 00137 00138 /** 00139 * Add WALL items in a line of length len beginning at (x,y). 00140 * If dir == HORIZONTAL, the line is in the direction of increasing x. 00141 * If dir == VERTICAL, the line is in the direction of increasing y. 00142 * 00143 * If there are already items in the map that collide with this line, they are 00144 * erased. 00145 */ 00146 void add_wall(int x, int y, int dir, int len); 00147 00148 /** 00149 * Add a PLANT item at (x,y). If there is already a MapItem at (x,y), erase it 00150 * before adding the plant. 00151 */ 00152 void add_plant(int x, int y); 00153 00154 #endif //MAP_H
Generated on Fri Aug 19 2022 16:39:02 by
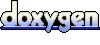