example using DS3234 RTC connected to BBC micro:bit using SPI
Embed:
(wiki syntax)
Show/hide line numbers
SparkFunDS3234RTC.h
00001 /****************************************************************************** 00002 SparkFunDS3234RTC.h 00003 Jim Lindblom @ SparkFun Electronics 00004 original creation date: October 2, 2016 00005 https://github.com/sparkfun/SparkFun_DS3234_RTC_Arduino_Library 00006 00007 Prototypes the DS3234 class. Defines sketch-usable global variables. 00008 00009 Resources: 00010 Wire.h - Arduino I2C Library 00011 00012 Development environment specifics: 00013 Arduino 1.6.8 00014 SparkFun RedBoard 00015 SparkFun Real Time Clock Module (v14) 00016 00017 Updated 16 October 2016 by Vassilis Serasidis <avrsite@yahoo.gr> 00018 - Added readFromSRAM' and 'writeToSRAM' functions 00019 00020 ******************************************************************************/ 00021 //#include <Arduino.h> 00022 #include "mbed.h" 00023 #include "MicroBit.h" 00024 00025 #ifndef SPARKFUNDS3234RTC_H 00026 #define SPARKFUNDS3234RTC_H 00027 00028 #define TWELVE_HOUR_MODE (1<<6) // 12/24-hour Mode bit in Hour register 00029 #define TWELVE_HOUR_PM (1<<5) // am/pm bit in hour register 00030 00031 #define AM false 00032 #define PM true 00033 00034 #define SQW_CONTROL_MASK 0xE3 // SQW bit(s) mask in control register 00035 #define SQW_ENABLE_BIT (1<<2) // SQW output enable bit in control register 00036 00037 #define ALARM_MODE_BIT (1<<7) // Alarm mode bit (A1M1, A1M2, etc) in alarm registers 00038 #define ALARM_DAY_BIT (1<<6) // Alarm day/date control bit in alarm day registers 00039 #define ALARM_1_FLAG_BIT (1<<0) // Alarm 1 flag in control/status register 00040 #define ALARM_2_FLAG_BIT (1<<1) // Alarm 2 flag in control/status register 00041 #define ALARM_INTCN_BIT (1<<2) // Interrupt-enable bit in control register 00042 00043 #define TIME_ARRAY_LENGTH 7 // Total number of writable time values in device 00044 enum time_order { 00045 TIME_SECONDS, // 0 00046 TIME_MINUTES, // 1 00047 TIME_HOURS, // 2 00048 TIME_DAY, // 3 00049 TIME_DATE, // 4 00050 TIME_MONTH, // 5 00051 TIME_YEAR, // 6 00052 }; 00053 00054 // sqw_rate -- enum for possible SQW pin output settings 00055 enum sqw_rate { 00056 SQW_SQUARE_1, // 0 00057 SQW_SQUARE_1K, // 1 00058 SQW_SQUARE_4K, // 2 00059 SQW_SQUARE_8K // 3 00060 }; 00061 00062 // DS3234_registers -- Definition of DS3234 registers 00063 enum DS3234_registers { 00064 DS3234_REGISTER_SECONDS, // 0x00 00065 DS3234_REGISTER_MINUTES, // 0x01 00066 DS3234_REGISTER_HOURS, // 0x02 00067 DS3234_REGISTER_DAY, // 0x03 00068 DS3234_REGISTER_DATE, // 0x04 00069 DS3234_REGISTER_MONTH, // 0x05 00070 DS3234_REGISTER_YEAR, // 0x06 00071 DS3234_REGISTER_A1SEC, // 0x07 00072 DS3234_REGISTER_A1MIN, // 0x08 00073 DS3234_REGISTER_A1HR, // 0x09 00074 DS3234_REGISTER_A1DA, // 0x0A 00075 DS3234_REGISTER_A2MIN, // 0x0B 00076 DS3234_REGISTER_A2HR, // 0x0C 00077 DS3234_REGISTER_A2DA, // 0x0D 00078 DS3234_REGISTER_CONTROL, // 0x0E 00079 DS3234_REGISTER_STATUS, // 0x0F 00080 DS3234_REGISTER_XTAL, // 0x10 00081 DS3234_REGISTER_TEMPM, // 0x11 00082 DS3234_REGISTER_TEMPL, // 0x12 00083 DS3234_REGISTER_TEMPEN, // 0x13 00084 DS3234_REGISTER_RESERV1, // 0x14 00085 DS3234_REGISTER_RESERV2, // 0x15 00086 DS3234_REGISTER_RESERV3, // 0x16 00087 DS3234_REGISTER_RESERV4, // 0x17 00088 DS3234_REGISTER_SRAMA, // 0x18 00089 DS3234_REGISTER_SRAMD // 0x19 00090 }; 00091 00092 // Base register for complete time/date readings 00093 #define DS3234_REGISTER_BASE DS3234_REGISTER_SECONDS 00094 00095 // dayIntToStr -- convert day integer to the string 00096 static const char *dayIntToStr[7] { 00097 "Sunday", 00098 "Monday", 00099 "Tuesday", 00100 "Wednesday", 00101 "Thursday", 00102 "Friday", 00103 "Saturday" 00104 }; 00105 00106 // dayIntToChar -- convert day integer to character 00107 static const char dayIntToChar[7] = {'U', 'M', 'T', 'W', 'R', 'F', 'S' }; 00108 00109 class DS3234 00110 { 00111 public: 00112 00113 //////////////////// 00114 // Initialization // 00115 //////////////////// 00116 // Constructor -- Initialize class variables to 0 00117 DS3234(); 00118 // Begin -- Initialize SPI interface, and sets up the chip-select pin 00119 void begin(MicroBitPin* csPin, MicroBit* uBit); 00120 00121 /////////////////////// 00122 // Setting the Clock // 00123 /////////////////////// 00124 // setTime -- Set time and date/day registers of DS3234 00125 void setTime(uint8_t sec, uint8_t min, uint8_t hour, 00126 uint8_t day, uint8_t date, uint8_t month, uint8_t year); 00127 void setTime(uint8_t sec, uint8_t min, uint8_t hour12, bool pm, 00128 uint8_t day, uint8_t date, uint8_t month, uint8_t year); 00129 // setTime -- Set time and date/day registers of DS3234 (using data array) 00130 void setTime(uint8_t * time, uint8_t len); 00131 // autoTime -- Set time with compiler time/date 00132 bool autoTime(); 00133 00134 // To set specific values of the clock, use the set____ functions: 00135 void setSecond(uint8_t s); 00136 void setMinute(uint8_t m); 00137 void setHour(uint8_t h); 00138 void setDay(uint8_t d); 00139 void setDate(uint8_t d); 00140 void setMonth(uint8_t mo); 00141 void setYear(uint8_t y); 00142 00143 /////////////////////// 00144 // Reading the Clock // 00145 /////////////////////// 00146 // update -- Read all time/date registers and update the _time array 00147 void update(void); 00148 // update should be performed before any of the following. It will update 00149 // all values at one time. 00150 inline uint8_t second(void) { return BCDtoDEC(_time[TIME_SECONDS]); }; 00151 inline uint8_t minute(void) { return BCDtoDEC(_time[TIME_MINUTES]); }; 00152 inline uint8_t hour(void) { return BCDtoDEC(_time[TIME_HOURS]); }; 00153 inline uint8_t day(void) { return BCDtoDEC(_time[TIME_DAY]); }; 00154 inline const char dayChar(void) { return dayIntToChar[BCDtoDEC(_time[TIME_DAY]) - 1]; }; 00155 inline const char * dayStr(void) { return dayIntToStr[BCDtoDEC(_time[TIME_DAY]) - 1]; }; 00156 inline uint8_t date(void) { return BCDtoDEC(_time[TIME_DATE]); }; 00157 inline uint8_t month(void) { return BCDtoDEC(_time[TIME_MONTH]); }; 00158 inline uint8_t year(void) { return BCDtoDEC(_time[TIME_YEAR]); }; 00159 00160 // To read a single value at a time, use the get___ functions: 00161 uint8_t getSecond(void); 00162 uint8_t getMinute(void); 00163 uint8_t getHour(void); 00164 uint8_t getDay(void); 00165 uint8_t getDate(void); 00166 uint8_t getMonth(void); 00167 uint8_t getYear(void); 00168 00169 // is12Hour -- check if the DS3234 is in 12-hour mode | returns true if 12-hour mode 00170 bool is12Hour(void); 00171 // pm -- Check if 12-hour state is AM or PM | returns true if PM 00172 bool pm(void); 00173 00174 // DS3234 has a die-temperature reading. Value is produced in multiples of 0.25 deg C 00175 float temperature(void); 00176 00177 ///////////////////////////// 00178 // Alarm Setting Functions // 00179 ///////////////////////////// 00180 // Alarm 1 can be set to trigger on seconds, minutes, hours, and/or date/day. 00181 // Any of those can be masked out -- ignored for an alarm match. By setting 00182 // If a value is set to 255, the library will mask out that data. 00183 // By default the "date" value is the day-of-month (1-31) 00184 // The "day" boolean changes the "date" value to a day (between 1-7). 00185 void setAlarm1(uint8_t second = 255, uint8_t minute = 255, uint8_t hour = 255, uint8_t date = 255, bool day = false); 00186 // Second alarm 1 set function if the clock is in 12-hour mode: 00187 void setAlarm1(uint8_t second, uint8_t minute, uint8_t hour12, bool pm, uint8_t date = 255, bool day = false); 00188 // Alarm 2 functions similarly to alarm 1, but does not have a seconds value: 00189 void setAlarm2(uint8_t minute = 255, uint8_t hour = 255, uint8_t date = 255, bool day = false); 00190 // Second alarm 2 set function if the clock is in 12-hour mode: 00191 void setAlarm2(uint8_t minute, uint8_t hour12, bool pm, uint8_t date = 255, bool day = false); 00192 00193 // The SQW pin can be used as an interrupt. It is active-low, and can be 00194 // set to trigger on alarm 1 and/or alarm 2: 00195 void enableAlarmInterrupt(bool alarm1 = true, bool alarm2 = true); 00196 00197 // alarm1 and alarm2 check their respective flag in the control/status 00198 // register. They return true is the flag is set. 00199 // The "clear" boolean, commands the alarm function to clear the flag 00200 // (assuming it was set). 00201 bool alarm1(bool clear = true); 00202 bool alarm2(bool clear = true); 00203 00204 /////////////////////////////// 00205 // SQW Pin Control Functions // 00206 /////////////////////////////// 00207 void writeSQW(sqw_rate value); // Write SQW pin high, low, or to a set rate 00208 00209 ///////////////////////////// 00210 // Misc. Control Functions // 00211 ///////////////////////////// 00212 void enable(void); // Enable the oscillator 00213 void disable(void); // Disable the oscillator (no counting!) 00214 00215 void set12Hour(bool enable12 = true); // Enable/disable 12-hour mode 00216 void set24Hour(bool enable24 = true); // Enable/disable 24-hour mode 00217 00218 void writeToSRAM(uint8_t address, uint8_t data); 00219 uint8_t readFromSRAM(uint8_t address); 00220 00221 private: 00222 MicroBitPin* _csPin; 00223 MicroBit* _uBit; 00224 uint8_t _time[TIME_ARRAY_LENGTH]; 00225 bool _pm; 00226 00227 uint8_t BCDtoDEC(uint8_t val); 00228 uint8_t DECtoBCD(uint8_t val); 00229 00230 void spiWriteBytes(DS3234_registers reg, uint8_t * values, uint8_t len); 00231 void spiWriteByte(DS3234_registers reg, uint8_t value); 00232 uint8_t spiReadByte(DS3234_registers reg); 00233 void spiReadBytes(DS3234_registers reg, uint8_t * dest, uint8_t len); 00234 }; 00235 00236 extern DS3234 rtc; 00237 00238 #endif // SPARKFUNDS3234RTC_H
Generated on Wed Jul 20 2022 07:11:22 by
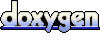