
4180 Lab ????
Dependencies: 4DGL-uLCD-SE SDFileSystem ShiftBrite mbed-rtos mbed wave_player
Fork of rtos_basic by
main.cpp
00001 #include "mbed.h" 00002 #include "rtos.h" 00003 #include "uLCD_4DGL.h" 00004 #include "ShiftBrite.h" 00005 #include "SDFileSystem.h" 00006 #include "wave_player.h" 00007 00008 Mutex mutex; //handles writing to the LCD 00009 Mutex mutex2; //used for color changing 00010 DigitalOut led1(LED1); 00011 DigitalOut led2(LED2); 00012 DigitalOut led3(LED3); 00013 DigitalOut led4(LED4); 00014 Thread thread1; 00015 Thread thread2; 00016 Thread thread3; 00017 Thread thread4; 00018 DigitalOut latch(p15); 00019 DigitalOut enable(p16); 00020 SPI spi(p11, p12, p13); 00021 //uLCD_4DGL uLCD(p28,p27,p29); //(p27, p28, p30); //tx, rx, rst 00022 uLCD_4DGL uLCD(p28, p27, p30); 00023 ShiftBrite myBrite(p15,p16,spi); //latch, enable, spi 00024 SDFileSystem sd(p5, p6, p7, p8, "sd"); //SD card 00025 AnalogOut DACout(p18); //must be p18 00026 RawSerial BT(p9, p10); //bluetooth pinout 00027 int red = 200; 00028 int blue = 0; 00029 int green = 0; 00030 FILE *wave_file = NULL; //global bc its gotta be changed by Main while running in child thread 00031 unsigned int redHex = 0xFF0000; 00032 unsigned int grnHex = 0x00FF00; 00033 unsigned int bluHex = 0x0000FF; 00034 00035 00036 wave_player waver(&DACout); 00037 00038 void LCD_thread1() { 00039 while(1){ 00040 mutex.lock(); 00041 uLCD.filled_circle(64, 64, 12, 0xFF0000); 00042 mutex.unlock(); 00043 wait(.5); 00044 mutex.lock(); 00045 uLCD.filled_circle(64, 64, 12, bluHex); 00046 mutex.unlock(); 00047 wait(.5); 00048 } 00049 } 00050 void LCD_thread2() { //update a timer on the display every 100ms 00051 uLCD.cls(); 00052 //float time = 0.0; 00053 int count =0; 00054 while(1) { 00055 Thread::wait(60); 00056 //time = time + 0.25; 00057 mutex.lock(); 00058 uLCD.locate(0,0); 00059 count++; 00060 uLCD.printf("Counting! %i \n", count); 00061 mutex.unlock(); 00062 } 00063 } 00064 void LED_thread() { 00065 //flash red & blue for police siren 00066 while(1){ 00067 mutex2.lock(); 00068 //myBrite.Write(red,green,blue); 00069 myBrite.Write(150, green, 0); 00070 mutex2.unlock(); 00071 wait(.5); 00072 mutex2.lock(); 00073 myBrite.Write(0,green,150); 00074 //myBrite.Write(blue,green,red); // change LEDs so Red=OFF, Blue=ON 00075 mutex2.unlock(); 00076 wait(.5); 00077 } 00078 } 00079 /* 00080 void BT_thread() { 00081 // use mutex to lock getc(), printf(), scanf() 00082 // don't unlock until you've checked that it's readable() 00083 char bnum=0; 00084 while(1) { 00085 mutex.lock(); 00086 if (BT.getc()=='!') { 00087 if (BT.getc()=='B') { //button data 00088 bnum = BT.getc(); //button number 00089 if (bnum == '1') { 00090 green = 250; 00091 } 00092 if (bnum == '2') { 00093 00094 } 00095 if (bnum == '3') { 00096 green = 250; 00097 } 00098 if (bnum == '4') { 00099 green = 0; 00100 } 00101 //if ((bnum>='1')&&(bnum<='4')) //is a number button 1..4 00102 // myled[bnum-'1']=blue.getc()-'0'; //turn on/off that num LED 00103 } 00104 } 00105 mutex.unlock(); 00106 } 00107 }*/ 00108 void sound_thread(){ 00109 //FILE *wave_file; 00110 wave_file=fopen("/sd/Police_Siren.wav","r"); 00111 if (wave_file == NULL){ 00112 led1=led2=led3=led4 = 1; 00113 } 00114 waver.play(wave_file); 00115 fclose(wave_file); 00116 } 00117 int main() { 00118 thread1.start(LED_thread); //police lights work 00119 thread2.start(LCD_thread1); 00120 thread3.start(LCD_thread2); 00121 thread4.start(sound_thread); 00122 // use mutex to lock getc(), printf(), scanf() 00123 // don't unlock until you've checked that it's readable() 00124 00125 char bnum=0; 00126 // 00127 while(1) { 00128 //mutex.lock(); 00129 led3=0; 00130 led4=1; 00131 if (BT.getc()=='!') { 00132 if (BT.getc()=='B') { //button data 00133 bnum = BT.getc(); //button number 00134 mutex.unlock(); 00135 if (bnum == '1') { //turn Green LED on 00136 green = 250; 00137 led1 = 1; 00138 led2=led3=led4=0; 00139 } 00140 if (bnum == '2') { // revert to normal operation 00141 green = 0; 00142 bluHex = 0x0000FF; 00143 led1=led3=led4=0; 00144 led2=1; 00145 } 00146 if (bnum == '3') { // change sound file playing 00147 led2=led1=led4=0; 00148 led3=1; 00149 if ( wave_file != NULL) { 00150 led2=1; // debug 00151 //fclose(wave_file); //stop police siren from playing 00152 thread4.terminate(); 00153 led2=0; 00154 } 00155 FILE *wave_file2; 00156 wave_file2=fopen("/sd/banker_calling.wav","r"); 00157 if (wave_file2 == NULL){ 00158 led1=led2=0; 00159 led3=led4=1; 00160 } 00161 waver.play(wave_file2); 00162 fclose(wave_file2); 00163 thread4.start(sound_thread); 00164 //wave_file=fopen("/sd/Police_Siren.wav","r"); 00165 //waver.play(wave_file); 00166 } 00167 if (bnum == '4') { // change LCD colors 00168 bluHex = 0x00FF00; //change the lights to flash red/grn 00169 led2=led3=led1=0; 00170 led4=1; 00171 } 00172 } 00173 //mutex.unlock(); 00174 led4=0; 00175 led3=1; 00176 } 00177 //mutex.unlock(); 00178 } 00179 00180 }
Generated on Wed Jul 20 2022 16:46:13 by
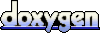