
Upper Version Add PUT method Delete POST method
Dependencies: Adafruit_GFX MbedJSONValue_v102 WIZnetInterface mbed
Fork of WIZwiki-REST-io_v102 by
main.cpp
00001 #include "mbed.h" 00002 #include "HTTPServer.h" 00003 #include "RequestHandler.h" 00004 #include "EthernetInterface.h" 00005 #include "MbedJSONValue.h" 00006 00007 #define SERVER_PORT 80 00008 //#define DHCP 00009 //#define DEBUG 00010 00011 //-- I2C OLED -- 00012 #include "Adafruit_SSD1306.h" 00013 00014 class I2CPreInit : public I2C 00015 { 00016 public: 00017 I2CPreInit(PinName sda, PinName scl) : I2C(sda, scl) 00018 { 00019 frequency(100000); 00020 start(); 00021 }; 00022 }; 00023 00024 //-- I2C OLED -- 00025 I2CPreInit gI2C(PA_10,PA_9); 00026 Adafruit_SSD1306_I2c gOled(gI2C,NC,0x78,64,128); 00027 00028 //-- PWM DC -- 00029 PwmOut DC(D6); 00030 00031 //-- GPIO LED -- 00032 DigitalInOut GP05(D5); 00033 00034 DigitalInOut GP04(D4); 00035 DigitalInOut GP03(D3); 00036 00037 00038 //-- ADC -- 00039 AnalogIn ain(A0); 00040 00041 EthernetInterface eth; 00042 HTTPServer WIZwikiWebSvr; 00043 MbedJSONValue WIZwikiREST; 00044 00045 // Enter a MAC address for your controller below. 00046 uint8_t mac_addr[6] = {0x00, 0x08, 0xDC, 0x00, 0x01, 0xFE}; 00047 char mac_str[20]; 00048 char ip_addr[] = "192.168.100.100"; 00049 char subnet_mask[] = "255.255.255.0"; 00050 char gateway_addr[] = "192.168.100.1"; 00051 float ain_value; 00052 00053 GetRequestHandler myGetReq; 00054 //PostRequestHandler myPostReq; 00055 PutRequestHandler myPutReq; 00056 00057 //-- I2C OLED -- 00058 bool oled_set(void* param) 00059 { 00060 char * oled; 00061 if(!param) return false; 00062 oled = (char*) param; 00063 gOled.clearDisplay(); 00064 gOled.setTextCursor(0,0); 00065 gOled.printf("%s",oled); 00066 gOled.display(); 00067 return true; 00068 } 00069 //-- PWM DC -- 00070 bool pwm_set(void* param) 00071 { 00072 if(!param) return false; 00073 DC.write((float)(*(int*)param)/100.0); 00074 return true; 00075 } 00076 //-- GPIO -- 00077 bool p5_set(void* param) 00078 { 00079 if(!param) return false; 00080 GP05.write(*(int*)param); 00081 return true; 00082 } 00083 bool p4_set(void* param) 00084 { 00085 if(!param) return false; 00086 GP04.write(*(int*)param); 00087 return true; 00088 } 00089 bool p3_set(void* param) 00090 { 00091 if(!param) return false; 00092 GP03.write(*(int*)param); 00093 return true; 00094 } 00095 00096 //-- ADC -- 00097 bool ain_read(void* param) 00098 { 00099 ((MbedJSONValue*)param)->_value.asDouble = ain.read(); 00100 return true; 00101 } 00102 00103 void debug_info() 00104 { 00105 printf("SP:0x%X\r\n",__current_sp()); 00106 __heapstats((__heapprt)fprintf,stderr); 00107 #ifdef DEBUG 00108 __heapvalid((__heapprt)fprintf,stderr, 1); 00109 #endif 00110 printf("\r\n"); 00111 } 00112 00113 void WIZwiki_REST_init(); 00114 00115 int main(void) 00116 { 00117 #ifdef DEBUG 00118 debug_info(); 00119 #endif 00120 sprintf(mac_str, "%02X:%02X:%02X:%02X:%02X:%02X",mac_addr[0],mac_addr[1],mac_addr[2],mac_addr[3],mac_addr[4],mac_addr[5]); 00121 00122 // OLED init 00123 gOled.begin(); 00124 gOled.clearDisplay(); 00125 00126 // PWM init 00127 DC.period_ms(1); 00128 DC.write(0); 00129 00130 //GPIO set & init 00131 GP05.output(); 00132 GP05.write(1); 00133 00134 //ADC init 00135 00136 printf("START \r\n"); 00137 printf("sizeof(MbedJSONValue)=%d\r\n",sizeof(MbedJSONValue)); 00138 printf("sizeof(vector)=%d\r\n",sizeof(std::vector<string*>)); 00139 printf("sizeof(string)=%d\r\n",sizeof(std::string)); 00140 #ifdef DEBUG 00141 debug_info(); 00142 #endif 00143 00144 WIZwiki_REST_init(); 00145 00146 #ifdef DEBUG 00147 debug_info(); 00148 #endif 00149 00150 // Serialize it into a JSON string 00151 printf("---------------------WIZwikiREST-------------------- \r\n"); 00152 printf("\r\n%s\r\n", WIZwikiREST.serialize().c_str()); 00153 printf("---------------------------------------------------- \r\n"); 00154 00155 WIZwikiWebSvr.add_request_handler("GET", &myGetReq); 00156 //WIZwikiWebSvr.add_request_handler("POST", &myPostReq); 00157 WIZwikiWebSvr.add_request_handler("PUT", &myPutReq); 00158 //WIZwikiWebSvr.add_request_handler("DELETE", new PostRequestHandler()); 00159 00160 #ifdef DHCP 00161 eth.init(mac_addr); //Use DHCP 00162 #else 00163 eth.init(mac_addr, ip_addr, subnet_mask, gateway_addr); //Not Use DHCP 00164 #endif 00165 00166 00167 printf("Check Ethernet Link\r\n"); 00168 00169 do{ 00170 printf(" Link - Wait... \r\n"); 00171 wait(1); 00172 }while(!eth.ethernet_link()); 00173 printf("-- Ethetnet PHY Link - Done -- \r\n"); 00174 00175 if (eth.connect() < 0 ) 00176 printf("-- EThernet Connect - Fail -- \r\n"); 00177 else 00178 { 00179 printf("-- Assigned Network Information -- \r\n"); 00180 printf(" IP : %s\r\n\r\n", eth.getIPAddress()); 00181 printf(" MASK : %s\r\n\r\n", eth.getNetworkMask()); 00182 printf(" GW : %s\r\n\r\n", eth.getGateway()); 00183 } 00184 00185 printf("Link up\r\n"); 00186 printf("IP Address is %s\r\n", eth.getIPAddress()); 00187 #ifdef DEBUG 00188 debug_info(); 00189 #endif 00190 00191 if(!WIZwikiWebSvr.init(SERVER_PORT)) 00192 { 00193 eth.disconnect(); 00194 return -1; 00195 } 00196 00197 while(1) 00198 { 00199 WIZwikiWebSvr.run(); 00200 } 00201 } 00202 00203 void WIZwiki_REST_init(void) 00204 { 00205 //Fill the object 00206 WIZwikiREST["Name"] = "WIZwikiREST-io ver1.01"; 00207 WIZwikiREST["Name"].accessible = false; 00208 #ifdef DEBUG 00209 debug_info(); 00210 #endif 00211 00212 //Network 00213 WIZwikiREST["Network"]["MAC"] = mac_str; 00214 WIZwikiREST["Network"]["IP"] = ip_addr; 00215 WIZwikiREST["Network"]["IP"].accessible = true; 00216 WIZwikiREST["Network"]["SN"] = subnet_mask; 00217 WIZwikiREST["Network"]["SN"].accessible = true; 00218 WIZwikiREST["Network"]["GW"] = gateway_addr; 00219 WIZwikiREST["Network"]["GW"].accessible = true; 00220 00221 // I2C OLED 00222 WIZwikiREST["I2C"]["OLED"] = "none"; 00223 WIZwikiREST["I2C"]["OLED"].accessible = true; 00224 WIZwikiREST["I2C"]["OLED"].cb_action = oled_set; 00225 00226 // PWM DC 00227 WIZwikiREST["PWM"]["DC"] = DC.read(); 00228 WIZwikiREST["PWM"]["DC"].accessible = true; 00229 WIZwikiREST["PWM"]["DC"].cb_action = pwm_set; 00230 00231 // GPIO 00232 WIZwikiREST["GPIOs"]["P03"] = GP03.read(); 00233 WIZwikiREST["GPIOs"]["P03"].accessible = true; 00234 WIZwikiREST["GPIOs"]["P03"].cb_action = p3_set; 00235 WIZwikiREST["GPIOs"]["P04"] = GP04.read(); 00236 WIZwikiREST["GPIOs"]["P04"].accessible = true; 00237 WIZwikiREST["GPIOs"]["P04"].cb_action = p4_set;\ 00238 WIZwikiREST["GPIOs"]["P05"] = GP05.read(); 00239 WIZwikiREST["GPIOs"]["P05"].accessible = true; 00240 WIZwikiREST["GPIOs"]["P05"].cb_action = p5_set; 00241 00242 // ADC 00243 WIZwikiREST["ADC"]["A0"] = ain.read(); 00244 WIZwikiREST["ADC"]["A0"].accessible = false; 00245 WIZwikiREST["ADC"]["A0"].cb_action = ain_read; 00246 00247 #ifdef DEBUG 00248 debug_info(); 00249 #endif 00250 }
Generated on Sat Jul 16 2022 05:32:29 by
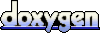