
Upper Version Add PUT method Delete POST method
Dependencies: Adafruit_GFX MbedJSONValue_v102 WIZnetInterface mbed
Fork of WIZwiki-REST-io_v102 by
HTTPServer.cpp
00001 #include <string.h> 00002 #include "HTTPServer.h" 00003 #include "MbedJSONValue.h" 00004 00005 extern MbedJSONValue WIZwikiREST; 00006 00007 bool cmp(char* a, char* b) 00008 { 00009 return strcmp(a,b) < 0; 00010 } 00011 00012 HTTPServer::HTTPServer(): 00013 socket(), 00014 handlers(&cmp) 00015 { 00016 } 00017 00018 HTTPServer::~HTTPServer() 00019 { 00020 for(std::map<char*, RequestHandler*>::iterator itor = handlers.begin(); 00021 itor != handlers.end(); 00022 ++itor) 00023 delete itor->second; 00024 } 00025 00026 bool HTTPServer::init(int port) 00027 { 00028 socket.set_blocking(true); 00029 if(socket.bind(port)) 00030 { 00031 printf("Could not bind on port %d.\n", port); 00032 return false; 00033 } 00034 00035 if(socket.listen()) 00036 { 00037 printf("Could not listen %d\n", port); 00038 return false; 00039 } 00040 00041 return true; 00042 } 00043 00044 void HTTPServer::run() 00045 { 00046 TCPSocketConnection c; 00047 while(true) 00048 { 00049 while(socket.accept(c)); 00050 c.set_blocking(false, 1000); 00051 while(c.is_connected()) 00052 { 00053 00054 int n = c.receive_all(HTTPBUF, sizeof(HTTPBUF)-1); 00055 00056 if(n == 0) 00057 { 00058 c.close(); 00059 break; 00060 } 00061 else if(n != -1) 00062 { 00063 #ifdef DEBUG_HTTP 00064 printf("Received data : %d\r\n",n); 00065 #endif 00066 00067 HTTPBUF[n] = '\0'; 00068 if(handle_request(HTTPBUF) == HTTP_SUCCESS) 00069 { 00070 c.send(rest_result, strlen(rest_result)); 00071 //c.send((char*)rest_result.c_str(), 159); 00072 } 00073 else 00074 { 00075 //printf("send fail : %s\r\n",(char*)rest_result.c_str()); 00076 c.send(rest_result, strlen(rest_result)); 00077 } 00078 } 00079 else 00080 printf("Error while receiving data\n"); 00081 00082 } 00083 } 00084 } 00085 00086 00087 HTTP_RESULT HTTPServer::handle_request(char *buffer) 00088 { 00089 char *request_type; 00090 char *request; 00091 00092 //{"Name":"WIZwiki-REST-01","Network":{"IP":"192.168.100.100","SN":"255.255.255.0","GW":"192.168.100.1"},"User":{"Name":"Lawrence","ID":"law","PSWD":"law1234"}} 159 00093 if(buffer) 00094 { 00095 #ifdef DEBUG_HTTP 00096 // buffer check 00097 printf("***********************\r\n"); 00098 printf(" buffer=%s \r\n",buffer); 00099 printf("***********************\r\n"); 00100 #endif 00101 // type parsing 00102 request_type = strtok(buffer," \r\n"); 00103 #ifdef DEBUG_HTTP 00104 printf("Type = %s\r\n", request_type); 00105 #endif 00106 00107 if(request_type) 00108 { 00109 request = strtok(NULL, " \r\n"); // corrested " " -> " /" : /Name -> Name 00110 if(request) 00111 { 00112 #ifdef DEBUG_HTTP 00113 printf("URI = %s\r\n", request); 00114 #endif 00115 } 00116 else 00117 { 00118 strcpy(rest_result, "Invaild URI"); 00119 #ifdef DEBUG_HTTP 00120 printf("%s\r\n",rest_result); 00121 #endif 00122 return HTTP_INVALID_URI; 00123 } 00124 } 00125 } 00126 00127 std::map<char*, RequestHandler*>::iterator itor = handlers.find(request_type); 00128 if(itor == handlers.end()) 00129 { 00130 strcpy(rest_result, "No request handler found for this type of request."); 00131 return HTTP_INVALID_HANDLE; 00132 } 00133 //if(itor != NULL) 00134 //itor->handle(request, rest_result.c_str()); 00135 if(itor->second != NULL) 00136 { 00137 char* request_data = 0; 00138 #ifdef HTTP_POST 00139 if(!strcmp(request_type,"POST")) 00140 { 00141 request_data = strstr(request+strlen(request)+1, "\r\n\r\n"); 00142 #ifdef DEBUG_HTTP 00143 printf("POST:request_data=%s\r\n",request_data+4); 00144 #endif 00145 } 00146 #endif 00147 if(!strcmp(request_type,"PUT")) 00148 { 00149 request_data = strstr(request+strlen(request)+1, "\r\n\r\n"); 00150 #ifdef DEBUG_HTTP 00151 printf("PUT:request_data=%s\r\n",request_data+4); 00152 #endif 00153 } 00154 itor->second->handle(request, request_data, rest_result); 00155 } 00156 else 00157 { 00158 strcpy(rest_result, "Invalid request handler"); 00159 return HTTP_INVALID_HANDLE; 00160 } 00161 return HTTP_SUCCESS; 00162 } 00163 00164 void HTTPServer::add_request_handler(char *name, RequestHandler* handler) 00165 { 00166 handlers[name] = handler; 00167 } 00168
Generated on Sat Jul 16 2022 05:32:29 by
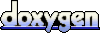