Changes to support running on smaller memory LPC device LPC1764
Fork of mbed-dev by
AnalogOut.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #ifndef MBED_ANALOGOUT_H 00017 #define MBED_ANALOGOUT_H 00018 00019 #include "platform.h" 00020 00021 #if DEVICE_ANALOGOUT 00022 00023 #include "analogout_api.h" 00024 #include "PlatformMutex.h" 00025 00026 namespace mbed { 00027 00028 /** An analog output, used for setting the voltage on a pin 00029 * 00030 * @Note Synchronization level: Thread safe 00031 * 00032 * Example: 00033 * @code 00034 * // Make a sawtooth output 00035 * 00036 * #include "mbed.h" 00037 * 00038 * AnalogOut tri(p18); 00039 * int main() { 00040 * while(1) { 00041 * tri = tri + 0.01; 00042 * wait_us(1); 00043 * if(tri == 1) { 00044 * tri = 0; 00045 * } 00046 * } 00047 * } 00048 * @endcode 00049 */ 00050 class AnalogOut { 00051 00052 public: 00053 00054 /** Create an AnalogOut connected to the specified pin 00055 * 00056 * @param AnalogOut pin to connect to (18) 00057 */ 00058 AnalogOut(PinName pin) { 00059 analogout_init(&_dac, pin); 00060 } 00061 00062 /** Set the output voltage, specified as a percentage (float) 00063 * 00064 * @param value A floating-point value representing the output voltage, 00065 * specified as a percentage. The value should lie between 00066 * 0.0f (representing 0v / 0%) and 1.0f (representing 3.3v / 100%). 00067 * Values outside this range will be saturated to 0.0f or 1.0f. 00068 */ 00069 void write(float value) { 00070 lock(); 00071 analogout_write(&_dac, value); 00072 unlock(); 00073 } 00074 00075 /** Set the output voltage, represented as an unsigned short in the range [0x0, 0xFFFF] 00076 * 00077 * @param value 16-bit unsigned short representing the output voltage, 00078 * normalised to a 16-bit value (0x0000 = 0v, 0xFFFF = 3.3v) 00079 */ 00080 void write_u16(unsigned short value) { 00081 lock(); 00082 analogout_write_u16(&_dac, value); 00083 unlock(); 00084 } 00085 00086 /** Return the current output voltage setting, measured as a percentage (float) 00087 * 00088 * @returns 00089 * A floating-point value representing the current voltage being output on the pin, 00090 * measured as a percentage. The returned value will lie between 00091 * 0.0f (representing 0v / 0%) and 1.0f (representing 3.3v / 100%). 00092 * 00093 * @note 00094 * This value may not match exactly the value set by a previous write(). 00095 */ 00096 float read() { 00097 lock(); 00098 float ret = analogout_read(&_dac); 00099 unlock(); 00100 return ret; 00101 } 00102 00103 /** An operator shorthand for write() 00104 */ 00105 AnalogOut& operator= (float percent) { 00106 // Underlying write call is thread safe 00107 write(percent); 00108 return *this; 00109 } 00110 00111 AnalogOut& operator= (AnalogOut& rhs) { 00112 // Underlying write call is thread safe 00113 write(rhs.read()); 00114 return *this; 00115 } 00116 00117 /** An operator shorthand for read() 00118 */ 00119 operator float() { 00120 // Underlying read call is thread safe 00121 return read(); 00122 } 00123 00124 virtual ~AnalogOut() { 00125 // Do nothing 00126 } 00127 00128 protected: 00129 00130 virtual void lock() { 00131 _mutex.lock(); 00132 } 00133 00134 virtual void unlock() { 00135 _mutex.unlock(); 00136 } 00137 00138 dac_t _dac; 00139 PlatformMutex _mutex; 00140 }; 00141 00142 } // namespace mbed 00143 00144 #endif 00145 00146 #endif
Generated on Tue Jul 12 2022 15:56:58 by
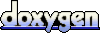