initial release
Embed:
(wiki syntax)
Show/hide line numbers
XNucleo53L1A1.h
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file XNucleo53L1A1.h 00004 * @author JS 00005 * @version V0.0.1 00006 * @date 15-January-2019 00007 * @brief Header file for component XNucleo53L1A1 00008 ****************************************************************************** 00009 * @attention 00010 * 00011 * <h2><center>© COPYRIGHT(c) 2019 STMicroelectronics</center></h2> 00012 * 00013 * Redistribution and use in source and binary forms, with or without modification, 00014 * are permitted provided that the following conditions are met: 00015 * 1. Redistributions of source code must retain the above copyright notice, 00016 * this list of conditions and the following disclaimer. 00017 * 2. Redistributions in binary form must reproduce the above copyright notice, 00018 * this list of conditions and the following disclaimer in the documentation 00019 * and/or other materials provided with the distribution. 00020 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00021 * may be used to endorse or promote products derived from this software 00022 * without specific prior written permission. 00023 * 00024 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00025 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00026 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00027 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00028 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00029 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00030 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00031 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00032 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00033 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 * 00035 ****************************************************************************** 00036 */ 00037 00038 #ifndef __VL53L1_X_NUCLEO_CLASS_H 00039 #define __VL53L1_X_NUCLEO_CLASS_H 00040 00041 00042 /* Includes ------------------------------------------------------------------*/ 00043 #include "VL53L1X_Class.h" 00044 #include "Stmpe1600.h" 00045 //#include "DevI2C.h" 00046 #include "VL53L1X_I2C.h" 00047 00048 00049 /** New device addresses */ 00050 //#define NEW_SENSOR_CENTRE_ADDRESS 0x52 00051 #define NEW_SENSOR_CENTRE_ADDRESS 0x54 00052 #define NEW_SENSOR_LEFT_ADDRESS 0x56 00053 #define NEW_SENSOR_RIGHT_ADDRESS 0x58 00054 00055 00056 /* Classes--------------------------------------------------------------------*/ 00057 00058 /* Classes -------------------------------------------------------------------*/ 00059 /** Class representing the X-NUCLEO-VL53L1A1 expansion board 00060 */ 00061 class XNucleo53L1A1 00062 { 00063 public: 00064 /** Constructor 1 00065 * @param[in] &i2c device I2C to be used for communication 00066 */ 00067 XNucleo53L1A1(VL53L1X_DevI2C *ext_i2c) : dev_i2c(ext_i2c) 00068 { 00069 stmpe1600_exp0 = new Stmpe1600((DevI2C *)ext_i2c, (0x43 * 2)); // U21 00070 00071 stmpe1600_exp1 = new Stmpe1600((DevI2C *)ext_i2c, (0x42 * 2)); // U19 00072 00073 xshutdown_centre = new Stmpe1600DigiOut((DevI2C *)dev_i2c, GPIO_15, (0x42 * 2)); // U19 on schematic 00074 sensor_centre = new VL53L1X(dev_i2c, xshutdown_centre, A2); 00075 00076 xshutdown_left = new Stmpe1600DigiOut((DevI2C *)dev_i2c, GPIO_14, (0x43 * 2)); // U21 on schematic 00077 sensor_left = new VL53L1X(dev_i2c, xshutdown_left, D8); 00078 00079 xshutdown_right = new Stmpe1600DigiOut((DevI2C *)dev_i2c, GPIO_15, (0x43 * 2)); // U21 on schematic 00080 sensor_right = new VL53L1X(dev_i2c, xshutdown_right, D2); 00081 } 00082 00083 /** Constructor 2 00084 * @param[in] &i2c device I2C to be used for communication 00085 * @param[in] PinName gpio1_top Mbed DigitalOut pin name to be used as a top sensor GPIO_1 INT 00086 * @param[in] PinName gpio1_bottom Mbed DigitalOut pin name to be used as a bottom sensor GPIO_1 INT 00087 * @param[in] PinName gpio1_left Mbed DigitalOut pin name to be used as a left sensor GPIO_1 INT 00088 * @param[in] PinName gpio1_right Mbed DigitalOut pin name to be used as a right sensor GPIO_1 INT 00089 */ 00090 XNucleo53L1A1(VL53L1X_DevI2C *ext_i2c, PinName gpio1_centre, 00091 PinName gpio1_left, PinName gpio1_right) : dev_i2c(ext_i2c) 00092 { 00093 stmpe1600_exp0 = new Stmpe1600((DevI2C *)ext_i2c, (0x43 * 2)); // U21 00094 00095 stmpe1600_exp1 = new Stmpe1600((DevI2C *)ext_i2c, (0x42 * 2)); // U19 00096 00097 xshutdown_centre = new Stmpe1600DigiOut((DevI2C *)dev_i2c, GPIO_15, (0x42 * 2)); // U19 on schematic 00098 sensor_centre = new VL53L1X(dev_i2c, xshutdown_centre, gpio1_centre); 00099 00100 xshutdown_left = new Stmpe1600DigiOut((DevI2C *)dev_i2c, GPIO_14, (0x43 * 2)); // U21 on schematic 00101 sensor_left = new VL53L1X(dev_i2c, xshutdown_left, gpio1_left); 00102 00103 xshutdown_right = new Stmpe1600DigiOut((DevI2C *)dev_i2c, GPIO_15, (0x43 * 2)); // U21 on schematic 00104 sensor_right = new VL53L1X(dev_i2c, xshutdown_right, gpio1_right); 00105 } 00106 00107 00108 /** Destructor 00109 */ 00110 ~XNucleo53L1A1() 00111 { 00112 if (xshutdown_centre != NULL) { 00113 delete xshutdown_centre; 00114 xshutdown_centre = NULL; 00115 } 00116 if (sensor_centre != NULL) { 00117 delete sensor_centre; 00118 sensor_centre = NULL; 00119 } 00120 if (xshutdown_left != NULL) { 00121 delete xshutdown_left; 00122 xshutdown_left = NULL; 00123 } 00124 if (sensor_left != NULL) { 00125 delete sensor_left; 00126 sensor_left = NULL; 00127 } 00128 if (xshutdown_right != NULL) { 00129 delete xshutdown_right; 00130 xshutdown_right = NULL; 00131 } 00132 if (sensor_right != NULL) { 00133 delete sensor_right; 00134 sensor_right = NULL; 00135 } 00136 00137 delete stmpe1600_exp0; 00138 stmpe1600_exp0 = NULL; 00139 delete stmpe1600_exp1; 00140 stmpe1600_exp1 = NULL; 00141 _instance = NULL; 00142 } 00143 00144 00145 /** 00146 * @brief Creates a singleton object instance 00147 * @param[in] &i2c device I2C to be used for communication 00148 * @return Pointer to the object instance 00149 */ 00150 static XNucleo53L1A1 *instance(VL53L1X_DevI2C *ext_i2c); 00151 00152 /** 00153 * @brief Creates a singleton object instance 00154 * @param[in] &i2c device I2C to be used for communication 00155 * @param[in] PinName gpio1_centre the pin connected to top sensor INT 00156 * @param[in] PinName gpio1_left the pin connected to left sensor INT 00157 * @param[in] PinName gpio1_right the pin connected to right sensor INT 00158 * @return Pointer to the object instance 00159 */ 00160 static XNucleo53L1A1 *instance(VL53L1X_DevI2C *ext_i2c, PinName gpio1_centre, 00161 PinName gpio1_left, PinName gpio1_right); 00162 00163 /** 00164 * @brief Initialize the board and sensors with deft values 00165 * @return 0 on success 00166 */ 00167 int init_board(); 00168 00169 VL53L1X_DevI2C *dev_i2c; 00170 VL53L1X *sensor_centre; 00171 VL53L1X *sensor_left; 00172 VL53L1X *sensor_right; 00173 Stmpe1600 *stmpe1600_exp0; 00174 Stmpe1600 *stmpe1600_exp1; 00175 Stmpe1600DigiOut *xshutdown_centre; 00176 Stmpe1600DigiOut *xshutdown_left; 00177 Stmpe1600DigiOut *xshutdown_right; 00178 00179 private: 00180 static XNucleo53L1A1 *_instance; 00181 }; 00182 00183 00184 #endif /* __VL53L1_X_NUCLEO_CLASS_H */
Generated on Thu Jul 14 2022 03:53:35 by
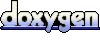