
Sample project to connect to AT&T M2X from the STM32 Nucleo + MTS Cellular SocketModem shield
Dependencies: M2XStreamClient jsonlite mbed
Fork of MTSAS_Cellular_Connect_Example_F411 by
main.cpp
00001 #include <jsonlite.h> 00002 00003 #include "mbed.h" 00004 #include "mtsas.h" 00005 #include "M2XStreamClient.h" 00006 00007 const char m2xKey[] = "06ce9a9febbfc50ffceb0d8214427767"; // Replace with your M2X API key 00008 const char feedId[] = "48c77c779f0eed1243e623190e588501"; // Replace with your blueprint Feed ID 00009 const char streamName[] = "temperature"; // Replace with your stream name 00010 00011 char name[] = "austin"; // Name of current location of datasource 00012 double latitude = 30.3748076; 00013 double longitude = -97.7386896; // You can also read those values from a GPS 00014 double elevation = 400.00; 00015 00016 // Note: D8, D2, D3, D6, D4, D7 are being used by MTSAS Cellular Modem 00017 00018 PwmOut mypwm(D13); // This is the Nucleo board LED2 00019 AnalogIn temp_sensor(A1); // There's also a conflict with A0, so connect temp_sensor A1 00020 00021 int main() 00022 { 00023 int ping_status = 0; 00024 char amb_temp[6]; 00025 int response; 00026 int adc_scale = 65536; //4096; 00027 int B = 3975; 00028 float resistance; 00029 float temperature; 00030 float temperature_f; 00031 00032 mypwm.period_ms(500); 00033 mypwm.pulsewidth_ms(250); 00034 00035 //Modify to match your apn if you are using an HSPA radio with a SIM card 00036 const char APN[] = "epc.tmobile.com"; 00037 printf("Starting....\n"); 00038 //Sets the log level to INFO, higher log levels produce more log output. 00039 //Possible levels: NONE, FATAL, ERROR, WARNING, INFO, DEBUG, TRACE 00040 MTSLog::setLogLevel(MTSLog::TRACE_LEVEL); 00041 00042 /** STMicro Nucelo F401RE 00043 * The supported jumper configurations of the MTSAS do not line up with 00044 * the pin mapping of the Nucleo F401RE. Therefore, the MTSAS serial TX 00045 * pin (JP8 Pin 2) must be manually jumped to Serial1 RX (Shield pin D2) 00046 * and the MTSAS serial RX pin (JP9 Pin 2) pin must be manually jumped to 00047 * Serial1 TX (Shield pin D8). 00048 * Uncomment the following line to use the STMicro Nuceleo F401RE 00049 */ 00050 MTSSerialFlowControl* io = new MTSSerialFlowControl(D8, D2, D3, D6); 00051 printf("FlowControl...\n"); 00052 /** Freescale KL46Z 00053 * To configure the serial pins for the Freescale KL46Z board, use MTSAS jumper 00054 * configuration B. Uncomment the following line to use the Freescale KL46Z board 00055 */ 00056 //MTSSerialFlowControl* io = new MTSSerialFlowControl(D2, D9, D3, D6); 00057 00058 /** Freescale K64F 00059 * To configure the serial pins for the Freescale K64F board, use MTSAS jumper 00060 * configuration A. Uncomment the following line to use the Freescale K64F board 00061 */ 00062 //MTSSerialFlowControl* io = new MTSSerialFlowControl(D1, D0, D3, D6); 00063 00064 //Sets the baud rate for communicating with the radio 00065 io->baud(115200); 00066 printf("Baud...\n"); 00067 //Create radio object 00068 Cellular* radio = CellularFactory::create(io); 00069 printf("CellularFactory...\n"); 00070 if (! radio) { 00071 logFatal("Failed to initialize radio"); 00072 return 1; 00073 } 00074 radio->configureSignals(D4,D7,RESET); 00075 Transport::setTransport(radio); 00076 printf("setTransport...\n"); 00077 //Set radio APN 00078 for (int i = 0; i < 10; i++) { 00079 if (i >= 10) { 00080 logError("Failed to set APN to %s", APN); 00081 } 00082 if (radio->setApn(APN) == MTS_SUCCESS) { 00083 logInfo("Successfully set APN to %s", APN); 00084 break; 00085 } else { 00086 wait(1); 00087 } 00088 } 00089 00090 //Establish PPP link 00091 for (int i = 0; i < 10; i++) { 00092 if (i >= 10) { 00093 logError("Failed to establish PPP link"); 00094 } 00095 if (radio->connect() == true) { 00096 logInfo("Successfully established PPP link"); 00097 break; 00098 } else { 00099 wait(1); 00100 } 00101 } 00102 00103 //Ping google.com 00104 for (int i = 0; i < 10; i++) { 00105 if (i >= 10) { 00106 logError("Failed to ping api-m2x.att.com"); 00107 } 00108 if (radio->ping("api-m2x.att.com") == true) { 00109 logInfo("Successfully pinged api-m2x.att.com"); 00110 ping_status = 1; 00111 break; 00112 } else { 00113 wait(1); 00114 } 00115 } 00116 00117 // Initialize the M2X client 00118 Client client; 00119 M2XStreamClient m2xClient(&client, m2xKey); 00120 00121 if (ping_status) 00122 { 00123 mypwm.pulsewidth_ms(500); 00124 00125 /* Main loop */ 00126 while (true) 00127 { 00128 00129 /* Wait 5 secs and then loop */ 00130 delay(2500); 00131 mypwm.pulsewidth_ms(0); 00132 00133 /* Read ADC value from analog sensor */ 00134 uint16_t a = temp_sensor.read_u16(); 00135 00136 /* Calculate the temperature in Fareheight and Celsius */ 00137 resistance = (float)(adc_scale-a)*10000/a; //get the resistance of the sensor; 00138 temperature = 1/(log(resistance/10000)/B+1/298.15)-273.15; //convert to temperature via datasheet ; 00139 temperature_f =(1.8 * temperature) + 32.0; 00140 sprintf(amb_temp, "%0.2f", temperature_f); 00141 00142 printf("Temp Sensor Analog Reading is 0x%X = %d \r\n", a, a); 00143 printf("Current Temperature: %f C %f F \r\n", temperature, temperature_f); 00144 00145 delay(2500); 00146 mypwm.pulsewidth_ms(500); 00147 00148 /* Post temperature to M2X site */ 00149 int response = m2xClient.put(feedId, streamName, amb_temp); 00150 printf("Post response code: %d\r\n", response); 00151 if (response == -1) 00152 { 00153 mypwm.pulsewidth_ms(250); 00154 printf("Temperature data transmit post error\n"); 00155 } 00156 /* Update location data */ 00157 response = m2xClient.updateLocation(feedId, name, latitude, longitude, elevation); 00158 printf("updateLocation response code: %d\r\n", response); 00159 if (response == -1) 00160 { 00161 mypwm.pulsewidth_ms(250); 00162 printf("Location data transmit post error\n"); 00163 } 00164 printf("\r\n"); 00165 } 00166 } 00167 00168 //Disconnect ppp link 00169 radio->disconnect(); 00170 00171 logInfo("End of example code"); 00172 return 0; 00173 }
Generated on Sun Jul 31 2022 15:25:15 by
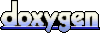