M2XStreamClient fork with a workaround in M2XStreamClient.cpp for the MTS_Wifi_Connect_M2X example.
Dependents: STM32_MTS_Wifi_Connect_M2X M2X_STM32_MTS_Temp MTS_WiFi_Connect_M2X_Example
Fork of M2XStreamClient by
Client.cpp
00001 #include "Client.h" 00002 #include "mbed.h" 00003 00004 #include <stdint.h> 00005 00006 Client::Client() : _len(0), _sock() { 00007 } 00008 00009 Client::~Client() { 00010 } 00011 00012 int Client::connect(const char *host, uint16_t port) { 00013 return _sock.connect(host, port) == 0; 00014 } 00015 00016 size_t Client::write(uint8_t b) { 00017 return write(&b, 1); 00018 } 00019 00020 size_t Client::write(const uint8_t *buf, size_t size) { 00021 _sock.set_blocking(false, 15000); 00022 // NOTE: we know it's dangerous to cast from (const uint8_t *) to (char *), 00023 // but we are trying to maintain a stable interface between the Arduino 00024 // one and the mbed one. What's more, while TCPSocketConnection has no 00025 // intention of modifying the data here, it requires us to send a (char *) 00026 // typed data. So we belive it's safe to do the cast here. 00027 return _sock.send_all(const_cast<char*>((const char*) buf), size); 00028 } 00029 00030 int Client::available() { 00031 if (_len > 0) { return 1; } 00032 int ret = read(_buf, 1); 00033 if (ret <= 0) { return 0; } 00034 _len = ret; 00035 return 1; 00036 } 00037 00038 int Client::read() { 00039 if (_len > 0) { 00040 _len = 0; 00041 return _buf[0]; 00042 } 00043 return -1; 00044 } 00045 00046 int Client::read(uint8_t *buf, size_t size) { 00047 return _sock.receive_all((char*) buf, size); 00048 } 00049 00050 void Client::flush() { 00051 // does nothing, TCP stack takes care of this 00052 } 00053 00054 void Client::stop() { 00055 _sock.close(); 00056 } 00057 00058 uint8_t Client::connected() { 00059 return _sock.is_connected(); 00060 }
Generated on Fri Jul 15 2022 12:10:59 by
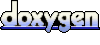