Test program making the LEDs blink.
Dependencies: mbed-rtos mbed-src
main.cpp
00001 /****************************************************************************** 00002 * DESCRIPTION: 00003 * A "Blinky" CMSIS RTOS program which demonstrates another safe way 00004 * to pass arguments to threads during thread creation. In this case, 00005 * a structure is used to pass multiple arguments. 00006 * AUTHOR: Jose M. Gomez 00007 * LAST REVISED: 23/SEP/2014 00008 ******************************************************************************/ 00009 00010 #include <mbed.h> 00011 #include <cmsis_os.h> 00012 #include <stdint.h> 00013 #include <limits.h> 00014 00015 #define NUM_THREADS 6 00016 00017 DigitalOut red(LED1); 00018 DigitalOut green(LED2); 00019 DigitalOut blue(LED3); 00020 00021 void Timer1_Callback (void const *arg) { 00022 red = !red; // update the counter 00023 } 00024 00025 void Timer2_Callback (void const *arg) { 00026 blue=!blue; // update the counter 00027 } 00028 00029 osTimerDef (timer1, Timer1_Callback); 00030 osTimerDef (timer2, Timer2_Callback); 00031 00032 int main (void) { 00033 osTimerId id1; 00034 osTimerId id2; 00035 00036 green = 0; 00037 id1 = osTimerCreate (osTimer(timer1), osTimerPeriodic, NULL); 00038 osTimerStart (id1, 100UL); 00039 id2 = osTimerCreate (osTimer(timer2), osTimerPeriodic, NULL); 00040 osTimerStart (id2, 157UL); 00041 green = 1; 00042 }
Generated on Thu Jul 14 2022 16:00:17 by
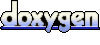