
Dhrystone Benchmark Program C/1 12/01/84
Embed:
(wiki syntax)
Show/hide line numbers
dhry.cpp
00001 00002 #include <stdio.h> 00003 #include <stdlib.h> 00004 #include <stdbool.h> 00005 #include <string.h> 00006 00007 #include "dhry.h" 00008 00009 00010 #ifdef NOSTRUCTASSIGN 00011 # define structassign(d, s) memcpy(&(d), &(s), sizeof(d)) 00012 #else 00013 # define structassign(d, s) d = s 00014 #endif 00015 00016 typedef enum { 00017 Ident1, Ident2, Ident3, Ident4, Ident5 00018 } Enumeration; 00019 00020 typedef int OneToThirty; 00021 typedef int OneToFifty; 00022 typedef char CapitalLetter; 00023 typedef char String30[31]; 00024 typedef int Array1Dim[51]; 00025 typedef int Array2Dim[51][51]; 00026 00027 struct Record { 00028 struct Record *PtrComp; 00029 Enumeration Discr; 00030 Enumeration EnumComp; 00031 OneToFifty IntComp; 00032 String30 StringComp; 00033 }; 00034 00035 typedef struct Record RecordType; 00036 typedef RecordType *RecordPtr; 00037 00038 #ifndef NULL 00039 # define NULL (void *)0 00040 #endif 00041 00042 #ifndef FALSE 00043 # define FALSE 0 00044 # define TRUE (!FALSE) 00045 #endif 00046 00047 #ifndef REG 00048 # define REG 00049 #endif 00050 00051 00052 void Proc0( void ); 00053 void Proc1( RecordPtr PtrParIn ); 00054 void Proc2( OneToFifty *IntParIO ); 00055 void Proc3( RecordPtr *PtrParOut ); 00056 void Proc4( void ); 00057 void Proc5( void ); 00058 void Proc6( Enumeration EnumParIn, 00059 Enumeration *EnumParOut ); 00060 void Proc7( OneToFifty IntParI1, 00061 OneToFifty IntParI2, 00062 OneToFifty *IntParOut ); 00063 void Proc8( Array1Dim Array1Par, 00064 Array2Dim Array2Par, 00065 OneToFifty IntParI1, 00066 OneToFifty IntParI2 ); 00067 Enumeration Func1( CapitalLetter CharPar1, 00068 CapitalLetter CharPar2 ); 00069 bool Func2( String30 StrParI1, 00070 String30 StrParI2 ); 00071 bool Func3( Enumeration EnumParIn ); 00072 00073 00074 int IntGlob; 00075 int cnt, sum_flag; 00076 bool BoolGlob; 00077 char Char1Glob; 00078 char Char2Glob; 00079 Array1Dim Array1Glob; 00080 Array2Dim Array2Glob; 00081 RecordPtr PtrGlb; 00082 RecordPtr PtrGlbNext; 00083 00084 /****************************************************************************/ 00085 00086 void Proc0 (void) 00087 { 00088 OneToFifty IntLoc1; 00089 OneToFifty IntLoc2; 00090 OneToFifty IntLoc3; 00091 char CharIndex; 00092 Enumeration EnumLoc; 00093 String30 String1Loc, String2Loc; 00094 unsigned long idx; 00095 00096 PtrGlbNext = (RecordPtr) malloc(sizeof(RecordType)); 00097 PtrGlb = (RecordPtr) malloc(sizeof(RecordType)); 00098 PtrGlb->PtrComp = PtrGlbNext; 00099 PtrGlb->Discr = Ident1; 00100 PtrGlb->EnumComp = Ident3; 00101 PtrGlb->IntComp = 40; 00102 strcpy(PtrGlb->StringComp, "DHRYSTONE PROGRAM, SOME STRING"); 00103 00104 for (idx = 0; idx < LOOPS; idx++) { 00105 Proc5(); 00106 Proc4(); 00107 00108 IntLoc1 = 2; 00109 IntLoc2 = 3; 00110 IntLoc3 = 0; 00111 00112 strcpy(String2Loc, "DHRYSTONE PROGRAM, 2'ND STRING"); 00113 00114 EnumLoc = Ident2; 00115 BoolGlob = ! Func2(String1Loc, String2Loc); 00116 00117 while (IntLoc1 < IntLoc2) { 00118 IntLoc3 = 5 * IntLoc1 - IntLoc2; 00119 Proc7(IntLoc1, IntLoc2, &IntLoc3); 00120 ++IntLoc1; 00121 } 00122 00123 Proc8(Array1Glob, Array2Glob, IntLoc1, IntLoc3); 00124 Proc1(PtrGlb); 00125 00126 for (CharIndex = 'A'; CharIndex <= Char2Glob; ++CharIndex) 00127 if (EnumLoc == Func1(CharIndex, 'C')) 00128 Proc6(Ident1, &EnumLoc); 00129 00130 IntLoc3 = IntLoc2 * IntLoc1; 00131 IntLoc2 = IntLoc3 / IntLoc1; 00132 IntLoc2 = 7 * (IntLoc3 - IntLoc2) - IntLoc1; 00133 Proc2(&IntLoc1); 00134 } 00135 00136 free(PtrGlbNext); 00137 free(PtrGlb); 00138 } 00139 00140 00141 void Proc1( RecordPtr PtrParIn ) 00142 { 00143 structassign(*(PtrParIn->PtrComp), *PtrGlb); 00144 PtrParIn->IntComp = 5; 00145 PtrParIn->PtrComp->IntComp = PtrParIn->IntComp; 00146 PtrParIn->PtrComp->PtrComp = PtrParIn->PtrComp; 00147 Proc3((RecordPtr *)(PtrParIn->PtrComp->PtrComp)); 00148 if ((PtrParIn->PtrComp)->Discr == Ident1) { 00149 PtrParIn->PtrComp->IntComp = 6; 00150 Proc6(PtrParIn->EnumComp, &(PtrParIn->PtrComp->EnumComp)); 00151 PtrParIn->PtrComp->PtrComp = PtrGlb->PtrComp; 00152 Proc7(PtrParIn->PtrComp->IntComp, 10, 00153 &(PtrParIn->PtrComp->IntComp)); 00154 } else 00155 structassign(*PtrParIn, *(PtrParIn->PtrComp)); 00156 } 00157 00158 00159 void Proc2( OneToFifty *IntParIO ) 00160 { 00161 OneToFifty IntLoc; 00162 Enumeration EnumLoc; 00163 00164 IntLoc = *IntParIO + 10; 00165 00166 for(;;) { 00167 if (Char1Glob == 'A') { 00168 IntLoc -= 1; 00169 *IntParIO = IntLoc - IntGlob; 00170 EnumLoc = Ident1; 00171 } 00172 00173 if (EnumLoc == Ident1) 00174 break; 00175 } 00176 } 00177 00178 00179 void Proc3( RecordPtr *PtrParOut ) 00180 { 00181 if (PtrGlb != NULL) 00182 *PtrParOut = PtrGlb->PtrComp; 00183 else 00184 IntGlob = 100; 00185 00186 Proc7(10, IntGlob, &PtrGlb->IntComp); 00187 } 00188 00189 00190 void Proc4( void ) 00191 { 00192 bool BoolLoc; 00193 00194 BoolLoc = Char1Glob == 'A'; 00195 BoolLoc |= BoolGlob; 00196 Char2Glob = 'B'; 00197 } 00198 00199 00200 void Proc5( void ) 00201 { 00202 Char1Glob = 'A'; 00203 BoolGlob = FALSE; 00204 } 00205 00206 00207 void Proc6( Enumeration EnumParIn, Enumeration *EnumParOut ) 00208 { 00209 *EnumParOut = EnumParIn; 00210 00211 if (! Func3(EnumParIn)) 00212 *EnumParOut = Ident4; 00213 00214 switch (EnumParIn) { 00215 case Ident1: 00216 *EnumParOut = Ident1; 00217 break; 00218 case Ident2: 00219 *EnumParOut = (IntGlob > 100)? Ident1 : Ident4; 00220 break; 00221 case Ident3: 00222 *EnumParOut = Ident2; 00223 break; 00224 case Ident4: 00225 break; 00226 case Ident5: 00227 *EnumParOut = Ident3; 00228 break; 00229 } 00230 } 00231 00232 00233 void Proc7( OneToFifty IntParI1, 00234 OneToFifty IntParI2, 00235 OneToFifty *IntParOut ) 00236 { 00237 OneToFifty IntLoc; 00238 00239 IntLoc = IntParI1 + 2; 00240 *IntParOut = IntParI2 + IntLoc; 00241 } 00242 00243 00244 void Proc8( Array1Dim Array1Par, 00245 Array2Dim Array2Par, 00246 OneToFifty IntParI1, 00247 OneToFifty IntParI2 ) 00248 { 00249 OneToFifty IntLoc; 00250 OneToFifty IntIndex; 00251 00252 IntLoc = IntParI1 + 5; 00253 Array1Par[IntLoc] = IntParI2; 00254 Array1Par[IntLoc+1] = Array1Par[IntLoc]; 00255 Array1Par[IntLoc+30] = IntLoc; 00256 00257 for (IntIndex = IntLoc; IntIndex <= (IntLoc+1); ++IntIndex) 00258 Array2Par[IntLoc][IntIndex] = IntLoc; 00259 00260 ++Array2Par[IntLoc][IntLoc-1]; 00261 Array2Par[IntLoc+20][IntLoc] = Array1Par[IntLoc]; 00262 IntGlob = 5; 00263 } 00264 00265 00266 Enumeration Func1( CapitalLetter CharPar1, 00267 CapitalLetter CharPar2 ) 00268 { 00269 CapitalLetter CharLoc1; 00270 CapitalLetter CharLoc2; 00271 00272 CharLoc1 = CharPar1; 00273 CharLoc2 = CharLoc1; 00274 00275 return ((CharLoc2 != CharPar2) ? Ident1 : Ident2); 00276 } 00277 00278 00279 bool Func2( String30 StrParI1, 00280 String30 StrParI2 ) 00281 { 00282 OneToThirty IntLoc; 00283 CapitalLetter CharLoc; 00284 00285 IntLoc = 1; 00286 while (IntLoc <= 1) { 00287 if (Func1(StrParI1[IntLoc], StrParI2[IntLoc+1]) == Ident1) { 00288 CharLoc = 'A'; 00289 ++IntLoc; 00290 } 00291 } 00292 00293 if (CharLoc >= 'W' && CharLoc <= 'Z') { 00294 IntLoc = 7; 00295 } 00296 00297 if (CharLoc == 'X') { 00298 return(TRUE); 00299 } else { 00300 if (strcmp(StrParI1, StrParI2) > 0) { 00301 IntLoc += 7; 00302 return (TRUE); 00303 } else 00304 return (FALSE); 00305 } 00306 } 00307 00308 00309 bool Func3( Enumeration EnumParIn ) 00310 { 00311 Enumeration EnumLoc; 00312 00313 EnumLoc = EnumParIn; 00314 00315 return (EnumLoc == Ident3); 00316 }
Generated on Mon Jul 25 2022 04:12:17 by
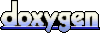