It is a library for controlling Wallbot
Embed:
(wiki syntax)
Show/hide line numbers
wallbot.h
00001 /* mbed wallbot Library 00002 * 00003 * wallbot.h 00004 * 00005 * Copyright (c) 2010-2013 jksoft 00006 * 00007 * Permission is hereby granted, free of charge, to any person obtaining a copy 00008 * of this software and associated documentation files (the "Software"), to deal 00009 * in the Software without restriction, including without limitation the rights 00010 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00011 * copies of the Software, and to permit persons to whom the Software is 00012 * furnished to do so, subject to the following conditions: 00013 * 00014 * The above copyright notice and this permission notice shall be included in 00015 * all copies or substantial portions of the Software. 00016 * 00017 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00018 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00019 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00020 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00021 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00022 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00023 * THE SOFTWARE. 00024 */ 00025 00026 #ifndef WALLBOT_H 00027 #define WALLBOT_H 00028 00029 #include "mbed.h" 00030 #include "HighSpeedAnalogIn.h" 00031 #include "EthernetPowerControl.h" 00032 #include "TB6612.h" 00033 00034 #define SENSOR_NOMAL 0 00035 #define SENSOR_EXT 1 00036 #define SENSOR_THRESHOLD 2500 00037 /** wallbot control class 00038 * 00039 * Example: 00040 * @code 00041 * // Drive the wwallbot forward, turn left, back, turn right, at half speed for half a second 00042 00043 #include "mbed.h" 00044 #include "wallbot.h" 00045 00046 wallbot wb; 00047 00048 int main() { 00049 00050 wait(0.5); 00051 00052 wb.forward(0.5); 00053 wait (0.5); 00054 wb.left(0.5); 00055 wait (0.5); 00056 wb.backward(0.5); 00057 wait (0.5); 00058 wb.right(0.5); 00059 wait (0.5); 00060 00061 wb.stop(); 00062 00063 } 00064 * @endcode 00065 */ 00066 class wallbot { 00067 00068 // Public functions 00069 public: 00070 00071 /** Create the wallbot object connected to the default pins 00072 */ 00073 wallbot(); 00074 00075 /** Directly control the speed and direction of the left motor 00076 * 00077 * @param speed A normalised number -1.0 - 1.0 represents the full range. 00078 */ 00079 void left_motor (float speed); 00080 00081 /** Directly control the speed and direction of the right motor 00082 * 00083 * @param speed A normalised number -1.0 - 1.0 represents the full range. 00084 */ 00085 void right_motor (float speed); 00086 00087 /** Drive both motors forward as the same speed 00088 * 00089 * @param speed A normalised number 0 - 1.0 represents the full range. 00090 */ 00091 void forward (float speed); 00092 00093 /** Drive both motors backward as the same speed 00094 * 00095 * @param speed A normalised number 0 - 1.0 represents the full range. 00096 */ 00097 void backward (float speed); 00098 00099 /** Drive left motor backwards and right motor forwards at the same speed to turn on the spot 00100 * 00101 * @param speed A normalised number 0 - 1.0 represents the full range. 00102 */ 00103 void left (float speed); 00104 00105 /** Drive left motor forward and right motor backwards at the same speed to turn on the spot 00106 * @param speed A normalised number 0 - 1.0 represents the full range. 00107 */ 00108 void right (float speed); 00109 00110 /** Stop both motors 00111 * 00112 */ 00113 void stop (void); 00114 00115 /** Get floorline position.(float value return.) 00116 * 00117 */ 00118 float GetLinePosition(void); 00119 00120 /** Get floorline position.(bit value return.) 00121 * 00122 */ 00123 void GetLinePosition(int *bit); 00124 00125 /** Get left switch .(switch OFF:0 or ON:1 return.) 00126 * 00127 */ 00128 int GetLeftSw(void); 00129 00130 /** Get GetRightSw switch .(switch OFF:0 or ON:1 return.) 00131 * 00132 */ 00133 int GetRightSw(void); 00134 00135 private : 00136 00137 TB6612 _right; 00138 TB6612 _left; 00139 00140 HighSpeedAnalogIn _ain; 00141 00142 DigitalIn _left_sw; 00143 DigitalIn _right_sw; 00144 00145 int _floorSensorThreshold; 00146 00147 }; 00148 00149 #endif
Generated on Sun Jul 17 2022 09:53:56 by
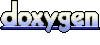