
うおーるぼっと用プログラム Wiiリモコンからのダイレクト操作モードのみ BlueUSBをベースに使用しています。
Dependencies: BD6211F mbed SimpleFilter
Wiimote.cpp
00001 /* Copyright (c) 2011, mbed 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy 00004 * of this software and associated documentation files (the "Software"), to deal 00005 * in the Software without restriction, including without limitation the rights 00006 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00007 * copies of the Software, and to permit persons to whom the Software is 00008 * furnished to do so, subject to the following conditions: 00009 * 00010 * The above copyright notice and this permission notice shall be included in 00011 * all copies or substantial portions of the Software. 00012 * 00013 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00014 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00015 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00016 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00017 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00018 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00019 * THE SOFTWARE. 00020 */ 00021 00022 // Simple decode class for Wii Remote data 00023 00024 #include "Wiimote.h" 00025 00026 // Wii Report Formats: 00027 // * http://wiibrew.org/wiki/Wiimote#0x37:_Core_Buttons_and_Accelerometer_with_10_IR_bytes_and_6_Extension_Bytes 00028 // * http://wiki.wiimoteproject.com/Reports#0x37_BTN_.2B_XLR_.2B_IR_.2B_6_EXT 00029 00030 // Input Report 0x37: 00031 // 00032 // 0 [ ? | X(1:0) | PLUS | UP | DOWN | RIGHT | LEFT ] 00033 // 1 [ HOME | Z(1) | Y(1) | MINUS | A | B | ONE | TWO ] 00034 // 2 [ X(9:2) ] 00035 // 3 [ Y(9:2) ] 00036 // 4 [ Z(9:2) ] 00037 // 00038 00039 #include <stdio.h> 00040 #include <math.h> 00041 00042 #define WII_ZERO 0x206 00043 #define WII_1G 0x6A 00044 00045 void Wiimote::decode(char* data) { 00046 00047 // read buttons 00048 left = (data[0] >> 0) & 0x1; 00049 right = (data[0] >> 1) & 0x1; 00050 down = (data[0] >> 2) & 0x1; 00051 up = (data[0] >> 3) & 0x1; 00052 plus = (data[0] >> 4) & 0x1; 00053 two = (data[1] >> 0) & 0x1; 00054 one = (data[1] >> 1) & 0x1; 00055 b = (data[1] >> 2) & 0x1; 00056 a = (data[1] >> 3) & 0x1; 00057 minus = (data[1] >> 4) & 0x1; 00058 home = (data[1] >> 7) & 0x1; 00059 00060 // read accelerometers 00061 rawx = (data[2] << 2) | (data[0] & 0x60) >> 5; 00062 rawy = (data[3] << 2) | (data[1] & 0x20) >> 4; 00063 rawz = (data[4] << 2) | (data[1] & 0x40) >> 5; 00064 00065 // calculate accelerometer gravity 00066 x = (float)((int)rawx - WII_ZERO) / (float)WII_1G; 00067 y = (float)((int)rawy - WII_ZERO) / (float)WII_1G; 00068 z = (float)((int)rawz - WII_ZERO) / (float)WII_1G; 00069 00070 // calculate wheel angle 00071 wheel = atan2(-y, -x) * (180.0 / 3.141592); 00072 } 00073 00074 void Wiimote::dump() { 00075 printf("%d%d%d%d%d%d%d%d%d%d%d %.3f %.3f %.3f %.2f\n", left, right, down, up, plus, two, one, b, a, minus, home, x, y, z, wheel); 00076 } 00077 00078 00079 // Accelerometer data 00080 // y- 00081 // +---+ +--+ 00082 // | + | + B 00083 // | A | A | 00084 // | | | | 00085 // x+ | | x- z+ | | z- 00086 // | 1 | 1 | 00087 // | 2 | 2 | 00088 // +---+ +--+ 00089 // y+ 00090 // 00091 // x+ 0x19B 00092 // x0 0x205 00093 // x- 0x26E 00094 // 00095 // y+ 0x19C 0x6A 00096 // y0 0x206 00097 // y- 0x26E 0x68 00098 // 00099 // z+ 0x19C 00100 // z0 0x208 00101 // z- 0x26E
Generated on Thu Jul 14 2022 13:01:38 by
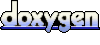