データの保存、更新、取得ができるWebサービス「milkcocoa」に接続し、データのプッシュ、送信、取得ができるライブラリです。 https://mlkcca.com/
Dependents: MilkcocoaSample MilkcocoaSampleESP8266_LED MilkcocoaSampleESP8266 MilkcocoaSample_3G ... more
Milkcocoa.cpp
00001 #include "Milkcocoa.h" 00002 00003 #if 0 00004 #if 0 00005 #include "SoftSerialSendOnry.h" 00006 extern SoftSerialSendOnry pc; 00007 #else 00008 extern Serial pc; 00009 #endif 00010 #define DBG(x) x 00011 #else 00012 #define DBG(x) 00013 #endif 00014 00015 DataElement::DataElement() { 00016 json_msg[0] = '\0'; 00017 strcpy(json_msg,"{\"params\":{"); 00018 } 00019 00020 DataElement::DataElement(char *json_string) { 00021 json_msg[0] = '\0'; 00022 strcpy(json_msg,json_string); 00023 } 00024 00025 void DataElement::setValue(const char *key, const char *v) { 00026 char json_string[64]; 00027 if( json_msg[strlen(json_msg)-1] != '{' ) 00028 { 00029 strcat(json_msg,","); 00030 } 00031 sprintf(json_string,"\"%s\":\"%s\"",key,v); 00032 strcat(json_msg,json_string); 00033 } 00034 00035 void DataElement::setValue(const char *key, int v) { 00036 char json_string[64]; 00037 if( json_msg[strlen(json_msg)-1] != '{' ) 00038 { 00039 strcat(json_msg,","); 00040 } 00041 sprintf(json_string,"\"%s\":\"%d\"",key,v); 00042 strcat(json_msg,json_string); 00043 } 00044 00045 void DataElement::setValue(const char *key, double v) { 00046 char json_string[64]; 00047 if( json_msg[strlen(json_msg)-1] != '{' ) 00048 { 00049 strcat(json_msg,","); 00050 } 00051 sprintf(json_string,"\"%s\":\"%f\"",key,v); 00052 strcat(json_msg,json_string); 00053 } 00054 00055 char *DataElement::getString(const char *key) { 00056 static char _word[64]; 00057 char *p; 00058 int i=0; 00059 00060 strcpy(_word , "\"\0"); 00061 strcat(_word , key ); 00062 strcat(_word , "\"" ); 00063 00064 p = strstr( (char*)json_msg , _word ) + 2 + strlen(_word); 00065 00066 while( (p[i] != ',')&&(p[i] != '\n')&&(p[i] != '\"') ) 00067 { 00068 _word[i] = p[i]; 00069 i++; 00070 } 00071 _word[i] = '\0'; 00072 00073 return _word; 00074 } 00075 00076 int DataElement::getInt(const char *key) { 00077 return atoi(getString(key)); 00078 } 00079 00080 float DataElement::getFloat(const char *key) { 00081 return atof(getString(key)); 00082 } 00083 00084 char *DataElement::toCharArray() { 00085 if( json_msg[strlen(json_msg)-1] != '{' ) 00086 { 00087 strcat(json_msg,"}"); 00088 } 00089 strcat(json_msg,"}"); 00090 00091 return(json_msg); 00092 } 00093 00094 Milkcocoa::Milkcocoa(MClient *_client, const char *host, uint16_t port, const char *_app_id, const char *client_id) { 00095 00096 client = _client; 00097 strcpy(servername,host); 00098 portnum = port; 00099 app_id = _app_id; 00100 strcpy(_clientid,client_id); 00101 strcpy(username,"sdammy"); 00102 strcpy(password,app_id); 00103 00104 } 00105 00106 Milkcocoa::Milkcocoa(MClient *_client, const char *host, uint16_t port, const char *_app_id, const char *client_id, char *_session) { 00107 00108 client = _client; 00109 strcpy(servername,host); 00110 portnum = port; 00111 app_id = _app_id; 00112 strcpy(_clientid,client_id); 00113 strcpy(username,_session); 00114 strcpy(password,app_id); 00115 00116 } 00117 00118 Milkcocoa* Milkcocoa::createWithApiKey(MClient *_client, const char *host, uint16_t port, const char *_app_id, const char *client_id, char *key, char *secret) { 00119 char session[60]; 00120 sprintf(session, "k%s:%s", key, secret); 00121 return new Milkcocoa(_client, host, port, _app_id, client_id, session); 00122 } 00123 00124 void Milkcocoa::connect() { 00125 00126 if(client->isConnected()) 00127 return; 00128 00129 if(client->connect(servername, portnum)!=0) { 00130 DBG(pc.printf("Network connect err\r\n");) 00131 return; 00132 } 00133 00134 MQTTPacket_connectData data = MQTTPacket_connectData_initializer; 00135 data.keepAliveInterval = 20; 00136 data.cleansession = 1; 00137 data.MQTTVersion = 4; 00138 data.clientID.cstring = _clientid; 00139 data.username.cstring = username; 00140 data.password.cstring = password; 00141 00142 if (client->connect(data) != 0) { 00143 DBG(pc.printf("Milkcocoa connect err\r\n");) 00144 return; 00145 } 00146 } 00147 00148 bool Milkcocoa::push(const char *path, DataElement dataelement) { 00149 char topic[100]; 00150 char *buf; 00151 MQTT::Message message; 00152 00153 sprintf(topic, "%s/%s/push", app_id, path); 00154 00155 message.qos = MQTT::QOS0; 00156 message.retained = 0; 00157 message.dup = false; 00158 buf = dataelement.toCharArray(); 00159 message.payload = (void*)buf; 00160 message.payloadlen = strlen(buf); 00161 if(client->publish(topic, message)!=0) 00162 return(false); 00163 00164 return true; 00165 } 00166 00167 bool Milkcocoa::send(const char *path, DataElement dataelement) { 00168 char topic[100]; 00169 char *buf; 00170 MQTT::Message message; 00171 00172 sprintf(topic, "%s/%s/send", app_id, path); 00173 message.qos = MQTT::QOS0; 00174 message.retained = 0; 00175 message.dup = false; 00176 buf = dataelement.toCharArray(); 00177 message.payload = (void*)buf; 00178 message.payloadlen = strlen(buf); 00179 if(client->publish(topic, message)!=0) 00180 return false; 00181 00182 return true; 00183 } 00184 00185 void Milkcocoa::loop() { 00186 connect(); 00187 client->yield(RECV_TIMEOUT); 00188 } 00189 00190 bool Milkcocoa::on(const char *path, const char *event, GeneralFunction cb) { 00191 MilkcocoaSubscriber *sub = new MilkcocoaSubscriber(cb); 00192 sprintf(sub->topic, "%s/%s/%s", app_id, path, event); 00193 00194 if (client->subscribe(sub->topic, MQTT::QOS0, cb) != 0) { 00195 DBG(pc.printf("Milkcocoa subscribe err\r\n");) 00196 return false; 00197 } 00198 for (int i=0; i<MILKCOCOA_SUBSCRIBERS; i++) { 00199 if (milkcocoaSubscribers[i] == sub) { 00200 return false; 00201 } 00202 } 00203 for (int i=0; i<MILKCOCOA_SUBSCRIBERS; i++) { 00204 if (milkcocoaSubscribers[i] == 0) { 00205 milkcocoaSubscribers[i] = sub; 00206 return true; 00207 } 00208 } 00209 return true; 00210 } 00211 00212 MilkcocoaSubscriber::MilkcocoaSubscriber(GeneralFunction _cb) { 00213 cb = _cb; 00214 }
Generated on Thu Jul 14 2022 13:39:07 by
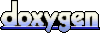