M0版mbedのGPSロガープログラムです。
Dependencies: ChaNFSSD FatFileSystem
main.cpp
00001 // GPS Logger mbed LPC11U24 00002 00003 #include "mbed.h" 00004 #include "SDFileSystem.h" 00005 00006 SDFileSystem sd(p5, p6, p7, p8, "fs"); 00007 00008 Serial gps(p9, p10); 00009 Serial pc(USBTX, USBRX); 00010 00011 InterruptIn pps(p14); 00012 00013 DigitalOut pps_stat(LED1); 00014 DigitalOut sd_access(LED2); 00015 DigitalOut rx_status(LED3); 00016 DigitalIn enable(p22); 00017 00018 int num = 0; 00019 int pps_count = 0; 00020 00021 // GPS 1pps interrupt 00022 void int_pps() { 00023 pps_stat =! pps_stat; 00024 pps_count += 1; 00025 } 00026 00027 00028 int main() { 00029 00030 char tmp; 00031 char data[80]; 00032 int log_count = 0; 00033 bool rec_flag = false; 00034 int before_sw = 1; 00035 FILE *fp = NULL; 00036 00037 enable.mode(PullUp); 00038 00039 pps_stat = 0; 00040 sd_access = 0; 00041 rx_status = 0; 00042 00043 pps.rise(&int_pps); 00044 gps.baud(115200); 00045 00046 00047 sd_access = 1; 00048 00049 while (1) { 00050 int sw = enable; 00051 00052 if( (sw == 0) && (before_sw == 1) ) 00053 { 00054 if( rec_flag ) 00055 { 00056 rec_flag = false; 00057 fclose(fp); 00058 fp = NULL; 00059 } 00060 else 00061 { 00062 rec_flag = true; 00063 fp = fopen("/fs/gps.log", "w"); 00064 } 00065 wait(0.2); 00066 } 00067 before_sw = sw; 00068 00069 if(gps.readable()) { 00070 tmp = gps.getc(); 00071 data[num] = tmp; 00072 if( data[num] == '$' ) { 00073 data[0] = '$'; 00074 num = 1; 00075 } 00076 else if( data[num-1] == '\r' && data[num] == '\n' ) { 00077 00078 if(rec_flag) rx_status = !rx_status; 00079 00080 data[num+1] = '\0'; 00081 00082 if(memcmp(data, "$GPRMC",6) == 0) { 00083 00084 if(pps_count >= 5) { 00085 pps_count = 0; 00086 00087 if( fp != NULL ) { 00088 sd_access =! sd_access; 00089 fprintf(fp,"%s",data); 00090 } 00091 00092 } 00093 if(rec_flag) deepsleep(); 00094 } 00095 data[0] = '\0'; 00096 num = 0; 00097 } 00098 else { 00099 num++; 00100 if(num > 80) 00101 { 00102 num = 0; 00103 } 00104 } 00105 } 00106 } 00107 }
Generated on Tue Jul 19 2022 16:23:40 by
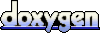