
Radio Structures in OOP
Embed:
(wiki syntax)
Show/hide line numbers
CC1101.h
00001 #ifndef CC1101_RADIO_H 00002 #define CC1101_RADIO_H 00003 00004 #include "mbed.h" 00005 #include "CommLink.h" 00006 #include "cmsis_os.h" 00007 #include "RTP.h" 00008 #include "CC1101-Defines.h" 00009 00010 #define CCXXX1_DEBUG_MODE 0 00011 00012 class CC1101 : public CommLink 00013 { 00014 public: 00015 // Default constructor 00016 CC1101(); 00017 CC1101(PinName mosi, PinName miso, PinName sck, PinName cs, PinName int_pin = NC); 00018 00019 // Deconstructor 00020 virtual ~CC1101(); 00021 00022 // These must have implementations in the CC1101 class since it is a derived class of the base class CommLink 00023 virtual void reset(void); 00024 virtual int32_t selfTest(void); 00025 virtual bool isConnected(void); 00026 00027 // Set/Get the chip's operating channel 00028 void channel(uint16_t); 00029 uint16_t channel(void); 00030 00031 // Set/Get the chip's address if applicable 00032 void address(uint8_t); 00033 uint8_t address(void); 00034 00035 // Get the chip's datarate (baudrate) 00036 uint16_t datarate(void); 00037 00038 // The NOP command used to get the CC1101's status byte 00039 uint8_t status(void); 00040 uint8_t status(uint8_t); 00041 uint8_t lqi(void); 00042 uint8_t version(void); 00043 int16_t rssi(void); 00044 00045 int32_t powerUp(void); 00046 00047 protected: 00048 // These must have implementations in the CC1101 class since it is a derived class of the base class CommLink 00049 virtual int32_t sendData(uint8_t*, uint8_t); 00050 virtual int32_t getData(uint8_t*, uint8_t*); 00051 00052 // Reading/Writing registers 00053 void write_reg(uint8_t, uint8_t); 00054 void write_reg(uint8_t, uint8_t*, uint8_t); 00055 uint8_t read_reg(uint8_t); 00056 void read_reg(uint8_t, uint8_t*, uint8_t); 00057 00058 // Send a command strobe to the CC1101 (1 byte SPI transfer) 00059 uint8_t strobe(uint8_t); 00060 00061 // Send the TX or RX command strobe for placing the CC1101 in the respective state 00062 void tx_mode(void); 00063 void rx_mode(void); 00064 void idle(void); 00065 00066 // Send the command strobe to flush the TX or RX buffers on the CC1101 00067 void flush_tx(void); 00068 void flush_rx(void); 00069 00070 void freq(uint32_t); 00071 void datarate(uint32_t); // set data rate 00072 void put_rf_settings(void); 00073 void init(void); 00074 00075 private: 00076 00077 00078 void calibrate(void); 00079 void tiny_delay(void); 00080 void rssi(uint8_t); 00081 uint8_t mode(void); 00082 00083 void assign_modem_params(void); 00084 void assign_packet_params(void); 00085 void interface_freq(uint32_t); 00086 void assign_channel_spacing(uint32_t); 00087 void set_rf_settings(void); 00088 void set_init_vars(void); 00089 void power_on_reset(void); 00090 00091 rf_settings_t rfSettings; 00092 radio_state_t _mode; 00093 pck_ctrl_t _pck_control; 00094 modem_t _modem; 00095 00096 uint16_t _channel; 00097 uint16_t _datarate; 00098 uint8_t _address; 00099 uint8_t _lqi; 00100 uint16_t _rssi; 00101 uint8_t _chip_version; 00102 uint32_t _base_freq; 00103 }; 00104 00105 #endif // CC1101_RADIO_H 00106
Generated on Mon Jul 18 2022 20:09:01 by
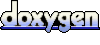