
A Hello World program showing how to get temperature readings from the MLX90614 infrared temperature sensor.
main.cpp
00001 /* 00002 * Example program showing how to get the temperature readings from 00003 * an MLX90614 infrared temperature sensor using an mbed. 00004 * http://www.haoyuelectronics.com/Attachment/GY-906/MLX90614.pdf 00005 * 00006 * Jonathan Jones 00007 */ 00008 00009 #include "mbed.h" 00010 #include "gy-906.h" 00011 00012 using namespace gy906; 00013 00014 I2C i2c(p9, p10); 00015 const uint8_t addr = default_addr; 00016 00017 // kelvin to fahrenheit 00018 float k2f(float raw_kelvin) 00019 { 00020 return (raw_kelvin - 273.15) * 1.8 + 32; 00021 } 00022 00023 // read and return one of the temperature regs 00024 float get_temp(uint8_t reg) { 00025 char cmd[3] = { 0 }; 00026 // read the temperature data (kelvin) 00027 cmd[0] = opcode::ram_access | reg; 00028 i2c.write(addr,cmd,1,true); i2c.read(addr,cmd,3); 00029 // convert to meaningful units, still in kelvin - just normalized 00030 return 0.02 * static_cast<float>((cmd[1]<<8)|cmd[0]); 00031 } 00032 00033 int main() { 00034 uint8_t reg_addrs[] = { ram::T_ambient, ram::T_obj1 }; 00035 float tt = 0.0; 00036 // clear terminal & hide cursor 00037 printf("\033[r\033[2J\033[?25l"); fflush(stdout); 00038 while (true) { 00039 for (size_t i = 0; i < sizeof(reg_addrs); ++i) { 00040 tt = get_temp(reg_addrs[i]); 00041 printf("\r\033[KT%u:\t%.2f°F\r\n", i, k2f(tt)); 00042 fflush(stdout); 00043 } 00044 wait(0.1); 00045 printf("\033[%uA", sizeof(reg_addrs)); fflush(stdout); 00046 } 00047 }
Generated on Fri Jul 22 2022 06:04:12 by
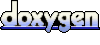